pandas转dict
时间: 2023-09-11 08:04:26 浏览: 30
可以使用`to_dict()`方法将pandas DataFrame转换为字典。
示例代码:
```python
import pandas as pd
# 创建一个DataFrame
data = {'name': ['Alice', 'Bob', 'Charlie'],
'age': [25, 30, 35],
'gender': ['F', 'M', 'M']}
df = pd.DataFrame(data)
# 将DataFrame转换为字典
d = df.to_dict(orient='records')
print(d)
```
输出结果:
```
[{'name': 'Alice', 'age': 25, 'gender': 'F'},
{'name': 'Bob', 'age': 30, 'gender': 'M'},
{'name': 'Charlie', 'age': 35, 'gender': 'M'}]
```
将`orient`参数设置为`'records'`可以将DataFrame转换为一个由字典组成的列表,每个字典表示一行数据。还可以设置为`'dict'`,将DataFrame转换为一个字典,每个键表示列名,每个值表示该列的数据。
相关问题
dataframe转dict
要将一个DataFrame转换为字典,你可以使用`to_dict()`方法。这个方法有几个参数,可以根据需要进行设置。下面是一个示例代码:
```python
import pandas as pd
# 创建一个示例DataFrame
data = {'Name': ['John', 'Emma', 'Mike'],
'Age': [25, 28, 30],
'City': ['New York', 'London', 'Paris']}
df = pd.DataFrame(data)
# 将DataFrame转换为字典
dictionary = df.to_dict()
print(dictionary)
```
这将输出以下结果:
```
{'Name': {0: 'John', 1: 'Emma', 2: 'Mike'}, 'Age': {0: 25, 1: 28, 2: 30}, 'City': {0: 'New York', 1: 'London', 2: 'Paris'}}
```
默认情况下,`to_dict()`方法将列名作为字典的键,并将每列的值转换为字典的值。如果你想要以其他方式进行转换,请参考`to_dict()`方法的参数文档。
pandas 两列转字典
可以使用pandas中的to_dict方法将两列数据转换为字典。假设有如下的两列数据:
```python
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3, 4, 5],
'B': ['a', 'b', 'c', 'd', 'e']})
```
可以使用下列代码将这两列数据转换为字典:
```python
result = df.set_index('A')['B'].to_dict()
print(result)
```
执行上述代码后,将会得到如下结果:
```
{1: 'a', 2: 'b', 3: 'c', 4: 'd', 5: 'e'}
```
这样就将DataFrame中的两列数据A和B转换成了一个字典,其中A列的值作为字典的键,而B列的值作为字典的值。这种方法在处理数据时非常方便,可以帮助我们快速将DataFrame中的两列数据转换为字典,以便后续的操作和分析。
相关推荐
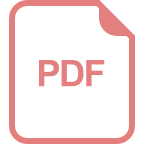
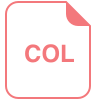











