写一个基于c➕➕类加数据库铁路客运之车次管理软件开发 编号 、车次、类型(动车、高铁、普车)、始发站、到达站、耗时、发车时间、经过站数
时间: 2023-09-18 13:11:43 浏览: 149
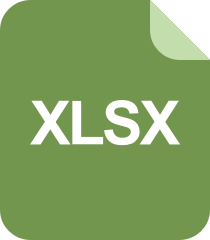
2003-2023年高铁列车信息数据.xlsx

好的,这里是一个简单的基于C++的铁路客运车次管理软件,同时使用SQLite数据库进行数据存储。
```c++
#include <iostream>
#include <string>
#include <vector>
#include "sqlite3.h"
using namespace std;
// 车次信息结构体
struct TrainInfo {
int id; // 编号
string train_no; // 车次
string type; // 类型
string start; // 始发站
string end; // 到达站
int duration; // 耗时
string depart; // 发车时间
int stops; // 经过站数
};
// SQLite数据库操作类
class TrainDB {
public:
TrainDB() {
// 初始化数据库连接
int rc = sqlite3_open("train.db", &db);
if (rc) {
cerr << "无法打开数据库: " << sqlite3_errmsg(db) << endl;
sqlite3_close(db);
exit(1);
}
// 创建数据表
string sql = "CREATE TABLE IF NOT EXISTS train ("
"id INTEGER PRIMARY KEY,"
"train_no TEXT,"
"type TEXT,"
"start TEXT,"
"end TEXT,"
"duration INT,"
"depart TEXT,"
"stops INT);";
rc = sqlite3_exec(db, sql.c_str(), NULL, 0, &zErrMsg);
if (rc != SQLITE_OK) {
cerr << "创建数据表失败: " << zErrMsg << endl;
sqlite3_free(zErrMsg);
sqlite3_close(db);
exit(1);
}
}
~TrainDB() {
sqlite3_close(db);
}
// 插入车次信息
void insertTrain(const TrainInfo& info) {
string sql = "INSERT INTO train (train_no, type, start, end, duration, depart, stops)"
"VALUES ('" + info.train_no + "', '" + info.type + "', '" + info.start + "', '"
+ info.end + "', " + to_string(info.duration) + ", '" + info.depart + "', "
+ to_string(info.stops) + ");";
int rc = sqlite3_exec(db, sql.c_str(), NULL, 0, &zErrMsg);
if (rc != SQLITE_OK) {
cerr << "插入车次信息失败: " << zErrMsg << endl;
sqlite3_free(zErrMsg);
}
}
// 根据车次查询车次信息
vector<TrainInfo> queryTrain(const string& train_no) {
vector<TrainInfo> result;
string sql = "SELECT * FROM train WHERE train_no='" + train_no + "';";
rc = sqlite3_exec(db, sql.c_str(), callback, &result, &zErrMsg);
if (rc != SQLITE_OK) {
cerr << "查询车次信息失败: " << zErrMsg << endl;
sqlite3_free(zErrMsg);
}
return result;
}
// 查询所有车次信息
vector<TrainInfo> queryAllTrains() {
vector<TrainInfo> result;
string sql = "SELECT * FROM train;";
rc = sqlite3_exec(db, sql.c_str(), callback, &result, &zErrMsg);
if (rc != SQLITE_OK) {
cerr << "查询车次信息失败: " << zErrMsg << endl;
sqlite3_free(zErrMsg);
}
return result;
}
private:
sqlite3* db;
char* zErrMsg = 0;
int rc;
// 回调函数
static int callback(void* data, int argc, char** argv, char** azColName) {
vector<TrainInfo>* result = static_cast<vector<TrainInfo>*>(data);
TrainInfo info;
for (int i = 0; i < argc; i++) {
string colName = azColName[i];
if (colName == "id") {
info.id = stoi(argv[i]);
} else if (colName == "train_no") {
info.train_no = argv[i];
} else if (colName == "type") {
info.type = argv[i];
} else if (colName == "start") {
info.start = argv[i];
} else if (colName == "end") {
