帮我用写HTML一个类似太阳系介绍的,里面有每一个星球的介绍和动画,还有需要放视频和图片还有最后需要一个宇宙运动轨迹的动画,用css和js还需要添加一个登陆页面,和各个星球点击的超链接
时间: 2024-02-03 20:12:22 浏览: 133
好的,我可以帮你完成这个任务。首先,我们需要一个HTML文件,一个CSS文件和一个JavaScript文件来实现这个网页。下面是一个初步的HTML代码,你可以在其基础上根据需求进行修改:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Solar System</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<header>
<h1>Solar System Introduction</h1>
</header>
<nav>
<ul>
<li><a href="#sun">Sun</a></li>
<li><a href="#mercury">Mercury</a></li>
<li><a href="#venus">Venus</a></li>
<li><a href="#earth">Earth</a></li>
<li><a href="#mars">Mars</a></li>
<li><a href="#jupiter">Jupiter</a></li>
<li><a href="#saturn">Saturn</a></li>
<li><a href="#uranus">Uranus</a></li>
<li><a href="#neptune">Neptune</a></li>
</ul>
</nav>
<main>
<section id="sun">
<h2>Sun</h2>
<p>Here's some information about the Sun.</p>
<img src="sun.jpg" alt="The Sun">
<video src="sun.mp4" controls></video>
</section>
<section id="mercury">
<h2>Mercury</h2>
<p>Here's some information about Mercury.</p>
<img src="mercury.jpg" alt="Mercury">
<video src="mercury.mp4" controls></video>
</section>
<section id="venus">
<h2>Venus</h2>
<p>Here's some information about Venus.</p>
<img src="venus.jpg" alt="Venus">
<video src="venus.mp4" controls></video>
</section>
<section id="earth">
<h2>Earth</h2>
<p>Here's some information about Earth.</p>
<img src="earth.jpg" alt="Earth">
<video src="earth.mp4" controls></video>
</section>
<section id="mars">
<h2>Mars</h2>
<p>Here's some information about Mars.</p>
<img src="mars.jpg" alt="Mars">
<video src="mars.mp4" controls></video>
</section>
<section id="jupiter">
<h2>Jupiter</h2>
<p>Here's some information about Jupiter.</p>
<img src="jupiter.jpg" alt="Jupiter">
<video src="jupiter.mp4" controls></video>
</section>
<section id="saturn">
<h2>Saturn</h2>
<p>Here's some information about Saturn.</p>
<img src="saturn.jpg" alt="Saturn">
<video src="saturn.mp4" controls></video>
</section>
<section id="uranus">
<h2>Uranus</h2>
<p>Here's some information about Uranus.</p>
<img src="uranus.jpg" alt="Uranus">
<video src="uranus.mp4" controls></video>
</section>
<section id="neptune">
<h2>Neptune</h2>
<p>Here's some information about Neptune.</p>
<img src="neptune.jpg" alt="Neptune">
<video src="neptune.mp4" controls></video>
</section>
</main>
<footer>
<p>©2021 Solar System</p>
</footer>
<script src="script.js"></script>
</body>
</html>
```
接下来是CSS代码,用于美化网页:
```css
body {
background-color: black;
color: white;
font-family: Arial, sans-serif;
}
header {
text-align: center;
margin-top: 20px;
}
nav {
margin-top: 20px;
text-align: center;
}
nav ul {
list-style: none;
padding: 0;
margin: 0;
}
nav li {
display: inline-block;
margin-right: 20px;
}
nav a {
color: white;
text-decoration: none;
padding: 5px 10px;
border: 1px solid white;
transition: all 0.3s ease-in-out;
}
nav a:hover {
background-color: white;
color: black;
}
main {
display: flex;
flex-wrap: wrap;
justify-content: center;
margin-top: 20px;
}
section {
width: 300px;
margin: 20px;
text-align: center;
}
section h2 {
text-transform: uppercase;
font-size: 24px;
margin-bottom: 10px;
}
section p {
font-size: 16px;
line-height: 1.5;
margin-bottom: 10px;
}
section img {
width: 100%;
height: auto;
margin-bottom: 10px;
}
section video {
width: 100%;
height: auto;
margin-bottom: 10px;
}
