QCamera *camera = new QCamera(this);使用QT5.15.2读取摄像头数据,并且消除QAbstructVideoSurface镜像效果。
时间: 2023-08-31 15:31:04 浏览: 134
### 回答1:
要读取摄像头数据并消除QAbstractVideoSurface的镜像效果,您需要创建一个自定义的QAbstractVideoSurface子类,并在QCamera中设置它作为视频数据的目标。
以下是一个简单的示例:
1. 首先,创建一个自定义的QAbstractVideoSurface子类,例如MirrorFreeSurface。在这个类中,我们将实现videoFrameProbed()函数来处理摄像头帧数据。在这个函数中,我们可以对数据进行操作来消除镜像效果。
```c++
#include <QAbstractVideoSurface>
class MirrorFreeSurface : public QAbstractVideoSurface
{
Q_OBJECT
public:
MirrorFreeSurface(QObject *parent = nullptr)
: QAbstractVideoSurface(parent)
{
}
QList<QVideoFrame::PixelFormat> supportedPixelFormats(QAbstractVideoBuffer::HandleType handleType) const override
{
Q_UNUSED(handleType);
// 可以使用的视频帧格式
return QList<QVideoFrame::PixelFormat>()
<< QVideoFrame::Format_RGB24
<< QVideoFrame::Format_ARGB32;
}
bool present(const QVideoFrame &frame) override
{
// 处理视频帧数据以消除镜像效果
QVideoFrame mirroredFrame = frame;
mirroredFrame.setMirrored(true);
// 发送已处理的帧数据
emit frameAvailable(mirroredFrame);
return true;
}
};
```
2. 在需要使用摄像头数据的地方创建QCamera对象,并将MirrorFreeSurface对象设置为其目标视频表面。
```c++
// 创建 MirrorFreeSurface 实例
auto *surface = new MirrorFreeSurface(this);
// 创建 QCamera 实例
auto *camera = new QCamera(this);
// 将 MirrorFreeSurface 实例设置为 QCamera 实例的目标视频表面
camera->setViewfinder(surface);
// 启动摄像头
camera->start();
```
现在,您可以在MirrorFreeSurface类的frameAvailable()函数中处理视频帧数据,以消除其镜像效果。
### 回答2:
QCamera *camera = new QCamera(this);使用QT5.15.2读取摄像头数据,并且消除QAbstractVideoSurface镜像效果
在使用Qt版本5.15.2读取摄像头数据并消除QAbstractVideoSurface镜像效果,我们可以使用Qt的多媒体框架进行操作。首先我们可以创建一个QAbstractVideoSurface的子类,例如MyVideoSurface,继承自QAbstractVideoSurface类,并在其实现中覆写present函数。在present函数中,我们使用QPainter对视频帧进行翻转操作,以消除镜像效果。
```cpp
#include <QAbstractVideoSurface>
#include <QCamera>
#include <QCameraViewfinder>
class MyVideoSurface : public QAbstractVideoSurface
{
public:
QList<QVideoFrame::PixelFormat> supportedPixelFormats(QAbstractVideoBuffer::HandleType type) const override
{
Q_UNUSED(type);
return QList<QVideoFrame::PixelFormat>() << QVideoFrame::Format_NV12;
}
bool present(const QVideoFrame &frame) override
{
QVideoFrame frameCopy(frame);
frameCopy.map(QAbstractVideoBuffer::ReadOnly);
QImage image(
frameCopy.bits(),
frameCopy.width(),
frameCopy.height(),
QVideoFrame::imageFormatFromPixelFormat(frame.pixelFormat())
);
image = image.mirrored(false, true); // 翻转图像,消除镜像效果
// 在这里可以对翻转后的图像进行其他操作,如保存到本地,显示等
frameCopy.unmap();
return true;
}
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QCamera camera;
MyVideoSurface *videoSurface = new MyVideoSurface;
camera.setViewfinder(videoSurface);
// 其他相关操作,如启动摄像头、对窗口进行显示等
return app.exec();
}
```
以上是一个简单的示例代码,使用QCamera创建摄像头对象,并通过setViewfinder方法设置使用我们自定义的videoSurface来渲染视频。在我们的MyVideoSurface中,我们首先定义了支持的像素格式,然后在present函数中对视频帧进行镜像翻转操作,最后返回true表示渲染成功。你可以根据自己的需求对翻转后的图像进行其他操作,如保存到本地或者显示在窗口上。
### 回答3:
在QT5.15.2中使用QCamera类来读取摄像头数据,并且消除QAbstractVideoSurface的镜像效果,你可以按照以下步骤操作:
1. 首先,创建一个QCamera对象并传递一个父对象作为参数,例如:
```cpp
QCamera *camera = new QCamera(this);
```
2. 接下来,创建一个QAbstractVideoSurface的子类(例如自定义的VideoSurface类)来处理摄像头数据的显示。在这个自定义类中,你可以重写QAbstractVideoSurface的paint()函数来实现消除镜像效果的逻辑。例如:
```cpp
class VideoSurface : public QAbstractVideoSurface
{
Q_OBJECT
public:
VideoSurface(QObject *parent = nullptr) : QAbstractVideoSurface(parent) {}
// 重写paint()函数来处理摄像头数据的显示逻辑
virtual bool present(const QVideoFrame &frame) override
{
// 在这里进行消除镜像效果的处理
// 然后将处理后的数据显示出来
return true;
}
};
```
3. 然后,将自定义的VideoSurface对象设置为QCamera对象的摄像头采集源(VideoSurface对象将会负责处理摄像头数据的显示)。例如:
```cpp
VideoSurface *videoSurface = new VideoSurface(this);
camera->setViewfinder(videoSurface);
```
4. 最后,通过开始摄像头捕获来获取和显示摄像头数据。例如,调用camera的start()函数来开始捕获摄像头数据:
```cpp
camera->start();
```
在自定义的VideoSurface类中,你可以根据具体的需求实现消除镜像效果的方法。你可以通过对图像进行水平翻转,或者在渲染时对图像进行变换等方式来消除镜像效果。具体的处理逻辑需要根据你的需求来实现。
相关推荐
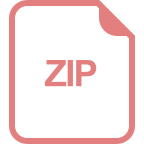
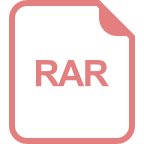













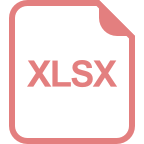
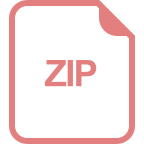