info.end = argv[i];
} else if (colName == "duration") {
info.duration = stoi(argv[i]);
} else if (colName == "depart") {
info.depart = argv[i];
} else if (colName == "stops") {
info.stops = stoi(argv[i]);
}
}
result->push_back(info);
return 0;
}
};
// 主函数
int main() {
TrainDB db;
string command;
do {
cout << "请输入操作命令: ";
getline(cin, command);
if (command == "add") { // 添加车次信息
TrainInfo info;
cout << "请输入车次编号: ";
cin >> info.id;
cout << "请输入车次号码: ";
cin >> info.train_no;
cout << "请输入车次类型: ";
cin >> info.type;
cout << "请输入始发站: ";
cin >> info.start;
cout << "请输入到达站: ";
cin >> info.end;
cout << "请输入耗时: ";
cin >> info.duration;
cout << "请输入发车时间: ";
cin >> info.depart;
cout << "请输入经过站数: ";
cin >> info.stops;
db.insertTrain(info);
} else if (command == "query") { // 查询车次信息
string train_no;
cout << "请输入要查询的车次号码: ";
cin >> train_no;
vector<TrainInfo> result = db.queryTrain(train_no);
if (result.empty()) {
cout << "未找到车次信息" << endl;
} else {
cout << "查询结果:" << endl;
for (const auto& info : result) {
cout << "车次编号: " << info.id << endl;
cout << "车次号码: " << info.train_no << endl;
cout << "车次类型: " << info.type << endl;
cout << "始发站: " << info.start << endl;
cout << "到达站: " << info.end << endl;
cout << "耗时: " << info.duration << "分钟" << endl;
cout << "发车时间: " << info.depart << endl;
cout << "经过站数: " << info.stops << endl;
}
}
} else if (command == "list") { // 列出所有车次信息
vector<TrainInfo> result = db.queryAllTrains();
if (result.empty()) {
cout << "暂无车次信息" << endl;
} else {
cout << "车次列表:" << endl;
for (const auto& info : result) {
cout << "车次编号: " << info.id << endl;
cout << "车次号码: " << info.train_no << endl;
cout << "车次类型: " << info.type << endl;
cout << "始发站: " << info.start << endl;
cout << "到达站: " << info.end << endl;
cout << "耗时: " << info.duration << "分钟" << endl;
cout << "发车时间: " << info.depart << endl;
cout << "经过站数: " << info.stops << endl;
}
}
} else if (command != "exit") { // 无效命令提示
cout << "无效的命令,请重新输入!" << endl;
}
} while (command != "exit");
return 0;
}
```
这个程序使用了SQLite数据库进行数据存储,可以通过命令行输入添加车次信息、查询车次信息、列出所有车次信息的操作。你可以根据需要进行修改和优化。
阅读全文
相关推荐
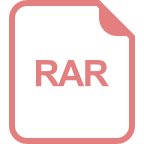
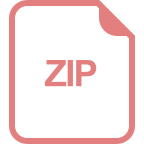
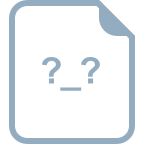
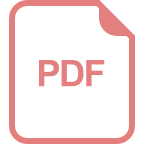
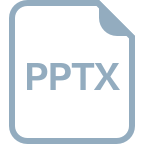
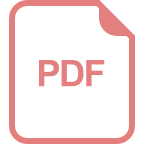
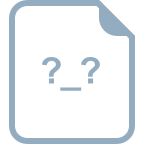
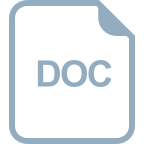
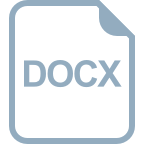
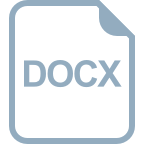
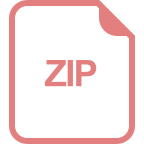
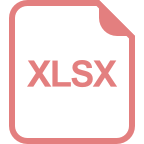
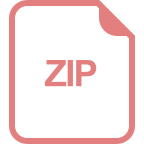
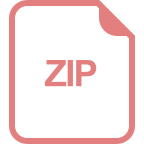
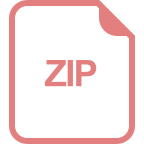
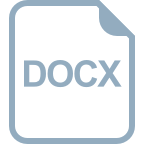