写段c#代码释放dll文件到指定目录
时间: 2024-05-06 07:17:42 浏览: 29
以下是一个示例代码段,可以在C#中使用来释放DLL文件到指定目录:
```csharp
using System.IO;
using System.Reflection;
// 获取当前程序集的路径
string assemblyPath = Path.GetDirectoryName(Assembly.GetExecutingAssembly().Location);
// DLL文件路径
string dllFilePath = Path.Combine(assemblyPath, "example.dll");
// 目标目录路径
string targetDirectory = @"C:\example";
// 将DLL文件复制到目标目录
File.Copy(dllFilePath, Path.Combine(targetDirectory, "example.dll"), true);
```
上述代码中,我们首先使用 `Assembly.GetExecutingAssembly().Location` 获取当前程序集的路径。然后使用 `Path.Combine` 将DLL文件名组合到路径中,以获取DLL文件的完整路径。
接下来,我们定义了一个目标目录路径,并调用 `File.Copy` 方法将DLL文件复制到该目录。需要注意的是,第三个参数 `true` 表示如果目标目录中已经存在同名文件,则覆盖原文件。
你可以将上述代码段放入你的C#项目中,并将其中的DLL文件路径和目标目录路径替换为实际的路径,以便实现将DLL文件释放到指定目录的功能。
相关问题
请实现代码,指定某个usb 设备只读,并允许C# 程式调用
以下是一份 C# 的示例代码,通过调用 libusb 库实现控制指定 USB 设备只读:
```csharp
using System;
using System.Runtime.InteropServices;
namespace USBDeviceReadOnlyControl {
class Program {
// 定义 libusb 库的函数
[DllImport("libusb-1.0.dll", CallingConvention = CallingConvention.Cdecl)]
public static extern int libusb_init(IntPtr ctx);
[DllImport("libusb-1.0.dll", CallingConvention = CallingConvention.Cdecl)]
public static extern int libusb_exit(IntPtr ctx);
[DllImport("libusb-1.0.dll", CallingConvention = CallingConvention.Cdecl)]
public static extern int libusb_get_device_list(IntPtr ctx, ref IntPtr list);
[DllImport("libusb-1.0.dll", CallingConvention = CallingConvention.Cdecl)]
public static extern void libusb_free_device_list(IntPtr list, int unref_devices);
[DllImport("libusb-1.0.dll", CallingConvention = CallingConvention.Cdecl)]
public static extern int libusb_get_device_descriptor(IntPtr dev, ref libusb_device_descriptor desc);
[DllImport("libusb-1.0.dll", CallingConvention = CallingConvention.Cdecl)]
public static extern int libusb_open(IntPtr dev, ref IntPtr handle);
[DllImport("libusb-1.0.dll", CallingConvention = CallingConvention.Cdecl)]
public static extern void libusb_close(IntPtr handle);
[DllImport("libusb-1.0.dll", CallingConvention = CallingConvention.Cdecl)]
public static extern int libusb_control_transfer(IntPtr handle, byte request_type, byte request, ushort value, ushort index, byte[] data, ushort length, uint timeout);
// 定义 libusb 库的结构体
[StructLayout(LayoutKind.Sequential)]
public struct libusb_device_descriptor {
public byte bLength;
public byte bDescriptorType;
public ushort bcdUSB;
public byte bDeviceClass;
public byte bDeviceSubClass;
public byte bDeviceProtocol;
public byte bMaxPacketSize0;
public ushort idVendor;
public ushort idProduct;
public ushort bcdDevice;
public byte iManufacturer;
public byte iProduct;
public byte iSerialNumber;
public byte bNumConfigurations;
}
// 控制 USB 设备只读的函数
public static int MakeUSBDeviceReadOnly(IntPtr dev_handle) {
