Java中可逆的加密算法代码
时间: 2024-05-17 08:14:55 浏览: 123
在Java中,常见的可逆加密算法包括AES和DES算法。以下是一个使用AES算法进行加密解密的示例代码:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import java.util.Base64;
public class AesUtil {
//定义加密算法,ECB模式,PKCS5Padding填充
private static final String ALGORITHM_MODE_PADDING = "AES/CBC/PKCS5Padding";
//定义向量,必须是16位
private static final String IV_STRING = "1234567890123456";
//定义密钥,必须是16位
private static final String KEY = "1234567890123456";
/**
* AES加密
* @param content 待加密的字符串
* @return 加密后的字符串
* @throws Exception
*/
public static String encrypt(String content) throws Exception {
Cipher cipher = Cipher.getInstance(ALGORITHM_MODE_PADDING);
SecretKeySpec keySpec = new SecretKeySpec(KEY.getBytes(), "AES");
IvParameterSpec ivSpec = new IvParameterSpec(IV_STRING.getBytes());
cipher.init(Cipher.ENCRYPT_MODE, keySpec, ivSpec);
byte[] encrypted = cipher.doFinal(content.getBytes());
return Base64.getEncoder().encodeToString(encrypted);
}
/**
* AES解密
* @param content 待解密的字符串
* @return 解密后的字符串
* @throws Exception
*/
public static String decrypt(String content) throws Exception {
Cipher cipher = Cipher.getInstance(ALGORITHM_MODE_PADDING);
SecretKeySpec keySpec = new SecretKeySpec(KEY.getBytes(), "AES");
IvParameterSpec ivSpec = new IvParameterSpec(IV_STRING.getBytes());
cipher.init(Cipher.DECRYPT_MODE, keySpec, ivSpec);
byte[] encrypted = Base64.getDecoder().decode(content);
byte[] decrypted = cipher.doFinal(encrypted);
return new String(decrypted);
}
}
```
使用示例:
```java
public static void main(String[] args) throws Exception {
String content = "Hello, world!";
String encrypted = AesUtil.encrypt(content);
String decrypted = AesUtil.decrypt(encrypted);
System.out.println("加密前:" + content);
System.out.println("加密后:" + encrypted);
System.out.println("解密后:" + decrypted);
}
```
阅读全文
相关推荐
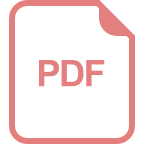
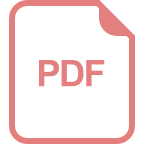
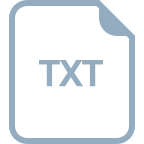
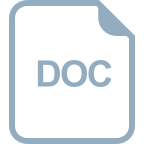
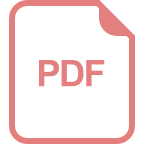
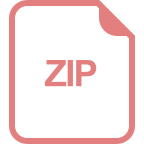
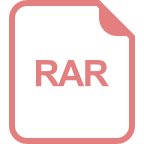
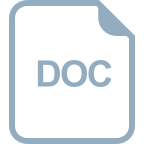
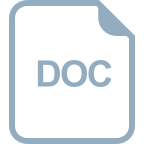
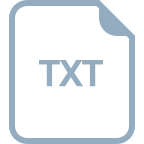
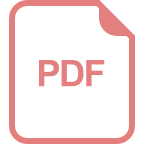
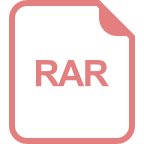
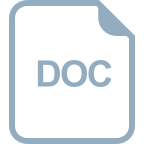
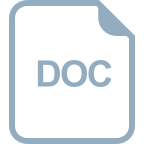
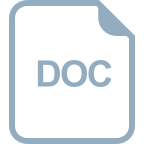