用python+txt编写随机抽题出卷程序
时间: 2023-09-23 14:00:39 浏览: 186
使用Python编写随机抽题出卷程序是可行的。下面是一个简单的实现示例:
首先,我们需要将题库保存在一个txt文件中。每道题包含题目和答案,可以按照一定的格式进行保存,比如每道题之间使用分隔符分开。
接下来,我们需要读取题库,将题目和答案分别保存在两个列表中。
然后,我们可以使用random模块中的函数来实现随机抽题。比如可以生成一个指定数量的随机数,作为题目的索引,然后将对应的题目和答案保存在新的列表中。
最后,我们可以将随机抽取的题目和答案输出到一个新的txt文件中,作为考试试卷。
下面是一个简单的示例代码:
```python
import random
# 题库文件路径
question_file = "question.txt"
# 输出试卷文件路径
exam_file = "exam.txt"
# 随机抽取的题目数量
num_questions = 5
# 读取题库,将题目和答案保存在两个列表中
questions = []
answers = []
with open(question_file, "r", encoding="utf-8") as file:
for line in file:
question, answer = line.strip().split(";")
questions.append(question)
answers.append(answer)
# 随机抽取题目
random_questions = []
for _ in range(num_questions):
index = random.randint(0, len(questions)-1)
random_questions.append((questions[index], answers[index]))
# 输出试卷
with open(exam_file, "w", encoding="utf-8") as file:
for i, (question, answer) in enumerate(random_questions, 1):
file.write("Question {}:\n".format(i))
file.write(question + "\n")
file.write("Answer: {}\n".format(answer))
file.write("\n")
```
这样,程序就可以从题库中随机抽题,并将抽取的题目和答案保存在exam.txt文件中。
阅读全文
相关推荐
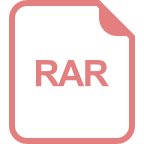
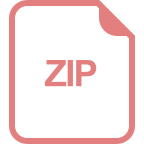
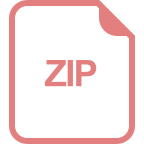

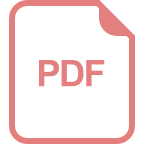
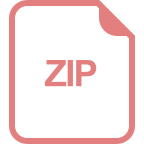
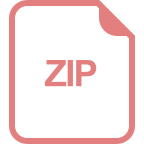
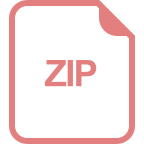
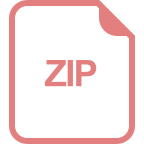
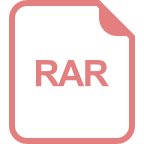
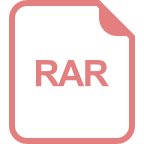