int r = 0;
byte[] buffer = new byte[8];
// 发送命令给 USB 设备
buffer[0] = 0x0F; // 假设 0x0F 是只读命令
r = libusb_control_transfer(dev_handle, 0x40, 0x00, 0x00, 0x00, buffer, (ushort)buffer.Length, 1000);
if (r < 0) {
// 控制失败,返回错误码
return r;
}
// 控制成功,返回 0
return 0;
}
// 获取指定 Vendor ID 和 Product ID 的 USB 设备
public static IntPtr GetUSBDeviceByVIDPID(IntPtr list, ushort vendor_id, ushort product_id) {
IntPtr dev = IntPtr.Zero;
IntPtr dev_handle = IntPtr.Zero;
int r = 0;
// 遍历 USB 设备列表,查找指定 Vendor ID 和 Product ID 的设备
for (int i = 0; ; i++) {
IntPtr p = Marshal.ReadIntPtr(list, i * IntPtr.Size);
if (p == IntPtr.Zero) {
break;
}
libusb_device_descriptor desc = new libusb_device_descriptor();
r = libusb_get_device_descriptor(p, ref desc);
if (r < 0) {
continue;
}
if (desc.idVendor == vendor_id && desc.idProduct == product_id) {
// 打开设备
r = libusb_open(p, ref dev_handle);
if (r < 0) {
continue;
}
// 控制设备只读
r = MakeUSBDeviceReadOnly(dev_handle);
if (r < 0) {
continue;
}
// 找到了指定设备,返回设备句柄
dev = dev_handle;
break;
}
}
return dev;
}
// 示例代码
static void Main(string[] args) {
int r = 0;
IntPtr ctx = IntPtr.Zero;
IntPtr list = IntPtr.Zero;
IntPtr dev_handle = IntPtr.Zero;
// 初始化 libusb 库
r = libusb_init(ref ctx);
if (r < 0) {
// 初始化失败,返回错误码
Console.WriteLine("Failed to initialize libusb: {0}", r);
return;
}
// 获取 USB 设备列表
r = libusb_get_device_list(ctx, ref list);
if (r < 0) {
// 获取失败,返回错误码
Console.WriteLine("Failed to get USB device list: {0}", r);
libusb_exit(ctx);
return;
}
// 获取指定 Vendor ID 和 Product ID 的 USB 设备
ushort vendor_id = 0x1234; // 假设 Vendor ID 是 0x1234
ushort product_id = 0x5678; // 假设 Product ID 是 0x5678
dev_handle = GetUSBDeviceByVIDPID(list, vendor_id, product_id);
if (dev_handle == IntPtr.Zero) {
// 没有找到指定设备,返回错误码
Console.WriteLine("Failed to find USB device: {0:X4}:{1:X4}", vendor_id, product_id);
libusb_free_device_list(list, 1);
libusb_exit(ctx);
return;
}
// 关闭 USB 设备
libusb_close(dev_handle);
// 释放 USB 设备列表和 libusb 资源
libusb_free_device_list(list, 1);
libusb_exit(ctx);
// 控制成功,输出提示信息
Console.WriteLine("USB device {0:X4}:{1:X4} is now read-only.", vendor_id, product_id);
}
}
}
```
以上代码中,`GetUSBDeviceByVIDPID` 函数可以根据 Vendor ID 和 Product ID 获取指定的 USB 设备,并控制其只读。在实际使用时,需要将 Vendor ID 和 Product ID 修改为实际的值。另外,需要将 libusb 库的 DLL 文件拷贝到程序运行目录下。
用c#读取其他电脑的文件
要读取其他电脑的文件,需要使用网络共享功能。以下是使用C#访问其他计算机上共享文件夹的步骤:
1. 确保目标计算机上的文件夹已设置为“共享”。
2. 在C#项目中添加对System.IO和System.Net命名空间的引用。
3. 使用NetworkCredential对象创建凭据对象,以便在访问共享文件夹时进行身份验证。
4. 使用UNC路径(例如 \\computername\sharedfolder)访问共享文件夹。
5. 使用File类或Directory类的方法访问共享文件夹中的文件或文件夹。
以下是一个示例代码:
```
using System;
using System.IO;
using System.Net;
class Program
{
static void Main(string[] args)
{
string path = @"\\computername\sharedfolder\myfile.txt";
