C++ while循环读入数据
时间: 2023-10-23 15:12:38 浏览: 133
好的,以下是 C++ 中使用 while 循环读入数据的示例代码:
```c++
#include <iostream>
using namespace std;
int main() {
int num;
while (cin >> num) {
cout << "输入的数字是:" << num << endl;
}
return 0;
}
```
在这个示例中,我们使用 `cin` 从标准输入流中读取数据,并将其存储在 `num` 变量中。当输入流结束时,`cin` 的状态会变为 `false`,此时 while 循环会结束。
相关问题
C++ while未知循环次数例子
以下是一个使用 while 循环的未知循环次数的 C++ 代码示例:
```c++
#include <iostream>
using namespace std;
int main() {
int num, sum = 0;
cout << "输入数字(输入0结束):" << endl;
while (true) {
cin >> num;
if (num == 0) {
break;
}
sum += num;
}
cout << "总和为:" << sum << endl;
return 0;
}
```
在这个例子中,我们要求用户输入一系列数字,直到输入 0 为止。我们使用一个 while 循环来实现这个要求,因为我们不知道用户会输入多少个数字。while 循环会一直执行,直到遇到 break 语句,这个语句会使循环停止。在每次循环中,我们会读取用户输入的数字,如果数字为 0,我们就跳出循环。如果数字不为 0,我们就将它加到 sum 变量中。最后,我们输出所有输入数字的总和。
C++循环重复读取txt文件指定数据
循环重复读取txt文件指定数据时,可以使用while循环和文件流中的seekg()函数实现。以下是一个示例代码:
```c++
#include <iostream>
#include <fstream>
using namespace std;
int main() {
ifstream inFile("data.txt"); // 打开txt文件
int n = 5; // 假设要读取第5个数据
int data;
while (true) {
inFile.seekg(0, ios::beg); // 将文件指针移动到文件开头
for (int i = 0; i < n-1; i++) { // 循环读取前n-1个数据
inFile >> data;
}
inFile >> data; // 读取第n个数据
if (!inFile.eof()) { // 如果读取成功则跳出循环
break;
}
cout << "无法读取第" << n << "个数据,文件中没有足够的数据!" << endl;
}
cout << "第" << n << "个数据为:" << data << endl;
inFile.close(); // 关闭文件流
return 0;
}
```
其中,seekg()函数用于将文件指针移动到指定位置。在循环读取数据时,每次循环都将文件指针移动到文件开头,然后重新读取数据。如果读取成功,则跳出循环;否则继续循环,直到读取成功或者到达文件末尾。
相关推荐
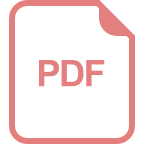
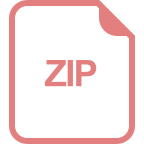
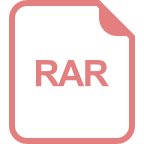












