用java编写程序,模拟猜拳游戏,循环输入两个玩家的手型,判断输赢后输出并退出循环。要求断言输入的数据为 0、1、2,否则输出异常。
时间: 2024-12-11 15:30:17 浏览: 4
在Java中,我们可以创建一个简单的命令行猜拳游戏,使用`Scanner`接收用户的输入,然后通过`switch`语句来判断结果。我们将使用断言`assert`来验证输入的有效性。以下是实现这个游戏的一个简单示例:
```java
import java.util.Scanner;
public class RockPaperScissorsGame {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
while (true) {
System.out.println("请输入玩家一的手型(0:石头,1:剪刀,2:布),输入0到2,否则退出:");
int playerOneHand = input.nextInt();
// 断言输入的有效性
assert playerOneHand >= 0 && playerOneHand <= 2 : "非法输入,只能选择0, 1, 或者2";
System.out.println("请输入玩家二的手型:");
int playerTwoHand = input.nextInt();
// 同样的断言
assert playerTwoHand >= 0 && playerTwoHand <= 2 : "非法输入,只能选择0, 1, 或者2";
switch (playerOneHand) {
case 0: // 石头
if (playerTwoHand == 0) {
System.out.println("平局!双方都选择了石头。");
} else if (playerTwoHand == 1) {
System.out.println("玩家二赢了!剪刀胜过石头。");
} else {
System.out.println("玩家一赢了!石头胜过布。");
}
break;
// 添加对其他手型的判断
// ...
default:
System.out.println("非法输入!请选择0, 1, 或者2.");
break;
}
System.out.print("是否继续游戏?(y/n): ");
String continueChoice = input.next();
if (!continueChoice.equalsIgnoreCase("y")) {
break;
}
}
input.close();
}
}
```
在这个游戏中,当用户输入合法的数字0, 1, 或者2时,程序会根据玩家的手型进行判断。如果用户不想继续,可以选择"n"退出循环。
阅读全文
相关推荐
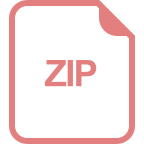
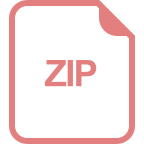
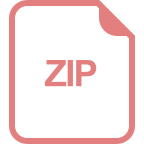













