python删除字符串的空格
时间: 2023-09-02 16:08:40 浏览: 37
Python中可以使用字符串的`strip()`方法删除字符串的空格,该方法会删除字符串开头和结尾的空格。例如:
```python
s = " hello world "
s = s.strip()
print(s) # 输出:hello world
```
如果你想只删除开头或结尾的空格,可以分别使用`lstrip()`和`rstrip()`方法。例如:
```python
s = " hello world "
s = s.lstrip()
print(s) # 输出:hello world
s = " hello world "
s = s.rstrip()
print(s) # 输出: hello world
```
另外,如果你想删除字符串中间的空格,可以使用`replace()`方法,将空格替换成空字符串即可。例如:
```python
s = "hello world"
s = s.replace(" ", "")
print(s) # 输出:helloworld
```
相关问题
python 删掉字符串空格
在 Python 中,可以使用 `strip()` 方法来删除字符串两侧的空格,使用 `lstrip()` 方法来删除字符串左侧的空格,使用 `rstrip()` 方法来删除字符串右侧的空格。
示例代码如下:
```python
str1 = " hello world "
print(str1.strip()) # 输出 "hello world"
print(str1.lstrip()) # 输出 "hello world "
print(str1.rstrip()) # 输出 " hello world"
```
如果要删除字符串中间的空格,可以使用 `replace()` 方法来替换空格为空字符串,示例代码如下:
```python
str1 = "hello world"
str2 = str1.replace(" ", "")
print(str2) # 输出 "helloworld"
```
python去除字符串空格
您好,关于python去除字符串空格,您可以使用strip()函数来实现,具体方法为:
str.strip():去除字符串两端的空格
str.lstrip():去除字符串左侧的空格
str.rstrip():去除字符串右侧的空格
示例代码如下:
``` python
s = " hello world "
s.strip() # 去除两端空格,输出:'hello world'
s.lstrip() # 去除左空格,输出:'hello world '
s.rstrip() # 去除右空格,输出:' hello world'
```
希望对您有所帮助。
相关推荐
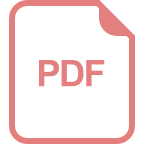
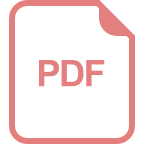










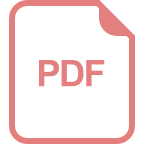
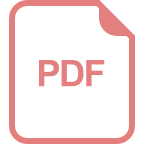