如何统计一串字符串中每个字母出现的次数并按照字母表顺序输出
时间: 2024-12-02 09:16:50 浏览: 33
在Python中,你可以使用内置的collections模odule中的Counter类来统计字符出现的频率,然后遍历排序后的结果来显示每个字母及其出现次数。以下是一个示例程序:
```python
from collections import Counter
def count_letters(s):
# 使用空格分隔字符串,得到单个字符
letters = s.split(' ')
# 使用Counter计算每个字符出现的次数
letter_counts = Counter(letters)
# 按字母顺序对字典进行排序
sorted_counts = sorted(letter_counts.items(), key=lambda x: x[0])
for letter, count in sorted_counts:
print(f"'{letter}': {count}")
# 测试字符串
test_string = "hello world python programming"
count_letters(test_string)
```
运行此程序,它将输出每个字母及其在字符串中出现的次数,并按字母表顺序排列。
相关问题
C++如何统计一串字符串中每个字母出现的次数并按照字母表顺序输出
在C++中,你可以使用`std::map`数据结构来统计一串字符串中每个字母出现的次数。首先,遍历字符串,对于每一个字符,如果它不在`std::map`中,就添加一个新的键值对(键为字符,值为1),如果已经在地图中,则对应的值加1。然后,可以将`std::map`转换为`std::vector<std::pair<char, int>>`,以便按字母顺序排序。
下面是一个简单的示例:
```cpp
#include <iostream>
#include <string>
#include <map>
#include <algorithm>
int main() {
std::string str = "hello world";
std::map<char, int> charCount;
// 遍历字符串,统计字符频率
for (char c : str) {
if (std::isalpha(c)) { // 只统计字母
charCount[c]++;
}
}
// 将map转换为vector并排序
std::vector<std::pair<char, int>> sortedCount;
for (auto& pair : charCount) {
sortedCount.push_back(pair);
}
std::sort(sortedCount.begin(), sortedCount.end());
// 输出结果
for (const auto& [letter, count] : sortedCount) {
std::cout << letter << ": " << count << "\n";
}
return 0;
}
```
python编写程序maxchar(s),输入字符串s,统计字符串中出现次数最多的字母及其出现次数,用字典存放结果,并返回该值。如果有多个字母出现次数一样,则按字母表顺序输出字母及其出现次数
以下是实现代码:
```python
def maxchar(s):
count = {}
for char in s:
if char not in count:
count[char] = 1
else:
count[char] += 1
max_count = max(count.values())
max_chars = [char for char, char_count in count.items() if char_count == max_count]
max_chars.sort()
result = {max_chars[0]: max_count}
return result
```
解释如下:
首先定义一个空字典count用于记录每个字母的出现次数。
然后遍历输入字符串s中的每个字符,如果该字符不在count中,则将其加入count,并将其出现次数初始化为1;如果已经在count中,则将其出现次数加1。
统计完每个字符的出现次数后,使用max函数找到最大的出现次数max_count。
接着使用列表解析式找到出现次数为max_count的所有字符max_chars,使用sort方法对其进行排序,以便按字母表顺序输出。
最后将max_chars中第一个字符与其出现次数max_count存入字典result中,并返回该字典作为函数的输出。
阅读全文
相关推荐
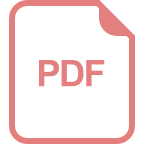
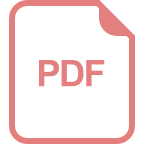
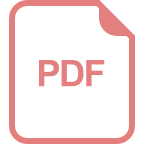













