用c写一个图书管理系统
时间: 2024-05-13 16:16:53 浏览: 51
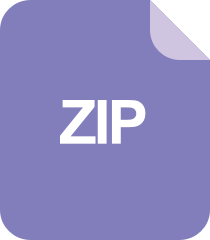
基于链表的图书管理系统项目
好的,下面是一个简单的图书管理系统的C代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_BOOKS 100 // 最大图书数量
typedef struct {
char title[50]; // 书名
char author[20]; // 作者
int year; // 出版年份
int copies; // 剩余复本数
} Book;
Book books[MAX_BOOKS]; // 图书数组
int num_books = 0; // 当前图书数量
void add_book() {
if (num_books >= MAX_BOOKS) {
printf("Error: too many books.\n");
return;
}
Book book;
printf("Enter book title: ");
scanf("%s", book.title);
printf("Enter author name: ");
scanf("%s", book.author);
printf("Enter publication year: ");
scanf("%d", &book.year);
printf("Enter number of copies: ");
scanf("%d", &book.copies);
books[num_books++] = book;
printf("Book added successfully.\n");
}
void search_book() {
char title[50];
printf("Enter book title: ");
scanf("%s", title);
for (int i = 0; i < num_books; i++) {
if (strcmp(title, books[i].title) == 0) {
printf("Title: %s\n", books[i].title);
printf("Author: %s\n", books[i].author);
printf("Year: %d\n", books[i].year);
printf("Copies: %d\n", books[i].copies);
return;
}
}
printf("Book not found.\n");
}
void display_books() {
printf("Title\tAuthor\tYear\tCopies\n");
for (int i = 0; i < num_books; i++) {
printf("%s\t%s\t%d\t%d\n", books[i].title, books[i].author, books[i].year, books[i].copies);
}
}
int main() {
int choice;
while (1) {
printf("1. Add book\n");
printf("2. Search book\n");
printf("3. Display all books\n");
printf("4. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_book();
break;
case 2:
search_book();
break;
case 3:
display_books();
break;
case 4:
exit(0);
default:
printf("Invalid choice.\n");
}
printf("\n");
}
return 0;
}
```
这个图书管理系统包括以下功能:
- 添加图书
- 搜索图书
- 显示所有图书
- 退出程序
你可以根据自己的需求进行修改和完善。
阅读全文
相关推荐
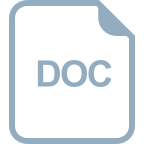
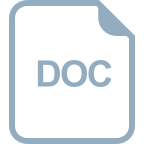


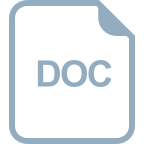
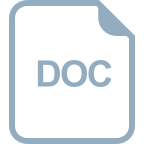
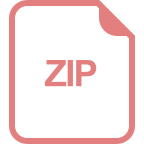
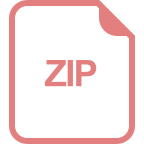
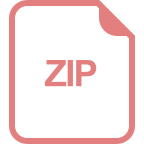
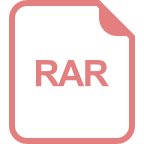
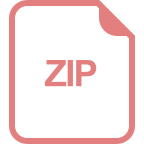
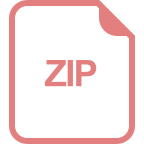
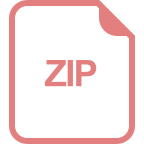
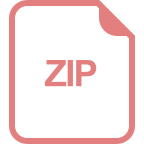
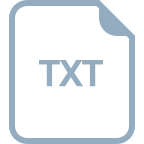
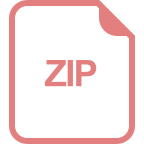
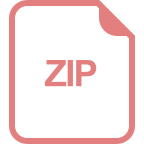
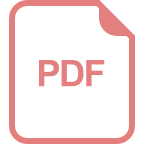