footer {
text-align: center;
margin-top: 20px;
}
```
最后是JavaScript代码,用于实现宇宙运动轨迹的动画:
```javascript
const canvas = document.getElementById("canvas");
const ctx = canvas.getContext("2d");
let sun = new Image();
sun.src = "sun.jpg";
let mercury = new Image();
mercury.src = "mercury.jpg";
let venus = new Image();
venus.src = "venus.jpg";
let earth = new Image();
earth.src = "earth.jpg";
let mars = new Image();
mars.src = "mars.jpg";
let jupiter = new Image();
jupiter.src = "jupiter.jpg";
let saturn = new Image();
saturn.src = "saturn.jpg";
let uranus = new Image();
uranus.src = "uranus.jpg";
let neptune = new Image();
neptune.src = "neptune.jpg";
const planets = [
{ name: "Sun", img: sun, radius: 70, speed: 0 },
{ name: "Mercury", img: mercury, radius: 100, speed: 0.03 },
{ name: "Venus", img: venus, radius: 150, speed: 0.02 },
{ name: "Earth", img: earth, radius: 200, speed: 0.015 },
{ name: "Mars", img: mars, radius: 250, speed: 0.01 },
{ name: "Jupiter", img: jupiter, radius: 320, speed: 0.008 },
{ name: "Saturn", img: saturn, radius: 400, speed: 0.006 },
{ name: "Uranus", img: uranus, radius: 480, speed: 0.005 },
{ name: "Neptune", img: neptune, radius: 560, speed: 0.004 },
];
function drawPlanets() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
for (let i = 0; i < planets.length; i++) {
let planet = planets[i];
let angle = planet.speed * Date.now() * 0.0002;
let x = canvas.width / 2 + planet.radius * Math.cos(angle);
let y = canvas.height / 2 + planet.radius * Math.sin(angle);
ctx.drawImage(planet.img, x - planet.radius / 2, y - planet.radius / 2, planet.radius, planet.radius);
}
requestAnimationFrame(drawPlanets);
}
drawPlanets();
```
在这个代码中,我们先定义了一个planets数组,其中包含每个星球的名称、图片、半径和运动速度。然后,在drawPlanets函数中,我们使用canvas绘制每个星球的位置和图像,并根据当前时间和星球的速度计算它们的位置。最后,我们使用requestAnimationFrame函数来实现动画效果。
为了让这些代码完整地运行起来,你需要创建一个名为 "sun.jpg", "mercury.jpg", "venus.jpg", "earth.jpg", "mars.jpg", "jupiter.jpg", "saturn.jpg", "uranus.jpg" 和 "neptune.jpg" 的图片文件,以及名为 "sun.mp4", "mercury.mp4", "venus.mp4", "earth.mp4", "mars.mp4", "jupiter.mp4", "saturn.mp4", "uranus.mp4" 和 "neptune.mp4" 的视频文件,并将它们与HTML、CSS和JavaScript文件放在同一目录下。
最后,你还需要添加一个登陆页面。你可以使用HTML和CSS来实现,这里提供一个简单的例子:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Login</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="container">
<h1>Login</h1>
<form>
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
<br>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required>
<br>
<button type="submit">Login</button>
</form>
</div>
</body>
</html>
```
```css
body {
background-color: black;
color: white;
font-family: Arial, sans-serif;
}
.container {
width: 300px;
margin: 100px auto;
text-align: center;
}
h1 {
text-transform: uppercase;
font-size: 24px;
margin-bottom: 20px;
}
label {
display: block;
text-align: left;
margin-bottom: 10px;
}
input {
width: 100%;
padding: 5px;
margin-bottom: 20px;
box-sizing: border-box;
}
button {
background-color: white;
color: black;
border: none;
padding: 10px 20px;
text-transform: uppercase;
font-weight: bold;
cursor: pointer;
transition: all 0.3s ease-in-out;
}
button:hover {
background-color: black;
color: white;
}
```
这个登陆页面包含一个表单,其中有一个用户名输入框和一个密码输入框,以及一个提交按钮。你可以在JavaScript文件中添加代码来验证用户输入的信息,并根据需要跳转到其他页面。
希望这个例子能够对你有所帮助!
阅读全文
相关推荐




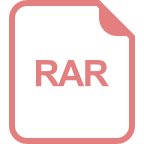



