请编写一个简单的多输入单输出的mlp模型进行回归预测
时间: 2023-08-31 15:20:24 浏览: 51
### 回答1:
好的,以下是一个简单的多输入单输出的MLP模型的代码:
```python
from keras.models import Sequential
from keras.layers import Dense
# 定义模型
model = Sequential()
model.add(Dense(10, input_dim=3, activation='relu'))
model.add(Dense(1))
# 编译模型
model.compile(loss='mean_squared_error', optimizer='adam')
# 训练模型
X = [[1, 2, 3], [4, 5, 6], [7, 8, 9], [10, 11, 12]] # 输入数据
y = [4, 10, 16, 22] # 目标值
model.fit(X, y, epochs=1000, verbose=0)
# 使用模型进行预测
x_new = [[13, 14, 15], [16, 17, 18]]
y_pred = model.predict(x_new)
print(y_pred)
```
在这个模型中,我们使用了一个输入维度为3的层,并且使用了激活函数relu,然后连接到一个输出层,输出一个标量值。我们使用了均方误差作为损失函数,使用Adam优化器进行优化。在训练过程中,我们使用了4个样本进行训练,训练了1000个epochs。最后,我们使用训练好的模型对两个新的样本进行预测,并打印出预测结果。
### 回答2:
多输入单输出的多层感知机(MLP)模型可以应用于回归预测任务。在编写代码之前,需要导入所需的库,例如`numpy`、`tensorflow`或`PyTorch`等。以下是使用`tensorflow`编写的一个简单的多输入单输出的MLP模型进行回归预测的示例代码:
```python
import numpy as np
import tensorflow as tf
# 创建模型
def create_mlp():
model = tf.keras.Sequential([
tf.keras.layers.Dense(units=64, activation='relu', input_shape=(2,)),
tf.keras.layers.Dense(units=32, activation='relu'),
tf.keras.layers.Dense(units=1)
])
return model
# 准备数据
x1 = np.random.rand(100) # 第一个输入数据,维度为100
x2 = np.random.rand(100) # 第二个输入数据,维度为100
y = 2*x1 + 3*x2 + np.random.normal(0, 0.1, size=100) # 输出数据,满足线性关系,添加噪声
# 构建模型
model = create_mlp()
# 编译模型
model.compile(optimizer='adam', loss='mse')
# 训练模型
model.fit([x1, x2], y, epochs=10, batch_size=32)
# 进行预测
test_x1 = np.random.rand(10)
test_x2 = np.random.rand(10)
predictions = model.predict([test_x1, test_x2])
print(predictions)
```
以上代码中,我们首先创建了一个简单的MLP模型,该模型包含一个输入层,两个隐藏层和一个输出层。输入层有两个节点,对应两个输入数据。隐藏层的节点数分别为64和32,使用ReLU激活函数。输出层只有一个节点。在准备好输入和输出数据后,我们通过编译模型、训练模型和进行预测来完成回归任务。在训练过程中,我们指定了优化器为Adam,损失函数为均方误差(Mean Squared Error)。
### 回答3:
要编写一个简单的多输入单输出的多层感知机(MLP)模型进行回归预测,首先需要导入所需的库,如 TensorFlow 和 Keras。然后可以按照以下步骤进行编写:
1. 导入所需的库:
```python
import tensorflow as tf
from tensorflow import keras
from tensorflow.keras import layers
```
2. 构建模型:
```python
input1 = keras.Input(shape=(n_features1,))
input2 = keras.Input(shape=(n_features2,))
# 使用层的序列构建模型
x1 = layers.Dense(32, activation="relu")(input1)
x2 = layers.Dense(32, activation="relu")(input2)
# 结合多个输入
merged = layers.Concatenate()([x1, x2])
# 输出层
output = layers.Dense(1)(merged)
# 定义模型
model = keras.Model(inputs=[input1, input2], outputs=output)
```
在上面的代码中,我们首先定义了两个输入层,每个输入层的形状分别为 `n_features1` 和 `n_features2`,然后将每个输入层连接到一个隐藏层,使用 `relu` 作为激活函数。接下来,我们使用 `Concatenate()` 层将两个隐藏层的输出连接起来,最后连接到输出层,输出层为一个神经元。
3. 编译模型:
```python
model.compile(optimizer="adam", loss="mse")
```
在这里,我们使用 Adam 优化器和均方误差作为损失函数进行模型编译。
4. 训练模型:
```python
model.fit([input1_train, input2_train], output_train, epochs=10, batch_size=32)
```
在这里,`input1_train` 和 `input2_train` 是训练数据的输入特征,`output_train` 是训练数据的输出目标。我们可以指定训练的轮数和批量大小。
5. 进行预测:
```python
predictions = model.predict([input1_test, input2_test])
```
在这里,`input1_test` 和 `input2_test` 是测试数据的输入特征,使用训练好的模型进行预测。
这是一个简单的多输入单输出的 MLP 模型进行回归预测的代码。你可以根据自己的数据和需求进行调整和扩展。
相关推荐
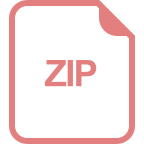
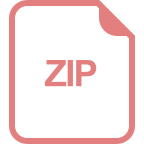
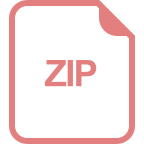














