在labeling的yolo模式中,用python统计一个有预标框的多个txt文件的文件夹中,统计手动拉框的数量
时间: 2024-09-15 14:03:39 浏览: 53
在YOLO的标注过程中,如果你有一个包含多个`.txt`文件(每个对应一张图片的预标注框)的文件夹,通常这些文件会记录物体位置、类别以及其他可能的属性。为了统计手动拉框的数量,我们可以按照以下步骤操作:
1. 使用Python的`os`模块遍历文件夹及其所有`.txt`文件:
```python
import os
folder_path = 'path_to_your_labelled_folder'
files = [f for f in os.listdir(folder_path) if f.endswith('.txt')]
```
2. 然后,对于每一个文件,我们需要加载数据并检查哪些框是经过人工调整的。这可以通过比较预标注框(通常是 `_pre.txt` 文件)和修改后的框(可能是 `_modified.txt` 或其他命名的文件)来完成。假设每个文件都遵循相同的格式,我们可以创建一个函数来读取文件并对修改过的框计数:
```python
def count_manual_boxes(file_path_pre, file_path_mod):
with open(file_path_pre, 'r') as pre_file, open(file_path_mod, 'r') as mod_file:
pre_lines = pre_file.readlines()
mod_lines = mod_file.readlines()
pre_boxes = [line.strip().split(' ') for line in pre_lines]
mod_boxes = [line.strip().split(' ') for line in mod_lines]
manual_boxes = [True if tuple(pre_box) != tuple(mod_box) else False for pre_box, mod_box in zip(pre_boxes, mod_boxes)]
return sum(manual_boxes)
# 获取每个文件的修改框计数
file_counts = {}
for txt_file in files:
if '_pre.txt' in txt_file:
base_name = txt_file.split('_pre.txt')[0]
mod_file_name = base_name + '_modified.txt' # 假设修改后的文件是以这种方式命名的
file_counts[txt_file] = count_manual_boxes(txt_file, mod_file_name)
```
3. 最终,`file_counts` 字典将包含每个文件手动拉框的数量,你可以通过`sum(file_counts.values())`来得到整个文件夹里手动拉框总数。
阅读全文
相关推荐
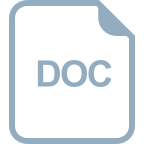
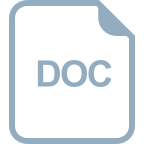
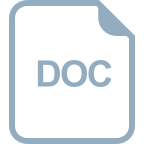
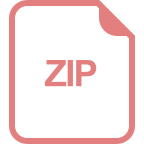
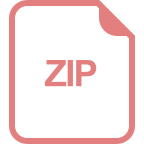
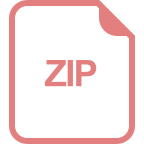
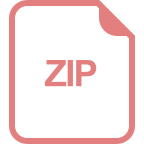
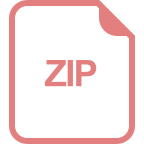
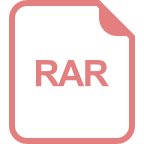
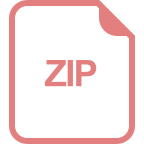
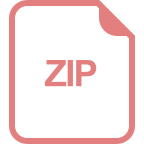
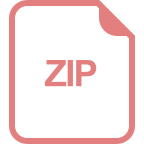
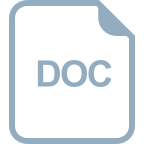
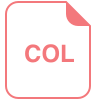




