基于stm32的74HC595驱动代码
时间: 2023-08-13 18:05:59 浏览: 168
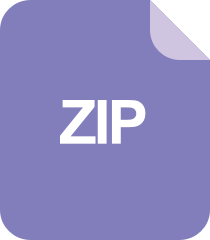
74HC595.zip_74HC595资料及代码_74hc595_74hc595 stm32_stm32 74HC595_st

好的,下面是基于STM32的74HC595驱动代码:
```c
#include "stm32f4xx.h"
#include "stm32f4xx_gpio.h"
#include "stm32f4xx_spi.h"
// 定义SPI口
#define SPIx SPI1
#define SPIx_CLK RCC_APB2Periph_SPI1
#define SPIx_IRQn SPI1_IRQn
#define SPIx_IRQHandler SPI1_IRQHandler
// 定义Latch口
#define LATCH_GPIO_PORT GPIOB
#define LATCH_GPIO_PIN GPIO_Pin_12
// 定义Data口
#define DATA_GPIO_PORT GPIOB
#define DATA_GPIO_PIN GPIO_Pin_15
// 定义Clock口
#define CLK_GPIO_PORT GPIOB
#define CLK_GPIO_PIN GPIO_Pin_13
// 定义74HC595控制引脚
#define HC595_LATCH_LOW() GPIO_ResetBits(LATCH_GPIO_PORT, LATCH_GPIO_PIN)
#define HC595_LATCH_HIGH() GPIO_SetBits(LATCH_GPIO_PORT, LATCH_GPIO_PIN)
#define HC595_CLOCK_LOW() GPIO_ResetBits(CLK_GPIO_PORT, CLK_GPIO_PIN)
#define HC595_CLOCK_HIGH() GPIO_SetBits(CLK_GPIO_PORT, CLK_GPIO_PIN)
#define HC595_SEND_BIT(value) GPIO_WriteBit(DATA_GPIO_PORT, DATA_GPIO_PIN, (BitAction)value)
void HC595_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
// 使能GPIOB时钟
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOB, ENABLE);
// 配置Latch口为推挽输出
GPIO_InitStructure.GPIO_Pin = LATCH_GPIO_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LATCH_GPIO_PORT, &GPIO_InitStructure);
// 配置Data口为推挽输出
GPIO_InitStructure.GPIO_Pin = DATA_GPIO_PIN;
GPIO_Init(DATA_GPIO_PORT, &GPIO_InitStructure);
// 配置Clock口为推挽输出
GPIO_InitStructure.GPIO_Pin = CLK_GPIO_PIN;
GPIO_Init(CLK_GPIO_PORT, &GPIO_InitStructure);
// 使能SPI时钟
RCC_APB2PeriphClockCmd(SPIx_CLK, ENABLE);
// 配置SPI口
SPI_InitTypeDef SPI_InitStructure;
SPI_InitStructure.SPI_Direction = SPI_Direction_1Line_Tx;
SPI_InitStructure.SPI_Mode = SPI_Mode_Master;
SPI_InitStructure.SPI_DataSize = SPI_DataSize_8b;
SPI_InitStructure.SPI_CPOL = SPI_CPOL_Low;
SPI_InitStructure.SPI_CPHA = SPI_CPHA_1Edge;
SPI_InitStructure.SPI_NSS = SPI_NSS_Soft;
SPI_InitStructure.SPI_BaudRatePrescaler = SPI_BaudRatePrescaler_128;
SPI_InitStructure.SPI_FirstBit = SPI_FirstBit_MSB;
SPI_Init(SPIx, &SPI_InitStructure);
// 使能SPI口
SPI_Cmd(SPIx, ENABLE);
}
void HC595_SendByte(uint8_t data)
{
int i;
// 禁止SPI口
SPI_Cmd(SPIx, DISABLE);
// 发送数据
for(i = 0; i < 8; i++)
{
HC595_CLOCK_LOW();
HC595_SEND_BIT((data & 0x80) >> 7);
data <<= 1;
HC595_CLOCK_HIGH();
}
// 使能SPI口
SPI_Cmd(SPIx, ENABLE);
}
void HC595_SendData(uint8_t *data, uint16_t length)
{
uint16_t i;
// 禁止SPI口
SPI_Cmd(SPIx, DISABLE);
// 发送数据
for(i = 0; i < length; i++)
{
HC595_SendByte(data[i]);
}
// 更新数据
HC595_LATCH_LOW();
HC595_LATCH_HIGH();
// 使能SPI口
SPI_Cmd(SPIx, ENABLE);
}
int main(void)
{
uint8_t data[2] = {0x01, 0x02};
HC595_Init();
while(1)
{
HC595_SendData(data, 2);
}
}
```
注意:74HC595的Data口需要接到SPI的MOSI(Master Output Slave Input)口,而Clock口需要接到SPI的SCK(Serial Clock)口。同时,还需要定义Latch口的引脚,将它作为74HC595的Latch口。以上代码中的SPI口配置为主机模式,每次发送1Byte的数据,波特率为128分频。
阅读全文
相关推荐
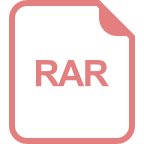
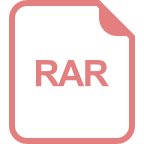
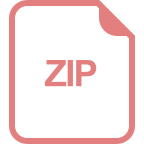


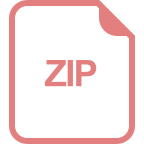
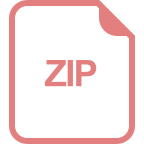
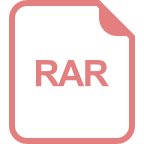
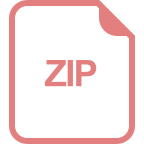
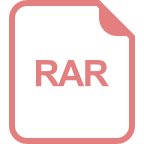
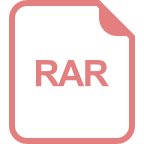
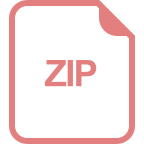
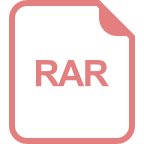
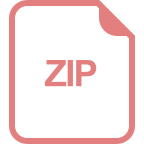
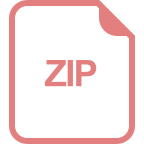