city = soup.find('div', class_='crumbs fl').find('a')[-1].text.strip()报错如何修改
时间: 2023-09-04 16:08:54 浏览: 45
这段代码的作用是从HTML文档中找到class为'crumbs fl'的div标签,然后找到该div标签下的最后一个a标签,提取其文本内容,并去掉首尾的空格,将结果赋值给变量city。
如果这段代码报错,可能是因为soup对象没有找到class为'crumbs fl'的div标签或该div标签下没有a标签。这种情况下,可以先检查一下HTML文档的结构,确保要找的标签存在。另外,还可以加上一些判断,例如:
```python
crumbs_div = soup.find('div', class_='crumbs fl')
if crumbs_div:
city_a = crumbs_div.find_all('a')[-1]
if city_a:
city = city_a.text.strip()
else:
city = None
else:
city = None
```
这样,即使某个标签不存在,代码也不会报错,而是会将city赋值为None。
相关问题
import requestsfrom bs4 import BeautifulSoup# 构造请求urlurl = 'https://www.tianqi.com/zhongmou/10/'# 发送GET请求headers={'User-Agent':'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/114.0.0.0 Safari/537.36 Edg/114.0.1823.43'}response = requests.get(url,headers=headers)# 判断请求是否成功if response.status_code == 200: # 解析HTML页面 soup = BeautifulSoup(response.text, 'html.parser') # 获取天气信息 city = soup.find_all('ul class_="weaul"', class_='crumbs fl').find_all('a')[-1].text.strip() weather = soup.find_all('ul class_="weaul"', class_='wea').text.strip() temperature = soup.fin_alld('ul class_="weaul"', class_='tem').span.text.strip() wind = soup.find_all('ul class_="weaul"', class_='').i.text.strip() humidity = soup.find_all('ul class_="weaul"', class_='humidity').span.text.strip() # 将天气信息写入文本文件 with open('weather.txt', 'w', encoding='utf-8') as f: f.write(f"城市:{city}\n天气:{weather}\n温度:{temperature}\n风力:{wind}\n湿度:{humidity}")else: print("请求天气预报数据失败!")检查并修改以上程序
在程序中,以下代码存在错误:
```
city = soup.find_all('ul class_="weaul"', class_='crumbs fl').find_all('a')[-1].text.strip()
weather = soup.find_all('ul class_="weaul"', class_='wea').text.strip()
temperature = soup.fin_alld('ul class_="weaul"', class_='tem').span.text.strip()
wind = soup.find_all('ul class_="weaul"', class_='').i.text.strip()
humidity = soup.find_all('ul class_="weaul"', class_='humidity').span.text.strip()
```
应将上述代码修改为:
```
city = soup.find('div', class_='crumbs fl').find_all('a')[-1].text.strip()
weather = soup.find('ul', class_='weaul').find('p', class_='wea').text.strip()
temperature = soup.find('ul', class_='weaul').find('p', class_='tem').span.text.strip()
wind = soup.find('ul', class_='weaul').find('p', class_='win').i.text.strip()
humidity = soup.find('ul', class_='weaul').find('p', class_='hum').span.text.strip()
```
修改后的代码如下:
```
import requests
from bs4 import BeautifulSoup
# 构造请求url
url = 'https://www.tianqi.com/zhongmou/10/'
# 发送GET请求
headers={'User-Agent':'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/114.0.0.0 Safari/537.36 Edg/114.0.1823.43'}
response = requests.get(url,headers=headers)
# 判断请求是否成功
if response.status_code == 200:
# 解析HTML页面
soup = BeautifulSoup(response.text, 'html.parser')
# 获取天气信息
city = soup.find('div', class_='crumbs fl').find_all('a')[-1].text.strip()
weather = soup.find('ul', class_='weaul').find('p', class_='wea').text.strip()
temperature = soup.find('ul', class_='weaul').find('p', class_='tem').span.text.strip()
wind = soup.find('ul', class_='weaul').find('p', class_='win').i.text.strip()
humidity = soup.find('ul', class_='weaul').find('p', class_='hum').span.text.strip()
# 将天气信息写入文本文件
with open('weather.txt', 'w', encoding='utf-8') as f:
f.write(f"城市:{city}\n天气:{weather}\n温度:{temperature}\n风力:{wind}\n湿度:{humidity}")
else:
print("请求天气预报数据失败!")
```
这样就可以正确的获取天气信息并将其写入文本文件中了。
body_data = soup.body.get_text() content = soup
在你提供的代码片段中,你使用了 BeautifulSoup 库来解析 HTML 页面,并从中提取文本内容。
根据代码片段,你将整个 `<body>` 标签的文本内容赋值给 `body_data` 变量。然后,你将整个解析后的 HTML 页面对象赋值给 `content` 变量。
如果你想从 HTML 页面中提取特定的信息,你可以使用 BeautifulSoup 的各种方法和选择器来定位和提取所需的元素。例如,你可以使用 `find()` 或 `find_all()` 方法来查找特定的标签或 CSS 类名,并提取它们的文本内容。
以下是一个示例代码,展示如何使用 BeautifulSoup 提取 HTML 页面中的特定元素:
```python
from bs4 import BeautifulSoup
# 假设 html 是你要解析的 HTML 页面内容
html = """
<html>
<body>
<div class="content">
<h1>标题</h1>
<p>段落内容</p>
</div>
</body>
</html>
"""
# 创建 BeautifulSoup 对象
soup = BeautifulSoup(html, 'html.parser')
# 使用选择器提取特定元素
title = soup.find('h1').get_text()
paragraph = soup.find('p').get_text()
print(title) # 输出:标题
print(paragraph) # 输出:段落内容
```
你可以根据自己的需求修改示例代码,并使用合适的选择器来提取所需的信息。
相关推荐
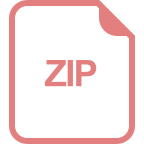












