用C sharp将List中的数据导入Excel文件中
时间: 2023-05-27 22:05:31 浏览: 150
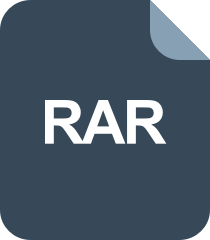
C#数据导入和导出.rar
以下是使用C#将List中的数据导入Excel文件的示例代码:
```csharp
using System;
using System.Collections.Generic;
using Excel = Microsoft.Office.Interop.Excel;
namespace ConsoleApp
{
class Program
{
static void Main(string[] args)
{
List<Person> people = new List<Person>
{
new Person { Name = "Alice", Age = 25, City = "New York" },
new Person { Name = "Bob", Age = 30, City = "Los Angeles" },
new Person { Name = "Charlie", Age = 35, City = "Chicago" }
};
// Create a new Excel workbook and sheet
Excel.Application excel = new Excel.Application();
excel.Visible = true;
Excel.Workbook workbook = excel.Workbooks.Add();
Excel.Worksheet sheet = workbook.ActiveSheet;
// Write the list data to the Excel sheet
sheet.Cells[1, 1] = "Name";
sheet.Cells[1, 2] = "Age";
sheet.Cells[1, 3] = "City";
int row = 2;
foreach (Person person in people)
{
sheet.Cells[row, 1] = person.Name;
sheet.Cells[row, 2] = person.Age;
sheet.Cells[row, 3] = person.City;
row++;
}
// Save the Excel workbook
workbook.SaveAs(@"C:\Temp\People.xlsx");
workbook.Close();
excel.Quit();
}
}
class Person
{
public string Name { get; set; }
public int Age { get; set; }
public string City { get; set; }
}
}
```
在上述示例代码中,我们使用了Microsoft Office Interop Excel库来创建Excel工作簿和工作表,并将List中的数据写入工作表中。最后,我们将工作簿保存到指定的文件路径,并关闭Excel应用程序。请注意,您需要在项目中引用Microsoft Office Interop Excel库(可以从NuGet获取)。
阅读全文
相关推荐
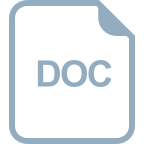
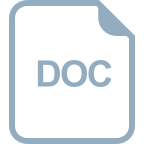
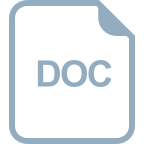
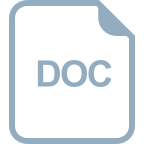
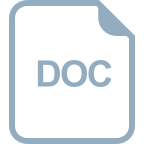
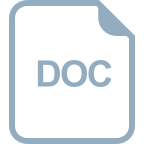
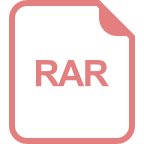
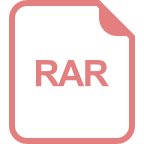
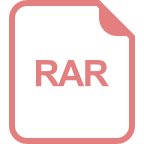
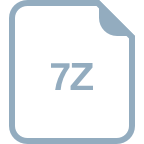
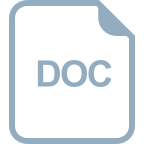
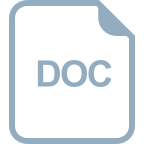
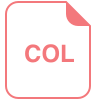
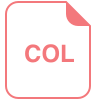




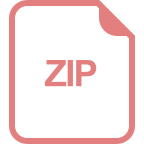