NetworkCredential credentials = new NetworkCredential("username", "password");
using (new NetworkConnection(path, credentials))
{
// 访问共享文件夹中的文件
string content = File.ReadAllText(path);
Console.WriteLine(content);
}
}
}
// NetworkConnection类,用于创建网络连接
public class NetworkConnection : IDisposable
{
private readonly string _networkName;
public NetworkConnection(string networkName, NetworkCredential credentials)
{
_networkName = networkName;
var netResource = new NetResource
{
Scope = ResourceScope.GlobalNetwork,
ResourceType = ResourceType.Disk,
DisplayType = ResourceDisplayType.Share,
RemoteName = networkName
};
var result = WNetAddConnection2(
netResource,
credentials.Password,
$"{credentials.Domain}\\{credentials.UserName}",
0);
if (result != 0)
{
throw new IOException($"Failed to connect to {_networkName}. Error: {result}");
}
}
~NetworkConnection()
{
Dispose(false);
}
public void Dispose()
{
Dispose(true);
GC.SuppressFinalize(this);
}
protected virtual void Dispose(bool disposing)
{
WNetCancelConnection2(_networkName, 0, true);
}
[System.Runtime.InteropServices.DllImport("mpr.dll")]
private static extern int WNetAddConnection2(NetResource netResource, string password, string username, int flags);
[System.Runtime.InteropServices.DllImport("mpr.dll")]
private static extern int WNetCancelConnection2(string name, int flags, bool force);
}
// NetResource类,用于设置网络资源的属性
public class NetResource
{
public ResourceScope Scope { get; set; }
public ResourceType ResourceType { get; set; }
public ResourceDisplayType DisplayType { get; set; }
public int Usage { get; set; }
public string LocalName { get; set; }
public string RemoteName { get; set; }
public string Comment { get; set; }
public string Provider { get; set; }
}
// 枚举类型,用于指定网络资源的范围、类型和显示方式
public enum ResourceScope : int
{
Connected = 1,
GlobalNetwork,
Remembered,
Recent,
Context
}
public enum ResourceType : int
{
Any = 0,
Disk = 1,
Print = 2,
Reserved = 8,
}
public enum ResourceDisplayType : int
{
Generic = 0x0,
Domain = 0x01,
Server = 0x02,
Share = 0x03,
File = 0x04,
Group = 0x05,
Network = 0x06,
Root = 0x07,
Shareadmin = 0x08,
Directory = 0x09,
Tree = 0x0a,
Ndscontainer = 0x0b
}
```
在上面的代码中,使用了一个名为“NetworkConnection”的自定义类,用于创建网络连接。该类使用WNetAddConnection2函数创建网络连接,并在不再需要时使用WNetCancelConnection2函数取消网络连接。使用using语句块创建NetworkConnection对象,以确保在使用完毕后自动释放资源。
请注意,为了访问共享文件夹,必须提供正确的凭据对象。在上面的示例代码中,使用NetworkCredential对象传递用户名和密码。如果目标计算机不需要凭据,则可以省略NetworkCredential对象。
相关推荐
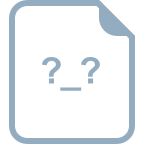
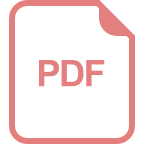
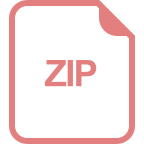









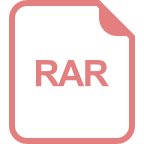
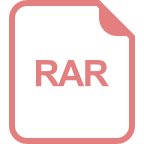
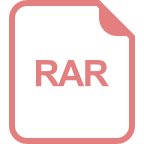
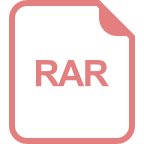