用c语言创建一个存放了{1,2,3}3个元素的带头结点的单链表L2
时间: 2023-06-01 20:04:36 浏览: 111
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct ListNode {
int val;
struct ListNode* next;
} ListNode;
void insert(ListNode* head, int val) {
ListNode* node = (ListNode*)malloc(sizeof(ListNode));
node->val = val;
node->next = head->next;
head->next = node;
}
void printList(ListNode* head) {
ListNode* p = head->next;
while(p != NULL) {
printf("%d ", p->val);
p = p->next;
}
printf("\n");
}
int main() {
ListNode* head = (ListNode*)malloc(sizeof(ListNode));
head->next = NULL;
insert(head, 3);
insert(head, 2);
insert(head, 1);
printList(head);
return 0;
}
```
相关问题
用C语言写一个带头结点单链表
C语言写一个带头结点的单链表,需要先定义一个结构体来存储每一个结点的数据。一般的结构体定义如下:
```
struct Node {
int data;
struct Node *next;
};
```
然后,需要定义一个指针变量来指向链表的头结点:
```
struct Node *head = NULL;
```
最后,可以实现一些基本的操作,如插入、删除、查询等。例如,插入操作如下:
```
void insert(int value) {
struct Node *new_node = (struct Node*)malloc(sizeof(struct Node));
new_node->data = value;
new_node->next = head;
head = new_node;
}
```
带头结点的单链表有很多应用,在实际开发中会经常用到。带头结点的单链表比不带头结点的链表更加方便和实用,同时也更加容易实现。
请用C语言创建一个不带头结点的单链表
好的,以下是创建一个不带头结点的单链表的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node *next;
};
int main() {
struct Node *head = NULL, *tail = NULL, *temp = NULL;
int num;
printf("请输入整数,输入 -1 结束:\n");
scanf("%d", &num);
while (num != -1) {
temp = (struct Node *) malloc(sizeof(struct Node));
temp -> data = num;
temp -> next = NULL;
if (head == NULL) {
head = temp;
tail = temp;
} else {
tail -> next = temp;
tail = temp;
}
scanf("%d", &num);
}
printf("链表的值为:\n");
temp = head;
while (temp != NULL) {
printf("%d ", temp -> data);
temp = temp -> next;
}
return 0;
}
```
希望这能够帮到您!
阅读全文
相关推荐
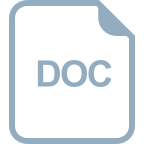
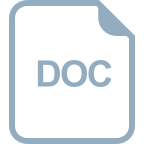











