c语言16进制转浮点
时间: 2024-09-11 11:13:19 浏览: 73
在C语言中,将十六进制字符串转换为浮点数通常需要几个步骤,因为C标准库本身并不直接提供这种转换函数。你可以通过手动解析十六进制字符串并逐位转换成二进制,然后利用`FLT_RADIX`宏确定的基数(通常为10)乘以对应的值来完成转换。这里是一个简单的示例:
```c
#include <stdio.h>
#include <float.h>
// 十六进制字符到十进制的转换函数
int hex_to_decimal(char hex) {
if (hex >= '0' && hex <= '9') return hex - '0';
else if (hex >= 'A' && hex <= 'F') return hex - 'A' + 10;
else if (hex >= 'a' && hex <= 'f') return hex - 'a' + 10;
return -1; // 非法字符处理
}
double hex_to_float(const char *hex_string) {
double result = 0.0;
int scale = 0;
while (*hex_string != '\0') {
int digit = hex_to_decimal(*hex_string);
if (digit == -1) break; // 非法字符处理
// 如果当前字符表示小数部分,先左移scale位再加
if (*hex_string == '.') {
scale = 1;
} else {
result += digit * pow(16, scale++);
}
hex_string++;
}
return result;
}
int main() {
const char* hex_str = "FF.FF";
double float_value = hex_to_float(hex_str);
printf("Hexadecimal %s converted to float is %.2f\n", hex_str, float_value);
return 0;
}
```
阅读全文
相关推荐
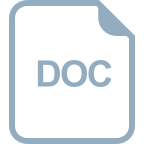
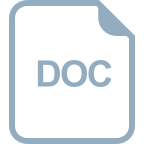
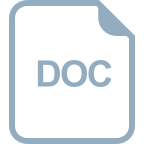







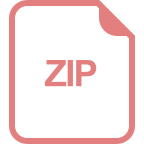
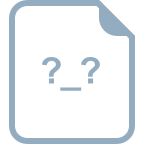
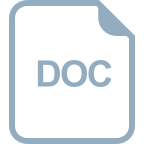




