import org.apache.commons.pool2.BasePooledObjectFactory;import org.apache.commons.pool2.PooledObject;import org.apache.commons.pool2.impl.GenericObjectPool;public class MyObjectFactory extends BasePooledObjectFactory<MyObject> { @Override public MyObject create() throws Exception { // 创建对象 return new MyObject(); } @Override public PooledObject<MyObject> wrap(MyObject obj) { // 包装对象 return new DefaultPooledObject<>(obj); } @Override public void validateObject(PooledObject<MyObject> pooledObject) { // 验证对象是否可用 MyObject obj = pooledObject.getObject(); if (!obj.isUsable()) { throw new IllegalStateException("Object is not usable"); } }}class MyObject { private boolean usable = true; public boolean isUsable() { return usable; } public void setUsable(boolean usable) { this.usable = usable; }}public class Main { public static void main(String[] args) { // 创建对象池 GenericObjectPool<MyObject> pool = new GenericObjectPool<>(new MyObjectFactory()); // 从对象池中获取对象 MyObject obj = null; try { obj = pool.borrowObject(); System.out.println("Object borrowed from pool"); } catch (Exception e) { e.printStackTrace(); } finally { // 归还对象到对象池 if (obj != null) { pool.returnObject(obj); System.out.println("Object returned to pool"); } } }},该段代码还是会报错:Object has already been returned to this pool or is invali
时间: 2024-02-14 12:11:35 浏览: 161
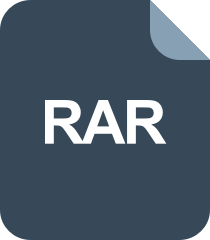
Apache common pool2 对象池
这个错误通常是因为对象在归还给池之前已经被关闭了,或者在归还时被其他线程抢占了。你可以加上同步锁来避免这个问题。修改代码如下:
```
public class Main {
public static void main(String[] args) {
// 创建对象池
GenericObjectPool<MyObject> pool = new GenericObjectPool<>(new MyObjectFactory());
// 从对象池中获取对象
MyObject obj = null;
try {
obj = pool.borrowObject();
System.out.println("Object borrowed from pool");
} catch (Exception e) {
e.printStackTrace();
} finally {
// 归还对象到对象池
if (obj != null) {
synchronized (pool) {
pool.returnObject(obj);
}
System.out.println("Object returned to pool");
}
}
}
}
```
在归还对象之前,我们加上了同步锁,确保归还对象操作的原子性。这样就可以避免出现对象被重复归还或者被其他线程抢占的情况。
阅读全文
相关推荐
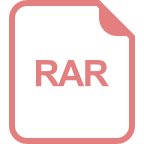
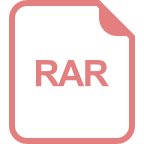
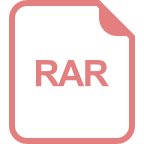
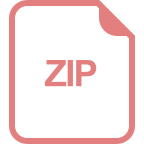
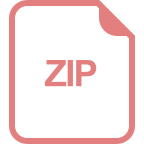
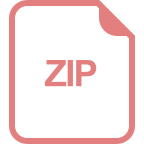
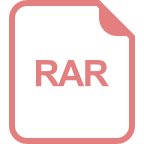
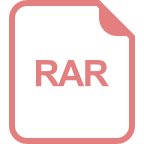
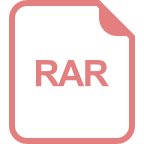
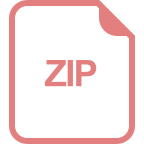
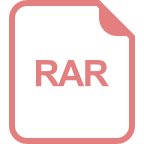
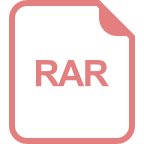
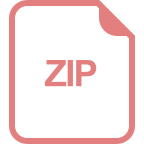
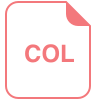
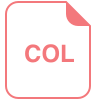
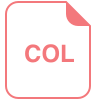
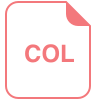
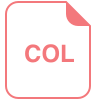