写一个登录拦截器的demo
时间: 2023-03-02 22:21:18 浏览: 66
好的,这是一个用 Java 实现的登录拦截器 demo:
```java
public class LoginInterceptor extends HandlerInterceptorAdapter {
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception {
HttpSession session = request.getSession();
Object user = session.getAttribute("user");
if (user == null) {
response.sendRedirect("/login");
return false;
}
return true;
}
}
```
这个拦截器需要继承 `HandlerInterceptorAdapter`,然后重写 `preHandle` 方法。在该方法中,我们从 `HttpSession` 中获取当前登录用户,如果用户没有登录,就重定向到登录页面。否则,继续请求处理。
注意:这只是一个简单的 demo,在实际的项目中,还需要进行其他处理,例如异常处理等。
相关问题
帮我写个拦截器demo
好的,以下是一个简单的拦截器的示例,使用 Java 实现:
```
import java.io.IOException;
import okhttp3.Interceptor;
import okhttp3.Request;
import okhttp3.Response;
public class LoggingInterceptor implements Interceptor {
@Override public Response intercept(Interceptor.Chain chain) throws IOException {
Request request = chain.request();
long t1 = System.nanoTime();
System.out.println(String.format("Sending request %s on %s%n%s",
request.url(), chain.connection(), request.headers()));
Response response = chain.proceed(request);
long t2 = System.nanoTime();
System.out.println(String.format("Received response for %s in %.1fms%n%s",
response.request().url(), (t2 - t1) / 1e6d, response.headers()));
return response;
}
}
```
在这个例子中,我们实现了一个 `Interceptor` 类,并重写了其中的 `intercept` 方法。在该方法中,我们打印了发送请求前和收到响应后的信息,以方便查看请求和响应的相关信息。
请帮我用spring安全框架做一个登录拦截的demo
好的,下面是一个简单的基于 Spring Security 的登录拦截 Demo:
1. 首先,需要添加 Spring Security 的依赖,例如在 Maven 中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-web</artifactId>
<version>5.2.2.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-config</artifactId>
<version>5.2.2.RELEASE</version>
</dependency>
```
2. 在 Spring 配置文件中,添加 Spring Security 的配置:
```xml
<security:http auto-config="true">
<security:intercept-url pattern="/secure/**" access="isAuthenticated()" />
<security:form-login login-page="/login" default-target-url="/secure/home" authentication-failure-url="/login?error" />
<security:logout logout-success-url="/login?logout" />
</security:http>
<security:authentication-manager>
<security:authentication-provider>
<security:user-service>
<security:user name="admin" password="123456" authorities="ROLE_USER" />
</security:user-service>
</security:authentication-provider>
</security:authentication-manager>
```
上面的配置中,`<security:http>` 定义了 HTTP 请求的安全配置,`<security:intercept-url>` 定义了需要认证的 URL,`<security:form-login>` 定义了登录页面的 URL 和登录成功后的默认页面,`<security:logout>` 定义了退出登录后的跳转页面。
`<security:authentication-manager>` 定义了认证管理器,`<security:authentication-provider>` 定义了用户认证方式,这里使用了内存中的用户信息。
3. 添加登录页面和安全页面:
在 `login.jsp` 中,添加登录表单:
```html
<form method="post" action="/login">
<input type="text" name="username" placeholder="Username" />
<input type="password" name="password" placeholder="Password" />
<button type="submit">Login</button>
</form>
```
在 `home.jsp` 中,添加安全页面的内容。
4. 在 Web.xml 中添加 Spring Security 的过滤器:
```xml
<filter>
<filter-name>springSecurityFilterChain</filter-name>
<filter-class>org.springframework.web.filter.DelegatingFilterProxy</filter-class>
</filter>
<filter-mapping>
<filter-name>springSecurityFilterChain</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
```
这样就完成了一个简单的基于 Spring Security 的登录拦截 Demo。
相关推荐
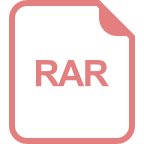












