java 实现可逆的加密和解密
时间: 2023-12-22 21:04:20 浏览: 36
可以使用Java提供的加密类库实现可逆的加密和解密,常用的加密算法有DES、AES、RSA等。
以下是一个示例代码,使用AES算法对字符串进行加密和解密:
```java
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import java.util.Base64;
public class AesEncryptor {
private static final String ALGORITHM = "AES";
private static final int KEY_SIZE = 128;
public static String encrypt(String input, String password) throws Exception {
Cipher cipher = Cipher.getInstance(ALGORITHM);
SecretKey secretKey = generateKey(password);
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
byte[] encryptedBytes = cipher.doFinal(input.getBytes());
return Base64.getEncoder().encodeToString(encryptedBytes);
}
public static String decrypt(String input, String password) throws Exception {
Cipher cipher = Cipher.getInstance(ALGORITHM);
SecretKey secretKey = generateKey(password);
cipher.init(Cipher.DECRYPT_MODE, secretKey);
byte[] decryptedBytes = cipher.doFinal(Base64.getDecoder().decode(input));
return new String(decryptedBytes);
}
private static SecretKey generateKey(String password) throws Exception {
KeyGenerator keyGenerator = KeyGenerator.getInstance(ALGORITHM);
keyGenerator.init(KEY_SIZE);
return new SecretKeySpec(password.getBytes(), ALGORITHM);
}
}
```
使用示例:
```java
String input = "Hello World!";
String password = "myPassword";
String encrypted = AesEncryptor.encrypt(input, password);
System.out.println("Encrypted: " + encrypted);
String decrypted = AesEncryptor.decrypt(encrypted, password);
System.out.println("Decrypted: " + decrypted);
```
输出结果:
```
Encrypted: WVQJZTQzL3XqA3r+Jn7VfA==
Decrypted: Hello World!
```
相关推荐
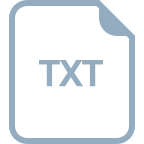










