利用JavaGUI和JDBC编程实现学生成绩查询功能。(输入学生学号,即可查询到该学生的所有成绩)
时间: 2024-02-25 13:56:21 浏览: 28
好的,我可以为您解答这个问题。
首先,您需要创建一个Java GUI界面,用于输入学生学号。您可以使用Swing或JavaFX库来创建GUI。
接下来,您需要连接到数据库并查询学生成绩。您可以使用JDBC(Java Database Connectivity)来连接到数据库,并执行SQL查询语句。
下面是一个简单的示例代码,用于连接到MySQL数据库,并查询学生成绩:
```
import java.sql.*;
public class StudentGradeQuery {
static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
static final String DB_URL = "jdbc:mysql://localhost/student";
static final String USER = "username";
static final String PASS = "password";
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
try{
Class.forName(JDBC_DRIVER);
conn = DriverManager.getConnection(DB_URL,USER,PASS);
String studentId = "your_student_id_here";
String sql = "SELECT * FROM grades WHERE student_id = " + studentId;
stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery(sql);
while(rs.next()){
String course = rs.getString("course");
int score = rs.getInt("score");
System.out.print("Course: " + course);
System.out.println(", Score: " + score);
}
rs.close();
stmt.close();
conn.close();
}catch(SQLException se){
se.printStackTrace();
}catch(Exception e){
e.printStackTrace();
}finally{
try{
if(stmt!=null)
stmt.close();
}catch(SQLException se2){
}
try{
if(conn!=null)
conn.close();
}catch(SQLException se){
se.printStackTrace();
}
}
}
}
```
您需要将以上代码中的“your_student_id_here”替换为您要查询的学生学号。
最后,将查询结果显示在GUI界面上。
这只是一个简单的示例,您需要根据自己的实际情况进行修改和完善。希望能对您有所帮助!
相关推荐
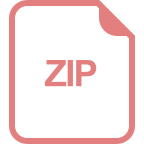
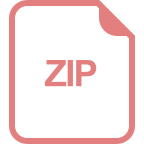














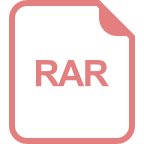