js undifined和null的区别
时间: 2023-05-31 11:20:44 浏览: 62
### 回答1:
JavaScript中的undefined和null都表示没有值,但它们有一些不同之处。
undefined表示一个变量已经声明但没有被赋值,或者一个对象属性不存在。例如:
```
var x;
console.log(x); // 输出undefined
var obj = {};
console.log(obj.prop); // 输出undefined
```
null表示一个变量或对象属性被明确地赋值为null,表示它没有值。例如:
```
var y = null;
console.log(y); // 输出null
var obj = { prop: null };
console.log(obj.prop); // 输出null
```
总的来说,undefined表示缺少值,而null表示有一个值,但这个值是空的。
### 回答2:
在JavaScript中,undefined和null都表示缺失或不存在的值,但它们有区别。
undefined表示变量声明了但没有给定值,或者调用对象属性时不存在该属性。例如:
var a; // 定义了变量但没给定值,a就是undefined
var b = {};
console.log(b.x); // b对象没有x属性,b.x就是undefined
null表示可以对某个变量赋空值,即给变量一个空的值。例如:
var a = null; // a表示空值
当你尝试使用undefined值时,可能会出现一些问题,而使用null不会。例如:
var a;
console.log(a + 1); // 输出NaN,Undefined与任何值的运算都是NaN
var b = null;
console.log(b + 1); // 输出1,null被自动转换为数字0
在JavaScript中,很多函数会返回null表示某个值不存在,但函数运行正常,而返回undefined可以表示函数运行出现了问题。例如:
function parseNumber(str) {
var n = Number(str);
if(isNaN(n)) {
return null;
} else {
return n;
}
}
var a = parseNumber('abc');
if(a === null) {
console.log("输入不是数字");
}
函数parseNumber会尝试将字符串转换为数字,如果输入的不是数字,返回null表示失败。如果函数遇到问题,应该返回undefined表示函数未正常执行。
总结而言,undefined表示声明变量但没有值或者调用对象不存在的属性,null表示可以给变量赋空值或者表示不存在的值。在函数中,返回null表示函数未能成功完成,返回undefined表示函数出现了问题。
### 回答3:
JS中的undefined和null是两个表示空或未定义的值。虽然它们是相似的,但是它们有一些区别。
Undefined是一种表示值为空或不存在的状态的原始类型。当我们尝试访问不存在的变量或属性时,它就会返回undefined。例如:
```
let x;
console.log(x); // undefined
```
此外,当我们没有将函数返回值明确设置为任何内容时,该函数将默认返回undefined。例如:
```
function foo() {}
console.log(foo()); // undefined
```
相比之下,null表示一个空的对象引用,它通常在代码中使用,表示没有任何有效值或空对象。例如:
```
const user = null;
console.log(user); // null
```
当我们想要明确表示某个值为空时,通常使用null。此外,null表示一个对象已经被显式地设置为空对象。
尽管undefined和null看起来非常相似,但在某些情况下,它们的行为可能不同。例如:
```
let x = undefined;
let y = null;
console.log(typeof x); // "undefined"
console.log(typeof y); // "object"
```
在这种情况下,typeof运算符返回不同的结果,说明undefined和null有差异。此外,当我们尝试将undefined与null进行比较时,它们的比较结果不相等。例如:
```
console.log(undefined == null); // true
console.log(undefined === null); // false
```
因此,虽然undefined和null都可以表示“空”或“未定义”的状态,但它们在某些方面表现出不同的行为,需要格外小心。
相关推荐
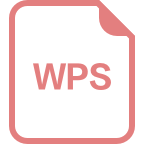
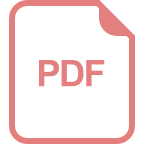













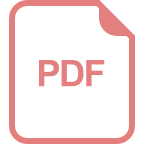
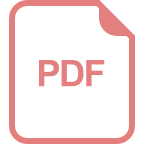