ESP32使用HttpClient库实现一个POST请求发送一个音频文件加一个字符串的功能
时间: 2024-05-09 17:17:24 浏览: 12
以下是一个使用HttpClient库在ESP32上发送POST请求发送音频文件和字符串的示例代码:
```C++
#include <WiFi.h>
#include <HTTPClient.h>
#include <SD.h>
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
const char* serverUrl = "http://example.com/upload.php";
const char* filePath = "/audio.wav";
const char* paramName = "audio_file";
const char* paramValue = "my_audio_file";
const char* stringParamName = "my_string";
const char* stringParamValue = "Hello World";
void setup() {
Serial.begin(115200);
// Connect to Wi-Fi
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
// Initialize SD card
if (!SD.begin()) {
Serial.println("SD card initialization failed");
while (1);
}
Serial.println("SD card initialized");
// Open file
File file = SD.open(filePath);
if (!file) {
Serial.println("Failed to open file");
while (1);
}
Serial.println("File opened");
// Calculate file size
size_t fileSize = file.size();
Serial.print("File size: ");
Serial.println(fileSize);
// Create HTTPClient object
HTTPClient http;
// Add audio file to request
http.begin(serverUrl);
http.addHeader("Content-Type", "multipart/form-data");
http.addFile(paramName, filePath, fileSize, paramValue);
// Add string parameter to request
http.addHeader("Content-Type", "application/x-www-form-urlencoded");
String data = String(stringParamName) + "=" + String(stringParamValue);
http.POST(data);
// Get response
int httpResponseCode = http.POST();
String response = http.getString();
Serial.println(httpResponseCode);
Serial.println(response);
// Cleanup
http.end();
file.close();
}
void loop() {
// Do nothing
}
```
在上面的代码中,我们首先连接到Wi-Fi网络并初始化SD卡。然后,我们打开要发送的音频文件并计算其大小。接下来,我们创建一个HTTPClient对象并将音频文件添加到请求中。我们还添加了一个字符串参数到请求中。最后,我们发送POST请求并获取响应。注意,我们调用了两次`http.POST()`,一次将音频文件添加到请求中,一次将字符串参数添加到请求中。
在实际使用中,您需要将上面的代码中的`your_SSID`和`your_PASSWORD`替换为您的Wi-Fi网络的凭据,将`http://example.com/upload.php`替换为您的服务器URL,将`/audio.wav`替换为您要发送的音频文件的路径,将`my_audio_file`替换为您要在服务器上存储文件的名称,将`my_string`替换为您要发送的字符串参数的名称,将`Hello World`替换为您要发送的字符串参数的值。
另外,请确保您的服务器端代码正确处理POST请求并保存音频文件和字符串参数。
相关推荐
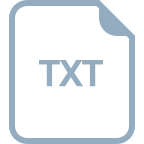
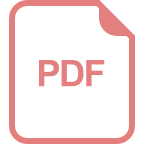
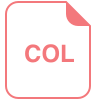













