python线程池用法
时间: 2023-12-29 16:25:37 浏览: 96
以下是使用Python线程池的两种方法:
1. 使用第三方库threadpool:
```python
import threadpool
# 定义一个任务函数
def task_func(arg1, arg2):
# 执行任务的代码
pass
# 创建线程池
pool = threadpool.ThreadPool(5) # 创建一个包含5个线程的线程池
# 创建任务列表
tasks = threadpool.makeRequests(task_func, [(arg1, arg2), (arg1, arg2), ...]) # 创建多个任务,每个任务有不同的参数
# 将任务添加到线程池中
for task in tasks:
pool.putRequest(task)
# 等待所有任务完成
pool.wait()
# 关闭线程池
pool.dismissWorkers(5) # 关闭线程池中的5个线程
```
2. 使用Python3中的concurrent.futures.ThreadPoolExecutor库:
```python
from concurrent.futures import ThreadPoolExecutor
# 定义一个任务函数
def task_func(arg1, arg2):
# 执行任务的代码
pass
# 创建线程池
with ThreadPoolExecutor(max_workers=5) as executor: # 创建一个包含5个线程的线程池
# 提交任务给线程池
future1 = executor.submit(task_func, arg1, arg2) # 提交一个任务,并获取一个Future对象
future2 = executor.submit(task_func, arg1, arg2)
# ...
# 获取任务的结果
result1 = future1.result() # 获取任务1的结果
result2 = future2.result()
# ...
```
阅读全文
相关推荐















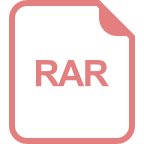
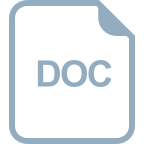