unity 使用MQTTnet.dll写的服务器demo
时间: 2024-02-28 19:53:13 浏览: 120
以下是一个使用 MQTTnet 库在 Unity 中实现 MQTT 服务器的示例代码:
```csharp
using System;
using System.Collections.Generic;
using System.Threading.Tasks;
using MQTTnet;
using MQTTnet.Server;
using UnityEngine;
public class MqttServer : MonoBehaviour
{
private IMqttServer mqttServer;
public string brokerIpAddress = "0.0.0.0"; // MQTT 服务器 IP 地址
public int brokerPort = 1883; // MQTT 服务器端口号
public List<string> topics = new List<string>() { "testTopic" }; // 要订阅的主题列表
async void Start()
{
// 创建 MQTT 服务器实例
var optionBuilder = new MqttServerOptionsBuilder()
.WithDefaultEndpoint()
.WithDefaultEndpointPort(brokerPort)
.WithConnectionValidator(c =>
{
// 验证客户端连接
if (c.ClientId.Length < 10)
{
c.ReasonCode = MQTTnet.Protocol.MqttConnectReasonCode.ClientIdentifierNotValid;
return;
}
c.ReasonCode = MQTTnet.Protocol.MqttConnectReasonCode.Success;
})
.WithSubscriptionInterceptor(c =>
{
// 订阅主题
foreach (var topic in topics)
{
c.AcceptSubscription = c.TopicFilter.Topic == topic;
}
})
.WithApplicationMessageInterceptor(c =>
{
// 处理接收到的消息
var payload = c.ApplicationMessage.Payload;
var message = System.Text.Encoding.UTF8.GetString(payload);
Debug.Log($"Received message: {message}");
});
mqttServer = new MqttFactory().CreateMqttServer();
await mqttServer.StartAsync(optionBuilder.Build());
}
async void OnDestroy()
{
// 关闭 MQTT 服务器
await mqttServer.StopAsync();
}
}
```
这里使用了 MQTTnet 库,需要在 Unity 项目中导入该库的 DLL 文件。在 `Start` 方法中创建了一个 MQTT 服务器实例,并设置了连接验证器、订阅拦截器和应用消息拦截器。其中,订阅拦截器中订阅了指定的主题,应用消息拦截器中处理接收到的消息。在 `OnDestroy` 方法中关闭 MQTT 服务器。
需要注意的是,这个示例只是一个简单的 MQTT 服务器,没有考虑并发连接和性能优化问题,仅供参考。如果需要开发生产环境中使用的 MQTT 服务器,需要进行更多的测试和优化。
阅读全文
相关推荐

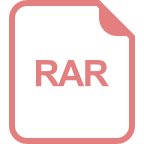
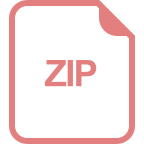
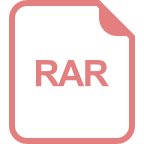
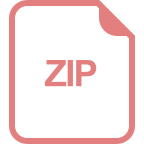
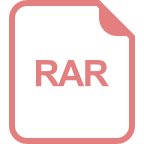
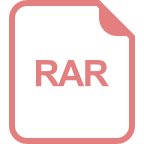
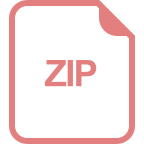
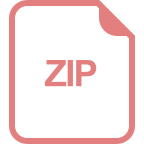
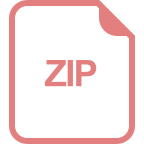