哈夫曼编码和译码代码
时间: 2024-05-06 18:14:24 浏览: 110
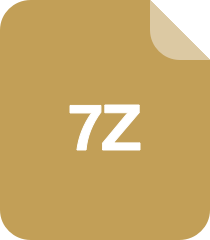
哈夫曼编码和译码
哈夫曼编码是一种基于字符出现频率进行编码的算法,其主要思想是将出现频率高的字符用较短的编码表示,而出现频率低的字符则用较长的编码表示。这样可以有效地减少编码长度,从而达到压缩数据的目的。下面是一个简单的哈夫曼编码和译码的代码实现:
```c++
#include <iostream>
#include <queue>
#include <unordered_map>
using namespace std;
// 哈夫曼树节点
struct Node {
char ch; // 字符
int freq; // 频率
Node* left;
Node* right;
Node(char c, int f) : ch(c), freq(f), left(nullptr), right(nullptr) {}
};
// 比较函数
struct cmp {
bool operator() (Node* a, Node* b) {
return a->freq > b->freq;
}
};
// 构建哈夫曼树
Node* buildHuffmanTree(const string& s) {
unordered_map<char, int> freqMap;
for (char c : s) {
freqMap[c]++;
}
priority_queue<Node*, vector<Node*>, cmp> pq;
for (auto it = freqMap.begin(); it != freqMap.end(); it++) {
pq.push(new Node(it->first, it->second));
}
while (pq.size() > 1) {
Node* left = pq.top(); pq.pop();
Node* right = pq.top(); pq.pop();
Node* parent = new Node('$', left->freq + right->freq);
parent->left = left;
parent->right = right;
pq.push(parent);
}
return pq.top();
}
// 生成哈夫曼编码表
void generateHuffmanCodes(Node* root, string code, unordered_map<char, string>& codes) {
if (!root) return;
if (root->ch != '$') {
codes[root->ch] = code;
}
generateHuffmanCodes(root->left, code + "0", codes);
generateHuffmanCodes(root->right, code + "1", codes);
}
// 哈夫曼编码
string encode(const string& s) {
Node* root = buildHuffmanTree(s);
unordered_map<char, string> codes;
generateHuffmanCodes(root, "", codes);
string res = "";
for (char c : s) {
res += codes[c];
}
return res;
}
// 哈夫曼译码
string decode(Node* root, const string& code) {
string res = "";
Node* cur = root;
for (char c : code) {
if (c == '0') {
cur = cur->left;
} else {
cur = cur->right;
}
if (cur->ch != '$') {
res += cur->ch;
cur = root;
}
}
return res;
}
int main() {
string s = "hello world";
string encoded = encode(s);
cout << "Encoded: " << encoded << endl;
Node* root = buildHuffmanTree(s);
string decoded = decode(root, encoded);
cout << "Decoded: " << decoded << endl;
return 0;
}
```
阅读全文
相关推荐
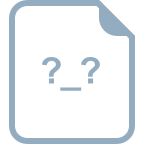
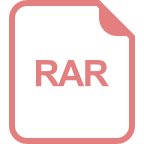



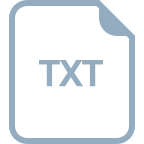
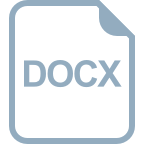
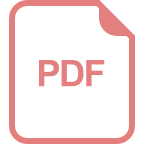







