c++ 读取,储存和输出用户名的代码,关联用户名和余额,输入用户名时可显示该用户的余额,实现可录入多人资料,使用ofs ifs,并且尽量精简代码
时间: 2024-01-28 10:03:50 浏览: 61
好的,下面是使用 `ofstream` 和 `ifstream` 实现储存和读取用户名及其余额信息的示例代码:
```cpp
#include <iostream>
#include <fstream>
#include <map>
using namespace std;
// 定义用户信息结构体
struct UserInfo {
string name;
double balance;
};
// 定义用户信息映射表
map<string, UserInfo> user_map;
// 存储用户信息到文件
void save_user_info() {
ofstream outFile("user_info.txt");
if (outFile.is_open()) {
for (auto& user : user_map) {
outFile << user.first << " " << user.second.balance << endl;
}
outFile.close();
} else {
cout << "Unable to open file for writing." << endl;
}
}
// 从文件读取用户信息
void load_user_info() {
ifstream inFile("user_info.txt");
if (inFile.is_open()) {
string name;
double balance;
while (inFile >> name >> balance) {
UserInfo user_info;
user_info.name = name;
user_info.balance = balance;
user_map[name] = user_info;
}
inFile.close();
} else {
cout << "Unable to open file for reading." << endl;
}
}
// 显示用户余额
void show_balance(const string& name) {
auto it = user_map.find(name);
if (it != user_map.end()) {
cout << "Balance of " << name << ": " << it->second.balance << endl;
} else {
cout << "User " << name << " not found." << endl;
}
}
int main() {
// 先从文件中读取用户信息
load_user_info();
// 进行用户信息录入和显示
while (true) {
cout << "Enter user name (or 'quit' to exit): ";
string name;
cin >> name;
if (name == "quit") {
break;
}
auto it = user_map.find(name);
if (it != user_map.end()) {
// 用户已存在,显示余额
show_balance(name);
} else {
// 用户不存在,进行录入
cout << "Enter balance for " << name << ": ";
double balance;
cin >> balance;
UserInfo user_info;
user_info.name = name;
user_info.balance = balance;
user_map[name] = user_info;
}
}
// 录入完成后,保存用户信息到文件
save_user_info();
return 0;
}
```
在这个示例中,我们使用了 `map<string, UserInfo>` 类型来存储用户信息,其中 `string` 类型表示用户名,`UserInfo` 结构体表示用户信息。使用 `ofstream` 和 `ifstream` 来分别创建文件输出流和输入流对象,使用 `is_open()` 方法检查文件是否成功打开,然后使用 `<<` 操作符写入数据到文件中,使用 `>>` 操作符从文件中读取数据并存储到对应的映射表中。当需要显示用户余额时,我们通过查找映射表来找到对应的用户信息,然后输出余额即可。最后,我们使用 `save_user_info()` 函数将用户信息保存到文件中。
阅读全文
相关推荐



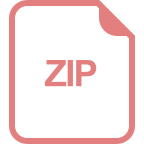













