date转localdatetime
时间: 2023-09-03 17:04:43 浏览: 107
### 回答1:
date 对象可以使用 java.time 包中的 LocalDateTime.ofInstant() 方法来转换为 LocalDateTime 对象。
例如:
```
import java.time.Instant;
import java.time.LocalDateTime;
import java.time.ZoneId;
import java.util.Date;
public class Main {
public static void main(String[] args) {
Date date = new Date();
Instant instant = date.toInstant();
ZoneId zoneId = ZoneId.systemDefault();
LocalDateTime localDateTime = LocalDateTime.ofInstant(instant, zoneId);
System.out.println(localDateTime);
}
}
```
在上面的代码中,我们首先将 Date 对象转换为 Instant 对象,然后使用 LocalDateTime.ofInstant() 方法将 Instant 对象转换为 LocalDateTime 对象。
### 回答2:
在Java中,我们可以使用`java.util.Date`和`java.time.LocalDateTime`来进行日期的转换。
要将`Date`对象转换为`LocalDateTime`对象,我们可以使用`Instant`来进行中间转换。首先,使用`getTime()`方法从`Date`对象中获取毫秒数,然后使用`Instant.ofEpochMilli()`方法将毫秒数转换为`Instant`对象。最后,使用`atZone()`方法将`Instant`对象以默认时区转换为`ZonedDateTime`对象,并使用`toLocalDateTime()`方法将其转换为`LocalDateTime`对象。
以下是将`Date`对象转换为`LocalDateTime`对象的示例代码:
```java
import java.util.Date;
import java.time.LocalDateTime;
import java.time.Instant;
import java.time.ZoneId;
import java.time.ZonedDateTime;
public class DateToLocalDateTimeExample {
public static void main(String[] args) {
Date date = new Date();
Instant instant = date.toInstant();
ZonedDateTime zonedDateTime = instant.atZone(ZoneId.systemDefault());
LocalDateTime localDateTime = zonedDateTime.toLocalDateTime();
System.out.println("Date: " + date);
System.out.println("LocalDateTime: " + localDateTime);
}
}
```
以上代码会将当前日期转换为`LocalDateTime`对象,并将其打印输出。
需要注意的是,`Date`类是不可变类,而`LocalDateTime`类是可变类。因此,如果需要更改日期的话,可以通过以上示例中的`LocalDateTime`对象来进行修改。
### 回答3:
要将Date对象转换为LocalDateTime对象,可以按照下面的步骤进行操作。
首先,需要将Date对象转换为Instant对象。可以使用toInstant()方法将Date对象转换为Instant对象。
然后,可以使用Instant对象的atZone()方法将其转换为ZonedDateTime对象。atZone()方法接受一个时区偏移作为参数,可以使用系统默认的时区偏移。
接下来,可以使用ZonedDateTime对象的toLocalDateTime()方法将其转换为LocalDateTime对象,该方法会去除时区信息,只保留日期时间信息。
下面是一个示例代码:
```
import java.util.Date;
import java.time.Instant;
import java.time.LocalDateTime;
import java.time.ZoneId;
import java.time.ZonedDateTime;
public class DateToLocalDateTimeExample {
public static void main(String[] args) {
Date date = new Date();
Instant instant = date.toInstant();
ZonedDateTime zonedDateTime = instant.atZone(ZoneId.systemDefault());
LocalDateTime localDateTime = zonedDateTime.toLocalDateTime();
System.out.println("LocalDateTime: " + localDateTime);
}
}
```
在上面的示例代码中,创建了一个Date对象date。然后,使用toInstant()方法将其转换为Instant对象instant。接着,使用atZone()方法将instant对象转换为ZonedDateTime对象zonedDateTime,传入系统默认的时区偏移作为参数。最后,使用toLocalDateTime()方法将zonedDateTime对象转换为LocalDateTime对象localDateTime。通过System.out.println()方法打印localDateTime对象的值。
运行上述代码,即可将Date对象成功转换为LocalDateTime对象。
阅读全文
相关推荐
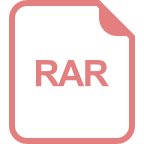











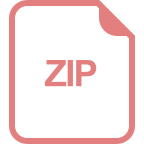
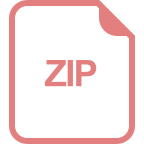