在ads1.2环境下实现程序,要求:使用汇编程序编写一段串拷贝程序,使用c语言编程调用串拷贝程序实现将一个字符串的内容拷贝到另一个字符串。
时间: 2024-02-03 11:14:47 浏览: 80
以下是一个使用汇编程序编写的串拷贝程序:
```asm
section .data
source db 'Hello, World!', 0
destination db 20 dup(0)
section .text
global copyString
copyString:
push ebp
mov ebp, esp
mov esi, [ebp + 8] ; source
mov edi, [ebp + 12] ; destination
cld
mov ecx, 0xFFFFFFFF ; set ecx to maximum value to copy entire string
xor eax, eax ; clear eax
repne scasb ; find end of source string
not ecx ; calculate length of source string
rep movsb ; copy string
mov esp, ebp
pop ebp
ret
```
然后,我们可以使用C语言编写一个调用这个汇编程序的函数:
```c
void copyString(char* source, char* destination);
int main()
{
char source[] = "Hello, World!";
char destination[20];
copyString(source, destination);
printf("Source string: %s\n", source);
printf("Destination string: %s\n", destination);
return 0;
}
void copyString(char* source, char* destination)
{
__asm
{
push destination
push source
call copyString
}
}
```
注意,在调用汇编程序时,需要先将destination和source压入栈中。
阅读全文
相关推荐
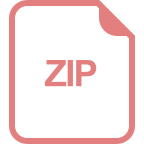
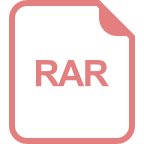
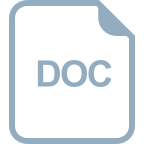
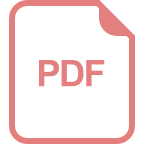
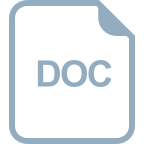
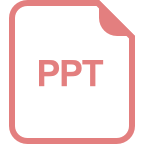
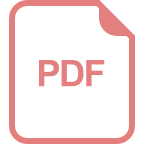
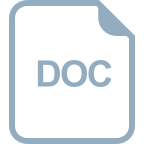
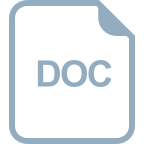
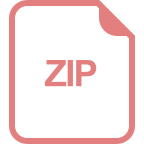
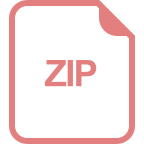
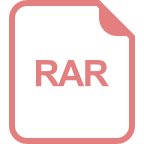
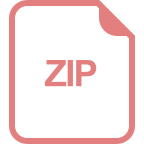
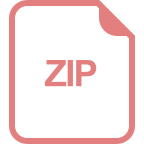