能否帮我写一个输入简谱播放音乐的c++程序
时间: 2023-03-19 20:21:32 浏览: 299
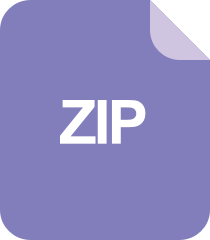
Qt/C++编写的带频谱的音乐播放器

当然可以!以下是一个简单的C程序,用于读取一个字符串表示的简谱,然后将其转换为MIDI音符,并通过MIDI输出设备播放。
请注意,此程序需要使用MIDI输出设备才能正常工作。您需要将MIDI输出设备连接到您的计算机,并根据需要调整程序中的设备名称。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#include <windows.h>
#include <mmsystem.h>
#define NOTE_ON 0x90
#define NOTE_OFF 0x80
#define VELOCITY 127
void playNote(int note, int duration);
void sleep(int duration);
int main()
{
char input[1024];
printf("请输入简谱:");
scanf("%s", input);
// 将简谱转换为MIDI音符,并播放
int len = strlen(input);
for (int i = 0; i < len; i++) {
char c = input[i];
int note = 0;
int duration = 500;
switch (c) {
case '1':
note = 60; // 中央C
break;
case '2':
note = 62; // D
break;
case '3':
note = 64; // E
break;
case '4':
note = 65; // F
break;
case '5':
note = 67; // G
break;
case '6':
note = 69; // A
break;
case '7':
note = 71; // B
break;
case ' ':
// 空格表示休止符
sleep(duration);
continue;
default:
continue; // 跳过非数字字符
}
playNote(note, duration);
}
return 0;
}
void playNote(int note, int duration)
{
// 打开MIDI输出设备
HMIDIOUT hMidiOut;
midiOutOpen(&hMidiOut, MIDI_MAPPER, 0, 0, 0);
// 发送Note On消息
midiOutShortMsg(hMidiOut, NOTE_ON | (0 << 8) | note << 16 | VELOCITY << 24);
// 等待一段时间
sleep(duration);
// 发送Note Off消息
midiOutShortMsg(hMidiOut, NOTE_OFF | (0 << 8) | note << 16 | VELOCITY << 24);
// 关闭MIDI输出设备
midiOutClose(hMidiOut);
}
void sleep(int duration)
{
// 等待一段时间,单位是毫秒
clock_t start = clock();
while (clock() - start < duration) {
Sleep(1);
}
}
```
这个程序会提示您输入简谱,然后将其转换为MIDI音符并播放。如果您输入的简谱不包含任何数字字符,则程序将不会播放任何音符。
请注意,此程序不处理节拍和节奏。每个音符的持续时间都是相同的,为500毫秒。如果您需要更复杂的节奏和持续时间,请根据需要修改程序。
阅读全文
相关推荐
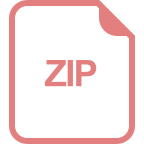
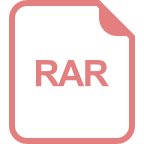
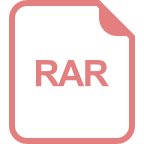



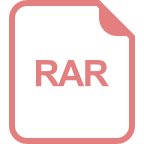
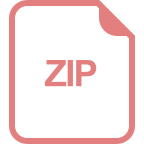
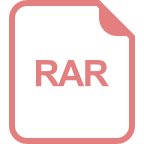
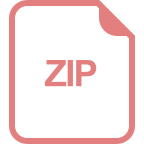
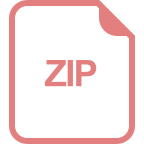
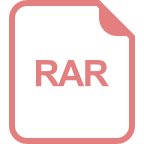
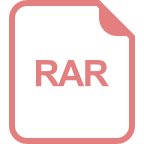
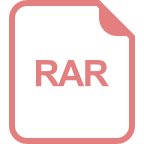
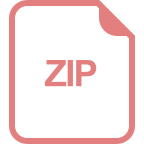
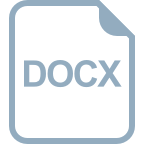
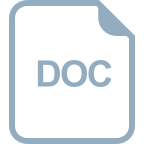