利用JavaGUI和JDBC编程实现学生成绩查询功能。(输入学生学号, 即可查询到该学 生的所有成绩)
时间: 2024-02-25 14:54:20 浏览: 86
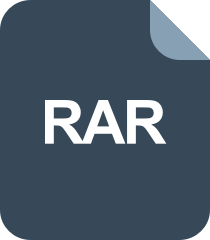
JAVA学生成绩查询系统

好的,您可以参考以下代码实现:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.sql.*;
public class GradeQuery extends JFrame implements ActionListener {
private JLabel label;
private JTextField textField;
private JTextArea textArea;
private JButton button;
private Connection conn;
private Statement stmt;
public GradeQuery() {
// 创建GUI界面
label = new JLabel("请输入学生学号:");
textField = new JTextField(10);
textArea = new JTextArea(20, 30);
button = new JButton("查询");
JPanel panel = new JPanel(new FlowLayout());
panel.add(label);
panel.add(textField);
panel.add(button);
add(panel, BorderLayout.NORTH);
add(new JScrollPane(textArea), BorderLayout.CENTER);
// 设置窗口属性
setTitle("学生成绩查询");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(400, 400);
setLocationRelativeTo(null);
setResizable(false);
// 注册按钮事件监听器
button.addActionListener(this);
// 连接数据库
try {
Class.forName("com.mysql.cj.jdbc.Driver");
String url = "jdbc:mysql://localhost:3306/test?serverTimezone=UTC";
String user = "root";
String password = "123456";
conn = DriverManager.getConnection(url, user, password);
stmt = conn.createStatement();
} catch (Exception e) {
e.printStackTrace();
}
}
public void actionPerformed(ActionEvent e) {
// 获取输入的学号
String id = textField.getText().trim();
try {
// 查询学生的成绩
String sql = "SELECT * FROM grade WHERE id='" + id + "'";
ResultSet rs = stmt.executeQuery(sql);
// 显示查询结果
textArea.setText("");
if (rs.next()) {
String name = rs.getString("name");
int chinese = rs.getInt("chinese");
int math = rs.getInt("math");
int english = rs.getInt("english");
int total = chinese + math + english;
double average = total / 3.0;
textArea.append("姓名:" + name + "\n");
textArea.append("语文:" + chinese + "\n");
textArea.append("数学:" + math + "\n");
textArea.append("英语:" + english + "\n");
textArea.append("总分:" + total + "\n");
textArea.append("平均分:" + average + "\n");
} else {
textArea.append("未找到该学生的成绩!");
}
} catch (Exception ex) {
ex.printStackTrace();
}
}
public static void main(String[] args) {
new GradeQuery().setVisible(true);
}
}
```
这个程序通过GUI界面输入学生的学号,然后利用JDBC连接MySQL数据库查询学生的成绩,并在GUI界面上显示查询结果。需要注意的是,这个程序需要依赖MySQL的JDBC驱动,您需要先下载并安装MySQL,并下载相应的驱动jar包,然后在程序中添加驱动的classpath。
阅读全文
相关推荐












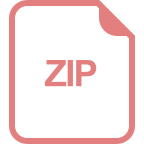
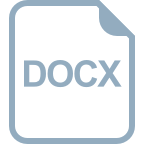
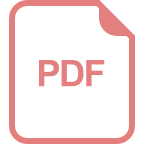