show me an example how to design a C++ monadic I/O on reading and writing a regular file.
时间: 2024-12-19 12:19:16 浏览: 12
设计C++的monadic I/O(函数式编程风格的I/O操作)通常会利用`std::experimental::io_context`和相关的流适配器,比如`std::io_context::work`, `std::async`, 和`std::piped_stream`等。以下是一个简单的例子,展示如何创建一个读写文件的基本monadic API:
```cpp
#include <iostream>
#include <fstream>
#include <future>
#include <asio.hpp>
// Monadic IO function for reading from a file
template <typename Stream, typename Result>
auto read_file(Stream& stream, Result&& result)
{
return [stream = std::move(stream), result = std::forward<Result>(result)] {
auto future_read = std::async(std::launch::async, [] (Stream& s) -> std::string {
std::stringstream ss;
ss << s.rdbuf();
return ss.str();
}, std::ref(stream));
// Wait for the async operation to complete
auto content = future_read.get();
// Use the result in your monadic chain
result(content);
};
}
// Monadic IO function for writing to a file
template <typename Stream, typename Input>
auto write_file(Stream& stream, Input input)
{
return [stream = std::move(stream)](Input input) mutable {
stream << input;
return stream.commit(); // Commit the write operation
};
}
int main()
{
try
{
std::ifstream reader("input.txt");
std::ofstream writer("output.txt");
// Monadic chaining of read and write operations
io_context ioc;
auto read_task = read_file(reader, [&](const std::string& content) {
auto write_task = write_file(writer, content);
ioc.run(write_task);
});
ioc.run(read_task);
}
catch (std::exception& e)
{
std::cerr << "Error: " << e.what() << '\n';
}
return 0;
}
```
在这个示例中,`read_file`和`write_file`都是接受输入流并返回一个新的函数,这个新函数用于继续链式操作。通过这种方式,你可以将一系列复杂的I/O操作组合在一起,形成一个简洁、易理解的序列。
阅读全文
相关推荐
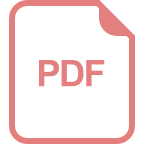
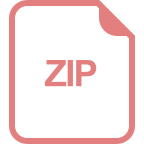
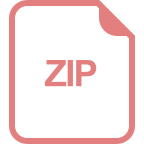
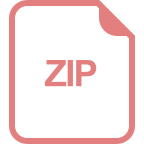
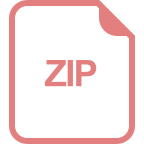
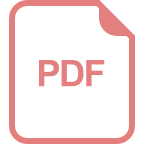
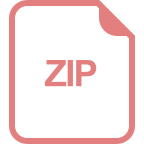
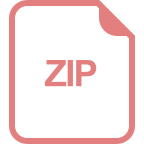
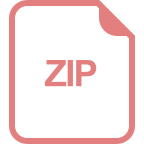
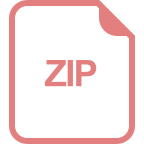
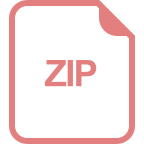
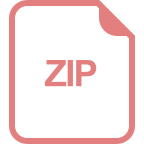
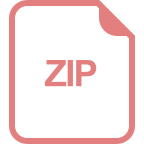
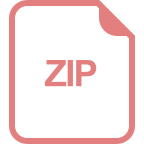
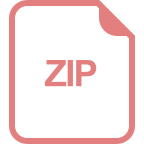
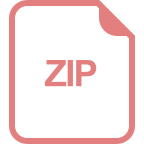
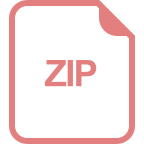
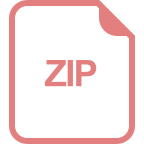
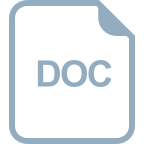