用matlab写randaugment
时间: 2023-07-20 18:08:39 浏览: 170
RandAugment是一种数据增强技术,旨在增强训练集中的多样性,从而提高模型的泛化能力。它使用随机选择的多个数据增强方法来增强每个图像,从而创建一个更大的训练集。下面是MATLAB的实现代码:
```matlab
function augmentedImage = randAugment(originalImage)
% Define the maximum values for magnitude and count of augmentations
MAX_MAGNITUDE = 10;
MAX_COUNT = 3;
% Define the list of augmentations to choose from
augmentations = {
'AutoContrast'
'Brightness'
'Color'
'Contrast'
'Equalize'
'Invert'
'Posterize'
'Sharpness'
'Solarize'
};
% Randomly select the number of augmentations to apply
numAugmentations = randi(MAX_COUNT);
% Randomly select the magnitude for each augmentation
magnitudes = randi(MAX_MAGNITUDE, [numAugmentations, 1]);
% Apply the selected augmentations to the original image
augmentedImage = originalImage;
for i = 1:numAugmentations
% Randomly select an augmentation from the list
augmentation = augmentations{randi(numel(augmentations))};
% Apply the augmentation with the selected magnitude
augmentedImage = augmentImage(augmentedImage, augmentation, magnitudes(i));
end
end
function augmentedImage = augmentImage(originalImage, augmentation, magnitude)
% Apply the specified augmentation to the original image with the specified magnitude
switch augmentation
case 'AutoContrast'
augmentedImage = imadjust(originalImage);
case 'Brightness'
augmentedImage = imadjust(originalImage, [], [], magnitude);
case 'Color'
augmentedImage = imadjust(originalImage, [], [], [], magnitude);
case 'Contrast'
augmentedImage = imadjust(originalImage, [], [], magnitude);
case 'Equalize'
augmentedImage = histeq(originalImage);
case 'Invert'
augmentedImage = imcomplement(originalImage);
case 'Posterize'
augmentedImage = posterize(originalImage, magnitude);
case 'Sharpness'
augmentedImage = imsharpen(originalImage, 'Amount', magnitude);
case 'Solarize'
augmentedImage = solarize(originalImage, magnitude);
otherwise
error('Unknown augmentation: %s', augmentation);
end
end
function posterizedImage = posterize(originalImage, numBits)
% Posterize the image to the specified number of bits per color channel
if numBits > 8
error('Number of bits must be 8 or less');
end
numLevels = 2^numBits;
posterizedImage = uint8(floor(double(originalImage)/(256/numLevels))*(256/numLevels));
end
function solarizedImage = solarize(originalImage, threshold)
% Solarize the image with the specified threshold
if threshold > 255
error('Threshold must be 255 or less');
end
solarizedImage = originalImage;
solarizedImage(originalImage > threshold) = 255 - originalImage(originalImage > threshold);
end
```
这个实现使用了MATLAB的图像处理工具箱函数来实现各种增强方法。您可以根据需要添加、修改或删除增强方法,以创建自己的RandAugment实现。
阅读全文
相关推荐




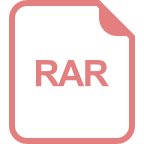


