请个我写一个.vue和苹果手机一样的备忘录代码
时间: 2023-09-13 07:05:23 浏览: 56
### 回答1:
这里提供一个简单的备忘录的Vue代码示例,你可以根据需要进行修改和完善。
```html
<template>
<div class="memo">
<h2>备忘录</h2>
<div class="input-container">
<input type="text" placeholder="添加新的备忘录" v-model="newMemo" @keyup.enter="addMemo">
<button @click="addMemo">添加</button>
</div>
<ul class="memo-list">
<li v-for="(memo, index) in memoList" :key="index">
<span class="memo-text">{{ memo }}</span>
<button class="delete-btn" @click="deleteMemo(index)">删除</button>
</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
newMemo: '',
memoList: []
}
},
methods: {
addMemo() {
if (this.newMemo.trim() !== '') {
this.memoList.push(this.newMemo.trim())
this.newMemo = ''
}
},
deleteMemo(index) {
this.memoList.splice(index, 1)
}
}
}
</script>
<style scoped>
.memo {
max-width: 600px;
margin: 0 auto;
padding: 20px;
font-family: Arial, sans-serif;
text-align: center;
}
.memo h2 {
font-size: 24px;
font-weight: bold;
margin-bottom: 20px;
}
.input-container {
display: flex;
align-items: center;
justify-content: center;
margin-bottom: 20px;
}
.input-container input[type=text] {
width: 70%;
padding: 10px;
border: none;
border-radius: 4px;
font-size: 16px;
margin-right: 10px;
}
.input-container button {
background-color: #4CAF50;
color: white;
border: none;
border-radius: 4px;
cursor: pointer;
padding: 10px;
font-size: 16px;
}
.memo-list {
list-style: none;
padding: 0;
margin: 0;
}
.memo-list li {
display: flex;
justify-content: space-between;
align-items: center;
padding: 10px;
border-bottom: 1px solid #ddd;
}
.memo-list li:last-child {
border-bottom: none;
}
.memo-list li .memo-text {
flex: 1;
text-align: left;
}
.memo-list li .delete-btn {
background-color: #f44336;
color: white;
border: none;
border-radius: 4px;
cursor: pointer;
padding: 10px;
font-size: 16px;
}
</style>
```
这个备忘录的界面和苹果手机的备忘录类似,你可以在苹果手机上参考备忘录的布局和样式设计。
### 回答2:
备忘录是一种记录事物、提醒事项的工具,我们可以使用Vue框架来构建一个类似于苹果手机备忘录的应用。下面是一个简单的备忘录代码示例:
```
<template>
<div>
<h2>备忘录</h2>
<input type="text" v-model="newNote" placeholder="输入新的备忘录">
<button @click="addNote">添加</button>
<ul>
<li v-for="(note, index) in notes" :key="index">
{{ note }}
<button @click="deleteNote(index)">删除</button>
</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
newNote: '',
notes: []
};
},
methods: {
addNote() {
if (this.newNote) {
this.notes.push(this.newNote);
this.newNote = '';
}
},
deleteNote(index) {
this.notes.splice(index, 1);
}
}
};
</script>
<style scoped>
h2 {
text-align: center;
}
input {
margin-bottom: 10px;
}
ul {
list-style-type: none;
padding: 0;
}
li {
display: flex;
justify-content: space-between;
margin-bottom: 5px;
}
button {
background-color: #f5f5f5;
border: none;
padding: 5px 10px;
cursor: pointer;
}
</style>
```
这个代码通过Vue的数据绑定和列表渲染功能实现了一个备忘录应用。用户可以在输入框中输入备忘录内容,点击添加按钮后,备忘录会显示在备忘录列表中。用户可以点击每个备忘录后面的删除按钮来删除对应的备忘录。整个界面使用简洁的样式,类似于苹果手机备忘录的风格。
### 回答3:
当然可以!下面是一个简单的基于Vue.js的备忘录代码示例,它可以实现类似苹果手机备忘录的功能:
```html
<template>
<div class="memo-app">
<h1>备忘录</h1>
<div class="memo-list">
<div v-for="memo in memos" :key="memo.id" class="memo-item">
<p>{{ memo.text }}</p>
<button @click="deleteMemo(memo.id)">删除</button>
</div>
</div>
<div class="memo-form">
<input type="text" v-model="newMemo" placeholder="输入备忘录内容" />
<button @click="addMemo">添加</button>
</div>
</div>
</template>
<script>
export default {
data() {
return {
memos: [], // 备忘录列表
newMemo: "", // 新备忘录内容
memoId: 0, // 备忘录ID
};
},
methods: {
addMemo() {
if (this.newMemo.trim() !== "") {
this.memos.push({
id: this.memoId++,
text: this.newMemo,
});
this.newMemo = "";
}
},
deleteMemo(id) {
this.memos = this.memos.filter((memo) => memo.id !== id);
},
},
};
</script>
<style scoped>
.memo-app {
max-width: 500px;
margin: 0 auto;
}
.memo-list {
margin-top: 20px;
}
.memo-item {
display: flex;
justify-content: space-between;
align-items: center;
padding: 10px;
border: 1px solid #ddd;
margin-bottom: 10px;
}
.memo-form {
display: flex;
margin-top: 20px;
}
.memo-form input {
flex-grow: 1;
margin-right: 10px;
}
.memo-form button {
background-color: #007bff;
color: #fff;
border: none;
padding: 8px 12px;
cursor: pointer;
}
</style>
```
这个代码中,我们使用Vue.js的双向绑定和列表渲染功能来实现备忘录的展示和添加、删除功能。当用户输入备忘录内容后点击添加按钮,新备忘录会添加到备忘录列表中;点击备忘录条目的删除按钮,对应备忘录会从列表中删除。这样,用户就可以像使用苹果手机备忘录一样在页面中管理自己的备忘录。
相关推荐
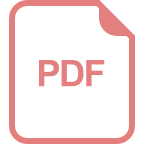
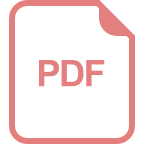














