贪吃蛇的Java代码
时间: 2023-07-02 09:05:40 浏览: 194
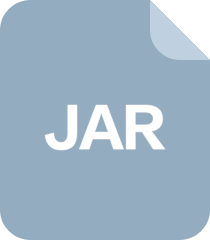
贪吃蛇java代码
以下是一个简单的贪吃蛇Java代码,仅供参考:
```
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.util.*;
public class Snake extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private static final int WIDTH = 500, HEIGHT = 500;
private Timer timer;
private int DELAY = 100;
private int x = 10, y = 10, size = 10;
private int apple_x, apple_y;
private ArrayList<Point> snake;
private int direction = KeyEvent.VK_RIGHT;
public Snake() {
setTitle("Snake");
setSize(WIDTH, HEIGHT);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
setResizable(false);
setVisible(true);
JPanel panel = new JPanel();
getContentPane().add(panel);
panel.setFocusable(true);
panel.addKeyListener(new KeyAdapter() {
public void keyPressed(KeyEvent e) {
int key = e.getKeyCode();
if (key == KeyEvent.VK_LEFT && direction != KeyEvent.VK_RIGHT) {
direction = KeyEvent.VK_LEFT;
} else if (key == KeyEvent.VK_RIGHT && direction != KeyEvent.VK_LEFT) {
direction = KeyEvent.VK_RIGHT;
} else if (key == KeyEvent.VK_UP && direction != KeyEvent.VK_DOWN) {
direction = KeyEvent.VK_UP;
} else if (key == KeyEvent.VK_DOWN && direction != KeyEvent.VK_UP) {
direction = KeyEvent.VK_DOWN;
}
}
});
timer = new Timer(DELAY, this);
startGame();
}
private void startGame() {
snake = new ArrayList<Point>();
snake.add(new Point(x, y));
generateApple();
timer.start();
}
private void generateApple() {
apple_x = (int) (Math.random() * (WIDTH / size)) * size;
apple_y = (int) (Math.random() * (HEIGHT / size)) * size;
}
private void move() {
Point head = snake.get(0);
switch (direction) {
case KeyEvent.VK_LEFT:
x -= size;
break;
case KeyEvent.VK_RIGHT:
x += size;
break;
case KeyEvent.VK_UP:
y -= size;
break;
case KeyEvent.VK_DOWN:
y += size;
break;
}
head.setLocation(x, y);
checkCollision();
if (x == apple_x && y == apple_y) {
snake.add(new Point(x, y));
generateApple();
} else {
snake.remove(snake.size() - 1);
}
}
private void checkCollision() {
if (x < 0 || x >= WIDTH || y < 0 || y >= HEIGHT) {
gameOver();
}
for (int i = 1; i < snake.size(); i++) {
if (x == snake.get(i).getX() && y == snake.get(i).getY()) {
gameOver();
}
}
}
private void gameOver() {
timer.stop();
JOptionPane.showMessageDialog(this, "Game Over", "Game Over", JOptionPane.YES_NO_OPTION);
startGame();
}
public void actionPerformed(ActionEvent e) {
move();
repaint();
}
public void paint(Graphics g) {
super.paint(g);
g.setColor(Color.RED);
g.fillRect(apple_x, apple_y, size, size);
g.setColor(Color.BLACK);
for (Point p : snake) {
g.fillRect((int) p.getX(), (int) p.getY(), size, size);
}
}
public static void main(String[] args) {
new Snake();
}
}
```
这个代码实现了一个简单的贪吃蛇游戏,玩家可以通过方向键控制蛇的移动方向,吃到苹果后蛇会变长,如果蛇触碰到边界或自己的身体则游戏结束。
阅读全文
相关推荐
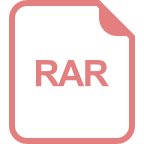
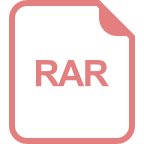
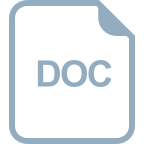
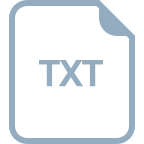




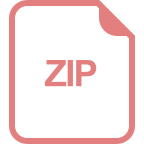
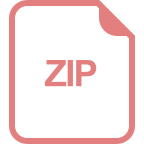
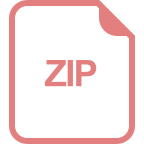
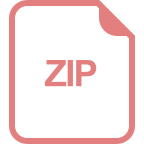