模拟python编译器
时间: 2023-04-08 11:03:23 浏览: 124
我可以回答这个问题。Python编译器是一种将Python代码转换为机器可执行代码的程序。Python编译器可以将Python代码转换为字节码,然后在运行时将字节码转换为机器代码。Python编译器有多种实现,包括CPython、Jython、IronPython等。您可以使用这些编译器来编写和运行Python程序。
相关问题
用python 模拟c++编译器的程序
模拟 C 编译器的程序需要使用 Python 语言创建一个解析器,将 C 代码转化为机器码。这个过程大致可以分为三个步骤:
1. 词法分析:将原始的 C 代码分解为关键字、标识符、运算符等基本单元,这个过程叫做词法分析。
2. 语法分析:将分解后的代码按照语法规则组织成语法树,这个过程叫做语法分析。
3. 代码生成:将语法树转换为机器码,并输出可执行程序。
下面是一个简单的示例,演示如何使用 Python 实现一个简单的 C 编译器:
```python
import re
# 定义 C 语言的关键字
keywords = ['auto', 'break', 'case', 'char', 'const', 'continue', 'default', 'do', 'double', 'else', 'enum', 'extern', 'float', 'for', 'goto', 'if', 'int', 'long', 'register', 'return', 'short', 'signed', 'sizeof', 'static', 'struct', 'switch', 'typedef', 'union', 'unsigned', 'void', 'volatile', 'while']
# 定义运算符和分隔符
operators = ['+', '-', '*', '/', '=', '>', '<', '>=', '<=', '==', '!=', '&&', '||', '!', '&', '|', '^', '~', '<<', '>>', '++', '--']
delimiters = ['(', ')', '{', '}', ';', ',']
# 定义一个函数,将源代码分解为基本单元
def tokenize(code):
tokens = []
source_code = code.strip()
while len(source_code) > 0:
match = None
# 匹配关键字、标识符、数字和字符串
for keyword in keywords:
pattern = '^' + keyword + r'(?![a-zA-Z0-9_])'
regex = re.compile(pattern)
match = regex.match(source_code)
if match:
tokens.append(('keyword', keyword))
source_code = source_code[match.end():].strip()
break
if not match:
pattern = r'^([a-zA-Z_]\w*)'
regex = re.compile(pattern)
match = regex.match(source_code)
if match:
tokens.append(('identifier', match.group()))
source_code = source_code[match.end():].strip()
continue
pattern = r'^(\d+)'
regex = re.compile(pattern)
match = regex.match(source_code)
if match:
tokens.append(('number', match.group()))
source_code = source_code[match.end():].strip()
continue
pattern = r'^"([^"]*)"'
regex = re.compile(pattern)
match = regex.match(source_code)
if match:
tokens.append(('string', match.group(1)))
source_code = source_code[match.end():].strip()
continue
# 匹配运算符和分隔符
for operator in operators + delimiters:
pattern = '^' + re.escape(operator)
regex = re.compile(pattern)
match = regex.match(source_code)
if match:
tokens.append(('operator', operator))
source_code = source_code[match.end():].strip()
break
if not match:
raise SyntaxError("Invalid syntax: " + source_code)
return tokens
# 将基本单元按照语法规则组织成语法树
def parse(tokens):
pass
# 将语法树转换为机器码,并输出可执行程序
def generate_code(tree):
pass
# 主程序,从文件中读取 C 代码,并编译为可执行程序
if __name__ == '__main__':
with open('test.c', 'r') as f:
code = f.read()
tokens = tokenize(code)
tree = parse(tokens)
generate_code(tree)
```
这个示例只实现了第一个步骤,将 C 代码分解为基本单元。你可以根据需要,自行实现后续的语法分析和代码生成过程。
阅读全文
相关推荐
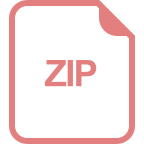
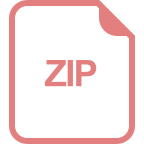
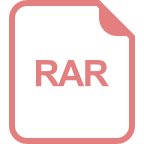
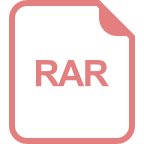
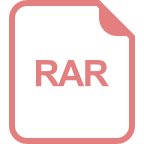
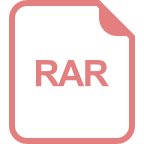
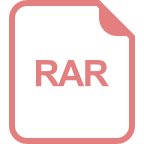
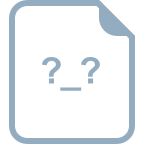
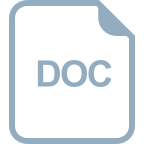
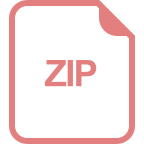
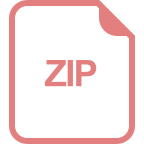
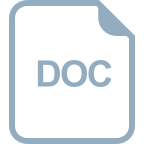
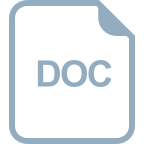
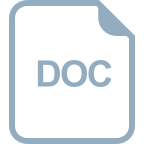
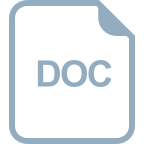