在stm32中用串口代码写modbus协议
时间: 2023-09-12 08:10:18 浏览: 67
下面是一个简单的示例代码,用于在STM32中使用串口实现Modbus协议:
```c
#include "stm32f10x.h"
#include "modbus.h"
#define MODBUS_SLAVE_ADDRESS 0x01
// 串口发送字节
void USART_SendByte(uint8_t byte) {
while (!(USART1->SR & USART_SR_TXE));
USART1->DR = byte;
}
// 串口接收字节
uint8_t USART_ReceiveByte() {
while (!(USART1->SR & USART_SR_RXNE));
return USART1->DR;
}
// 初始化串口
void USART_Init() {
// 配置GPIO
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置串口
USART_InitTypeDef USART_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
}
int main() {
// 初始化串口
USART_Init();
// 初始化Modbus
modbus_init(MODBUS_SLAVE_ADDRESS);
// 循环处理Modbus请求
while (1) {
// 接收请求
uint8_t request[MODBUS_MAX_MESSAGE_LENGTH];
uint8_t request_length = 0;
while (1) {
uint8_t byte = USART_ReceiveByte();
request[request_length++] = byte;
if (request_length >= MODBUS_MAX_MESSAGE_LENGTH) {
break;
}
if (byte == '\n') {
break;
}
}
// 处理请求
uint8_t response[MODBUS_MAX_MESSAGE_LENGTH];
uint8_t response_length = modbus_process(request, request_length, response);
// 发送响应
for (int i = 0; i < response_length; i++) {
USART_SendByte(response[i]);
}
}
}
```
在上述示例代码中,我们通过 `USART_SendByte` 和 `USART_ReceiveByte` 函数来实现通过串口发送和接收字节的功能。我们还通过 `modbus_init` 函数来初始化Modbus协议,然后在一个无限循环中不断接收Modbus请求,处理请求并发送响应。需要注意的是,此处仅为示例代码,具体的Modbus处理函数需要根据自己的需求进行编写。
相关推荐
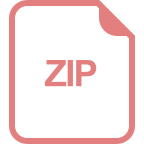
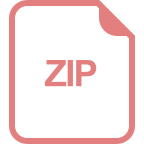
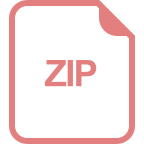
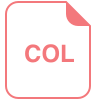
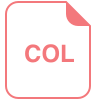
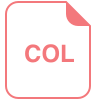
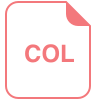
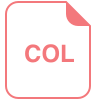









