c语言输入一个班的成绩求最高分
时间: 2024-11-15 11:26:59 浏览: 12
在C语言中,你可以使用数组来存储班级成绩,并通过遍历数组来找到最高分。以下是一个简单的示例程序:
```c
#include <stdio.h>
int main() {
int num_students, max_score = 0; // 初始化最大分数为0
int class_scores[50]; // 假设班级有最多50人
printf("请输入班级学生人数:");
scanf("%d", &num_students);
if (num_students > 50) {
printf("人数超过限制,输入无效。\n");
return 1;
}
for (int i = 0; i < num_students; i++) {
printf("请输入第%d名学生的成绩:", i+1);
scanf("%d", &class_scores[i]);
// 比较当前成绩是否大于已知的最大值
if (class_scores[i] > max_score) {
max_score = class_scores[i];
}
}
printf("班级最高分为:%d\n", max_score);
return 0;
}
相关问题
c语言输入四个成绩求最高分最低分
你好,这是一个关于C语言的问题。输入四个成绩求最高分最低分的代码可以这样写:
#include <stdio.h>
int main() {
float score1, score2, score3, score4;
printf("请输入四个成绩:\n");
scanf("%f%f%f%f", &score1, &score2, &score3, &score4);
float max_score = score1;
if (score2 > max_score) {
max_score = score2;
}
if (score3 > max_score) {
max_score = score3;
}
if (score4 > max_score) {
max_score = score4;
}
float min_score = score1;
if (score2 < min_score) {
min_score = score2;
}
if (score3 < min_score) {
min_score = score3;
}
if (score4 < min_score) {
min_score = score4;
}
printf("最高分是:%.2f,最低分是:%.2f\n", max_score, min_score);
return 0;
}
希望能帮到您。如果您还有其他问题,可以继续问我。
C语言输入12名学生成绩求出最高分学号并将成绩从高到低排序输出
下面是一个 C 语言程序可以实现您的要求:
```c
#include <stdio.h>
#define N 12
int main() {
int scores[N];
int max_score = -1, max_score_index = -1;
int i, j;
// 输入学生成绩
for (i = 0; i < N; i++) {
printf("请输入第 %d 名学生的成绩:", i + 1);
scanf("%d", &scores[i]);
// 更新最高分学号
if (scores[i] > max_score) {
max_score = scores[i];
max_score_index = i;
}
}
// 输出最高分学号
printf("最高分学生的学号为 %d,成绩为 %d\n", max_score_index + 1, max_score);
// 对成绩排序
for (i = 0; i < N - 1; i++) {
for (j = i + 1; j < N; j++) {
if (scores[i] < scores[j]) {
int temp = scores[i];
scores[i] = scores[j];
scores[j] = temp;
}
}
}
// 输出成绩排名
printf("成绩从高到低的排名为:\n");
for (i = 0; i < N; i++) {
printf("%d ", scores[i]);
}
return 0;
}
```
程序中首先定义了一个长度为 12 的数组 `scores`,用于存储学生成绩。然后通过一个循环输入每个学生的成绩,并且在输入的同时更新最高分学号。接着通过两个嵌套循环对学生成绩进行排序,最后输出最高分学号和成绩排名。
阅读全文
相关推荐
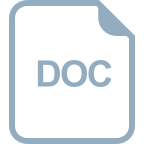
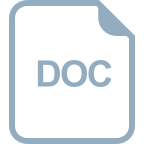
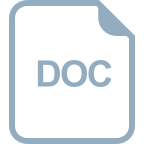













