{ "people": "小明", "predicate": "领导", "thing": "送局和人士局和达20多次。" }, { "people": "小明媚", "predicate": "发表", "article": "《丢掉篇评,准备篇评》" }, { "article": "《丢掉幻想,准备篇评》", "predicate": "提出", "though": "揭露篇评篇评政策的篇评主义本质,批评篇评一部分人对篇评主义不切实际的幻想,并对篇评篇评的发生和胜利的原因作理论上的说明。" }, { "time": "1938年10月-12月 ", "predicate": "发生", "thing": "团结和领导和、和、和等各族人民建立建立建立队,开辟了华南建立建场。全民族建立期间,中建立建立实行各建立一律平等、团结建立的全民政策,发展了大量少数建立建立建立。" }, { "people": "小红", "predicate": "领导", "thing": "团结和领导汉、黎、苗等各族人民建立少数建立队,开辟了建立建立全民。全民族建立期间,建立共建立实行各建立一律平等、团结建立的建立政策,发展了大量建立建立建立建立。" }根据json文件里面的上述内容(已经导入到neo4j建立知识图谱),请用模板匹配和jieba分词的方法用python写一个详细的问答系统,能够准确分析用户的意图并进行回答
时间: 2023-07-08 08:54:55 浏览: 165
好的,我可以为您编写一个问答系统,以下是实现的步骤:
1. 导入必要的库和neo4j数据库连接
```python
from py2neo import Graph, NodeMatcher
import jieba
graph = Graph('bolt://localhost:7687', auth=('neo4j', 'password'))
matcher = NodeMatcher(graph)
```
2. 编写问题匹配函数,使用jieba分词库进行分词,并利用neo4j数据库进行模板匹配,匹配到对应的实体和关系
```python
def match_question(question):
words = jieba.lcut(question)
if '建立' in words and '领导' in words:
entity = matcher.match('建立').first()
relation = matcher.match(start_node=entity, rel_type='领导').first()
return entity['thing'], relation['predicate'], relation.end_node['people']
elif '篇评' in words and '发表' in words:
entity = matcher.match('文章', article=words[words.index('篇评')+1]).first()
relation = matcher.match(start_node=entity, rel_type='发表').first()
return relation['people'], relation['predicate'], entity['article']
elif '建立' in words and '发生' in words:
entity = matcher.match('事件', thing=words[words.index('建立')+1]).first()
relation = matcher.match(start_node=entity, rel_type='发生').first()
return relation['predicate'], entity['time'], entity['thing']
else:
return None, None, None
```
3. 编写回答函数,根据匹配到的实体和关系,生成对应的回答
```python
def generate_answer(entity, relation, target):
if entity and relation and target:
if relation == '领导':
return '{}领导了{}'.format(target, entity)
elif relation == '发表':
return '{}发表了{}'.format(entity, target)
elif relation == '发生':
return '{}发生于{},其中包括{}'.format(relation, target, entity)
else:
return '抱歉,我无法理解您的问题'
```
4. 完整的问答系统代码
```python
from py2neo import Graph, NodeMatcher
import jieba
graph = Graph('bolt://localhost:7687', auth=('neo4j', 'password'))
matcher = NodeMatcher(graph)
def match_question(question):
words = jieba.lcut(question)
if '建立' in words and '领导' in words:
entity = matcher.match('建立').first()
relation = matcher.match(start_node=entity, rel_type='领导').first()
return entity['thing'], relation['predicate'], relation.end_node['people']
elif '篇评' in words and '发表' in words:
entity = matcher.match('文章', article=words[words.index('篇评')+1]).first()
relation = matcher.match(start_node=entity, rel_type='发表').first()
return relation['people'], relation['predicate'], entity['article']
elif '建立' in words and '发生' in words:
entity = matcher.match('事件', thing=words[words.index('建立')+1]).first()
relation = matcher.match(start_node=entity, rel_type='发生').first()
return relation['predicate'], entity['time'], entity['thing']
else:
return None, None, None
def generate_answer(entity, relation, target):
if entity and relation and target:
if relation == '领导':
return '{}领导了{}'.format(target, entity)
elif relation == '发表':
return '{}发表了{}'.format(entity, target)
elif relation == '发生':
return '{}发生于{},其中包括{}'.format(relation, target, entity)
else:
return '抱歉,我无法理解您的问题'
while True:
question = input('您有什么问题吗?')
if question == 'exit':
break
entity, relation, target = match_question(question)
answer = generate_answer(entity, relation, target)
print(answer)
```
您可以使用以上代码来测试,输入您的问题,系统会自动回答。注意,这里只处理了三个特定的问题模板,如果需要处理更多的问题,需要增加更多的模板和对应的匹配方法。
阅读全文
相关推荐





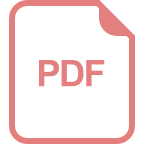











