基于VHDL的串口收发实现
时间: 2023-06-15 08:05:30 浏览: 254
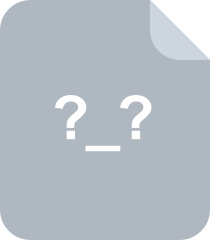
采用VHDL描写的一个串口收发程序

要实现基于VHDL的串口收发,首先需要了解串口通信的基本原理。串口通信是通过串行传输数据,即将数据按照位的顺序依次发送或接收。串口通信包含数据位、停止位和校验位等参数,需要在发送和接收端进行设置和匹配。
下面是一个基于VHDL的串口收发的简单实现:
```
entity uart is
generic(
clk_frequency: integer := 50_000_000; --时钟频率
baud_rate: integer := 9600; --波特率
data_bits: integer := 8; --数据位数
parity: string := "none"; --奇偶校验方式
stop_bits: integer := 1 --停止位数
);
port(
clk: in std_logic; --时钟
reset: in std_logic; --复位
tx: out std_logic; --发送端口
rx: in std_logic --接收端口
);
end uart;
architecture Behavioral of uart is
--内部信号
signal baud_tick: integer range 0 to clk_frequency/baud_rate-1 := 0; --波特率计数器
signal bit_count: integer range 0 to data_bits+stop_bits+1 := 0; --位计数器
signal data: std_logic_vector(data_bits-1 downto 0) := (others => '0'); --数据
signal parity_bit: std_logic := '0'; --奇偶校验位
signal stop_bit: std_logic := '1'; --停止位
signal tx_busy: std_logic := '0'; --发送忙标志
begin
--波特率计数器
baud_tick <= baud_tick + 1 when baud_tick /= clk_frequency/baud_rate-1 else 0;
--发送
tx <= '0' when tx_busy = '1' else stop_bit & data & parity_bit & stop_bit when bit_count = data_bits+stop_bits+1 else
'1' when bit_count = data_bits+stop_bits else
data(data_bits-1) when bit_count = 1 else
parity_bit when bit_count = data_bits+1 else
stop_bit when bit_count = data_bits+stop_bits+1 else
'1';
--接收
process(clk)
begin
if rising_edge(clk) then
if reset = '1' then
bit_count <= 0;
data <= (others => '0');
parity_bit <= '0';
stop_bit <= '1';
else
if rx = '0' and bit_count = 0 then --接收到起始位
bit_count <= 1;
elsif bit_count > 0 and bit_count <= data_bits then --接收数据位
data(bit_count-1) <= rx;
bit_count <= bit_count + 1;
elsif bit_count = data_bits+1 and parity /= "none" then --接收奇偶校验位
parity_bit <= rx;
bit_count <= bit_count + 1;
elsif bit_count = data_bits+1 and parity = "none" then --无奇偶校验
parity_bit <= '0';
bit_count <= bit_count + 1;
elsif bit_count = data_bits+2 then --接收停止位
stop_bit <= rx;
bit_count <= 0;
end if;
end if;
end if;
end process;
--发送数据
process(clk)
begin
if rising_edge(clk) then
if reset = '1' then
tx_busy <= '0';
else
if tx_busy = '1' then --发送忙
if bit_count = data_bits+stop_bits+1 then
tx_busy <= '0';
end if;
else
if bit_count > 0 then --开始发送
tx_busy <= '1';
bit_count <= bit_count + 1;
end if;
end if;
end if;
end if;
end process;
end Behavioral;
```
该实现中包含了发送和接收两个部分。发送部分包括波特率计数器、位计数器、数据、奇偶校验位、停止位和发送忙标志等信号。接收部分包括波特率计数器、位计数器、数据、奇偶校验位和停止位等信号。接收部分通过一个 process 进行处理,发送部分则通过另一个 process 处理。其中,tx_busy 标志表示发送忙,当发送忙时,将不会继续发送,直到发送完成。
需要注意的是,该实现中的波特率计数器是通过计算得到的,因此需要根据时钟频率和波特率设置相应的计数器值。此外,还需要根据实际需求设置数据位数、奇偶校验方式和停止位数等参数。
阅读全文
相关推荐
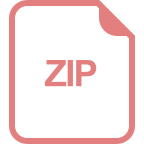
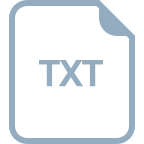
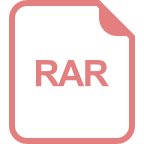
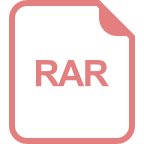
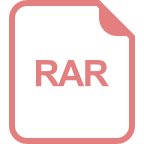
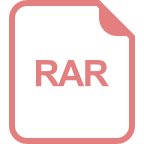
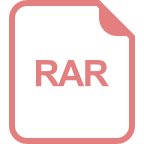
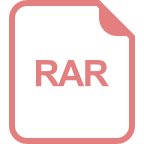

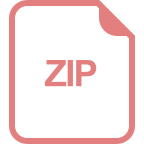
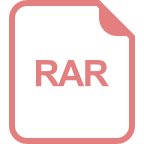
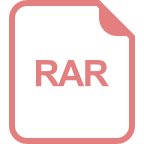
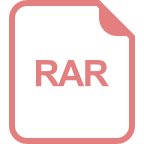
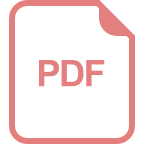
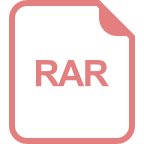
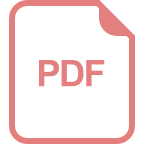