请再次检查代码是否有错误,当我在控制台看到“Enter the number of characters:”消息并输入一个数字后,程序并没有向后继续运行
时间: 2024-02-09 15:10:40 浏览: 89
这个问题可能是由于代码中的某些错误导致的。首先,您需要确保控制台消息的格式正确,以便用户能够正确地输入数字。您可以尝试使用以下代码来获取用户输入:
```
try:
num_chars = int(input("Enter the number of characters: "))
except ValueError:
print("Please enter a valid integer.")
```
该代码将尝试将用户输入转换为整数。如果用户输入的不是整数,则会引发ValueError异常,并显示一条错误消息。如果用户输入了一个有效的整数,则程序将继续执行。
如果您的代码已经包含类似的逻辑,请检查是否有任何错误,例如语法错误或逻辑错误。您还可以尝试在代码中添加调试语句,以便您能够更轻松地找到问题所在。
相关问题
import java.io.*;public class TextStatistics { private String inputFileName; private String outputFileName; private int numChars; private int numWords; public TextStatistics(String inputFile, String outputFile) { inputFileName = inputFile; outputFileName = outputFile; numChars = 0; numWords = 0; } public void count() { try { BufferedReader reader = new BufferedReader(new FileReader(inputFileName)); String line; while ((line = reader.readLine()) != null) { numChars += line.length(); String[] words = line.split(" "); numWords += words.length; } reader.close(); } catch (IOException e) { e.printStackTrace(); } } public void output(boolean toFile) { String output = "Number of characters: " + numChars + "\n"; output += "Number of words: " + numWords + "\n"; if (toFile) { try { BufferedWriter writer = new BufferedWriter(new FileWriter(outputFileName)); writer.write(output); writer.close(); } catch (IOException e) { e.printStackTrace(); } } else { System.out.println(output); } }}public class TextStatisticsTest { public static void main(String[] args) { System.out.println("Welcome to Text Statistics!"); System.out.println("Please enter the name of the input file: "); Scanner scanner = new Scanner(System.in); String inputFile = scanner.nextLine(); System.out.println("Please enter the name of the output file: "); String outputFile = scanner.nextLine(); System.out.println("Do you want to output to a file? (Y/N)"); boolean toFile = scanner.nextLine().equalsIgnoreCase("Y"); TextStatistics stats = new TextStatistics(inputFile, outputFile); stats.count(); stats.output(toFile); }}
这段代码是用 Java 编写的一个文本统计程序,可以统计输入文件中的字符数和单词数,并将结果输出到控制台或输出文件中。程序包括两个类,TextStatistics 类和 TextStatisticsTest 类。TextStatistics 类用于实现文本统计功能,其中 count() 方法用于统计字符数和单词数,output() 方法用于输出结果到控制台或输出文件中。TextStatisticsTest 类用于测试 TextStatistics 类的功能,其中通过控制台输入输入文件名、输出文件名和是否输出到文件的选项,并调用 TextStatistics 类的方法进行统计和输出。
用c语言写一个程序,要求倒计时20秒,同时还可以输入文字并检测是否符合要求
你可以使用C语言编写一个简单的控制台程序,结合`time.h`库实现倒计时,并使用标准输入流`stdin`接收用户输入,检查字符串是否符合特定要求。下面是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// 检查输入的字符串是否全部由数字组成
int is_number(const char *str) {
while (*str != '\0') {
if (!isdigit(*str)) {
return 0;
}
str++;
}
return 1;
}
void countdown(int seconds) {
for (int i = seconds; i > 0; i--) {
printf("Seconds remaining: %d\n", i);
sleep(1); // 使用sleep暂停1秒,实际环境需要替换为更精确的延时方法
}
printf("\nCountdown finished.\n");
}
int main() {
int input_seconds;
printf("Enter the number of seconds for countdown (0 to exit): ");
scanf("%d", &input_seconds);
if (input_seconds <= 0) {
printf("Exiting program.\n");
return 0;
}
countdown(input_seconds);
char user_input[100];
printf("Enter a string to check (numbers only accepted): ");
fgets(user_input, sizeof(user_input), stdin);
user_input[strcspn(user_input, "\n")] = '\0'; // Remove newline at the end
if (is_number(user_input)) {
printf("Input is valid (only numbers).\n");
} else {
printf("Input is not valid (contains non-number characters).\n");
}
return 0;
}
```
在这个程序中,我们首先定义了一个函数`is_number`来检查输入的字符串是否全为数字。然后在`main`函数中,获取用户的倒计时时间,如果小于等于0则退出。接着倒数并显示剩余秒数,最后读取用户输入并验证其是否只包含数字。
阅读全文
相关推荐
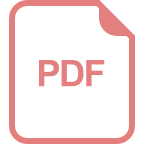
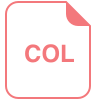
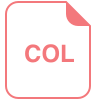
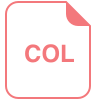
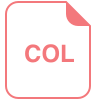
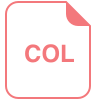
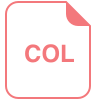
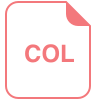
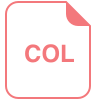


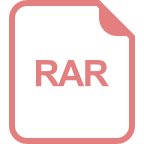
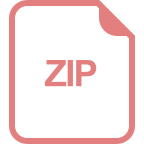
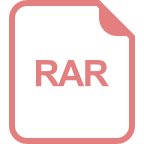