LambdaQueryWrapper<TopicResult> topicResultLambdaQueryWrapper = new LambdaQueryWrapper<>();请详细解释
时间: 2023-07-19 18:27:13 浏览: 37
这是一个 Java 代码段,使用了 MyBatis-Plus 框架中的 LambdaQueryWrapper 类来构建查询条件,查询的表名为 TopicResult。
LambdaQueryWrapper 是一个 Lambda 表达式查询构造器,可以方便地通过 Lambda 表达式构建查询条件,避免了手写 SQL 语句的繁琐和易错。通过 LambdaQueryWrapper 可以实现多种查询条件的组合,包括等值、模糊匹配、范围查询、排序等。
在上述代码中,LambdaQueryWrapper 的泛型参数为 TopicResult,表示查询的表名为 TopicResult,LambdaQueryWrapper 的实例化采用了无参构造函数。之后可以通过 LambdaQueryWrapper 的各种方法来添加查询条件,例如:
- eq:等于
- like:模糊匹配
- between:范围查询
- orderByAsc:升序排序
- orderByDesc:降序排序
最终通过 MyBatis-Plus 提供的方法来执行查询,例如:
- selectList:查询多条记录
- selectOne:查询一条记录
- selectCount:查询记录数
这些方法会返回一个查询结果集,可以通过 Java 对象进行封装和处理。
需要注意的是,该代码段中的 TopicResult 类需要有对应的属性和 getter 方法。同时,该代码段中的 LambdaQueryWrapper 的泛型参数需要和对应的实体类一致。
相关问题
LambdaQueryWrapper<User> wrapper = new LambdaQueryWrapper<>()
`LambdaQueryWrapper<User> wrapper = new LambdaQueryWrapper<>();` 是一个使用 MyBatis-Plus 库中的 LambdaQueryWrapper 类创建一个查询条件的示例代码。
`LambdaQueryWrapper` 是一个用于构建数据库查询条件的实用工具类。它基于 Lambda 表达式,使得查询条件的构建更加简洁和易于阅读。
在这个示例中,`User` 是一个实体类,代表数据库中的用户表。通过创建 `LambdaQueryWrapper<User>` 对象,你可以使用链式调用的方式来构建查询条件,例如:
```java
wrapper.eq(User::getName, "John")
.ge(User::getAge, 18)
.orderByAsc(User::getCreateTime);
```
上述代码的作用是构建一个查询条件,要求查询名为 "John" 的用户,年龄大于等于 18 岁,并按照创建时间升序排序。
完成查询条件的构建后,你可以将 `wrapper` 对象传递给 MyBatis-Plus 提供的查询方法,例如 `userMapper.selectList(wrapper)`,来执行数据库查询操作。
请注意,这只是一个示例代码,具体的使用方法和功能可能需要根据你的实际业务需求进行调整。
LambdaQueryWrapper<JiaoKaoExaminePoolRegisterRecord> wrapper = new LambdaQueryWrapper<>();
LambdaQueryWrapper是MyBatis-Plus框架提供的一个查询条件构造器,用于构建数据库查询条件。在这个例子中,我们创建了一个LambdaQueryWrapper对象,并指定了泛型类型为JiaoKaoExaminePoolRegisterRecord。
使用LambdaQueryWrapper可以方便地构建查询条件,例如添加等值条件、模糊查询、排序等。通过链式调用方法,可以根据需要添加多个查询条件。
下面是一些常用的LambdaQueryWrapper方法:
1. eq:等值条件
2. like:模糊查询
3. orderByAsc:升序排序
4. orderByDesc:降序排序
5. select:指定查询的字段
6. last:在SQL语句的最后添加自定义内容
示例代码如下:
```
LambdaQueryWrapper<JiaoKaoExaminePoolRegisterRecord> wrapper = new LambdaQueryWrapper<>();
wrapper.eq(JiaoKaoExaminePoolRegisterRecord::getId, 1)
.like(JiaoKaoExaminePoolRegisterRecord::getName, "张三")
.orderByAsc(JiaoKaoExaminePoolRegisterRecord::getCreateTime)
.select(JiaoKaoExaminePoolRegisterRecord::getId, JiaoKaoExaminePoolRegisterRecord::getName)
.last("limit 10");
```
这段代码创建了一个LambdaQueryWrapper对象,并添加了等值条件、模糊查询、排序、指定查询字段和自定义SQL语句。
相关推荐
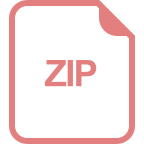
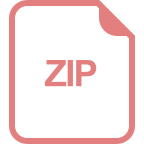












