用C语言写一个带头结点的循环单链表
时间: 2023-02-22 22:03:39 浏览: 96
以下是用C语言实现带头结点的循环单链表的代码示例:
```
#include <stdio.h>
#include <stdlib.h>
struct node {
int data;
struct node *next;
};
struct node *create(int data) {
struct node *new_node = (struct node *)malloc(sizeof(struct node));
new_node->data = data;
new_node->next = NULL;
return new_node;
}
void insert(struct node *head, int data) {
struct node *new_node = create(data);
struct node *temp = head->next;
head->next = new_node;
new_node->next = temp;
}
void print_list(struct node *head) {
struct node *temp = head->next;
while (temp != head) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
int main() {
struct node *head = create(0);
head->next = head;
insert(head, 1);
insert(head, 2);
insert(head, 3);
print_list(head);
return 0;
}
```
该代码实现了一个带头结点的循环单链表,它包含了以下几个函数:
1. `create`:创建一个新结点。
2. `insert`:在链表头插入一个新结点。
3. `print_list`:遍历链表并打印元素。
需要注意的是,在本代码中,链表是循环的,即最后一个结点的 `next` 指针指向链表头。
相关推荐
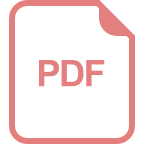
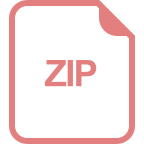
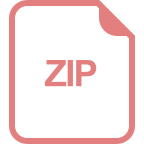
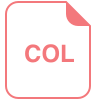
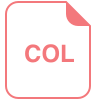
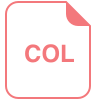
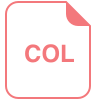
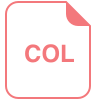









