Android studio实现一个界面,要求需要有消息列表框架、底部菜单栏。给出xml和java代码
时间: 2024-02-24 17:00:20 浏览: 136
好的,以下是一个基本的消息列表框架和底部菜单栏的XML和Java代码示例:
activity_main.xml:
```
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/message_list"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_above="@+id/bottom_navigation"/>
<com.google.android.material.bottomnavigation.BottomNavigationView
android:id="@+id/bottom_navigation"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:background="?android:attr/windowBackground"
android:menu="@menu/bottom_navigation_menu" />
</RelativeLayout>
```
bottom_navigation_menu.xml:
```
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:id="@+id/navigation_home"
android:icon="@drawable/ic_home"
android:title="@string/title_home" />
<item
android:id="@+id/navigation_messages"
android:icon="@drawable/ic_messages"
android:title="@string/title_messages" />
<item
android:id="@+id/navigation_notifications"
android:icon="@drawable/ic_notifications"
android:title="@string/title_notifications" />
<item
android:id="@+id/navigation_profile"
android:icon="@drawable/ic_profile"
android:title="@string/title_profile" />
</menu>
```
MainActivity.java:
```
import androidx.appcompat.app.AppCompatActivity;
import androidx.recyclerview.widget.LinearLayoutManager;
import androidx.recyclerview.widget.RecyclerView;
import android.os.Bundle;
import com.google.android.material.bottomnavigation.BottomNavigationView;
public class MainActivity extends AppCompatActivity {
private RecyclerView messageList;
private BottomNavigationView bottomNavigation;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Initialize RecyclerView and Adapter
messageList = findViewById(R.id.message_list);
messageList.setLayoutManager(new LinearLayoutManager(this));
MessageAdapter adapter = new MessageAdapter();
messageList.setAdapter(adapter);
// Initialize BottomNavigationView and set listener
bottomNavigation = findViewById(R.id.bottom_navigation);
bottomNavigation.setOnNavigationItemSelectedListener(item -> {
switch (item.getItemId()) {
case R.id.navigation_home:
// Handle Home button click
return true;
case R.id.navigation_messages:
// Handle Messages button click
return true;
case R.id.navigation_notifications:
// Handle Notifications button click
return true;
case R.id.navigation_profile:
// Handle Profile button click
return true;
}
return false;
});
}
}
```
MessageAdapter.java:
```
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
import androidx.annotation.NonNull;
import androidx.recyclerview.widget.RecyclerView;
import java.util.ArrayList;
public class MessageAdapter extends RecyclerView.Adapter<MessageAdapter.ViewHolder> {
private ArrayList<String> messages;
public MessageAdapter() {
messages = new ArrayList<>();
messages.add("Hello");
messages.add("How are you?");
messages.add("I'm fine, thank you.");
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.message_item, parent, false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull ViewHolder holder, int position) {
String message = messages.get(position);
holder.messageText.setText(message);
}
@Override
public int getItemCount() {
return messages.size();
}
public static class ViewHolder extends RecyclerView.ViewHolder {
private TextView messageText;
public ViewHolder(@NonNull View itemView) {
super(itemView);
messageText = itemView.findViewById(R.id.message_text);
}
}
}
```
message_item.xml:
```
<?xml version="1.0" encoding="utf-8"?>
<TextView xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/message_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="16dp"
android:textSize="16sp" />
```
注意:这只是一个基本的示例,您需要根据自己的需求进行修改和优化。
阅读全文
相关推荐
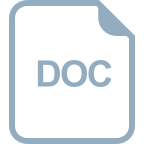
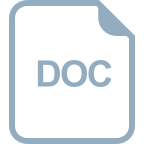
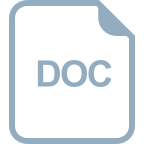
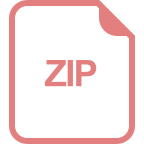
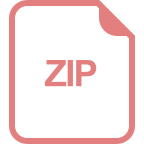
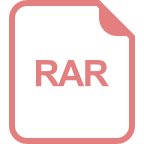
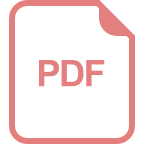
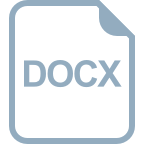
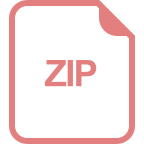
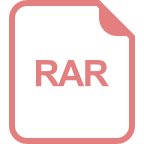
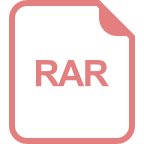
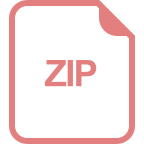
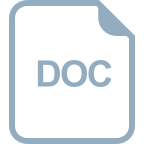
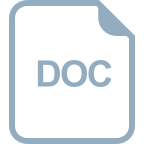
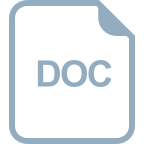
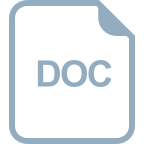
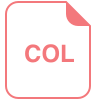
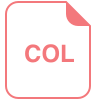
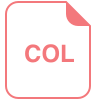