排课程序c++
时间: 2023-11-22 07:53:21 浏览: 79
排课程序需要具体的算法设计和数据结构,以下是一个简单的排课程序的思路:
1. 设定需要排课的时间段和教室数量。
2. 设定每个时间段内需要排的课程数以及每个课程的时间长度。
3. 设定每个教室的可用时间段。
4. 根据教师和课程的安排,确定每个时间段内需要排的课程。
5. 根据教室的可用时间段和每个时间段内需要排的课程,确定每个课程的具体时间和教室。
6. 输出最终的课程表。
以下是一个简单的 C++ 排课程序示例:
```cpp
#include <iostream>
#include <vector>
#include <map>
using namespace std;
// 定义课程信息结构体
struct Course {
string name;
int duration;
string teacher;
};
// 定义教室信息结构体
struct Classroom {
string name;
vector<int> available_timeslots;
};
// 定义时间段信息结构体
struct Timeslot {
string name;
int num_courses;
vector<Course> courses;
};
int main() {
// 设定时间段和教室数量
int num_timeslots = 5;
int num_classrooms = 3;
// 设定每个时间段的信息
vector<Timeslot> timeslots;
timeslots.push_back({"Monday morning", 3, {}});
timeslots.push_back({"Monday afternoon", 2, {}});
timeslots.push_back({"Tuesday morning", 2, {}});
timeslots.push_back({"Tuesday afternoon", 3, {}});
timeslots.push_back({"Wednesday morning", 2, {}});
// 设定每个教室的信息
vector<Classroom> classrooms;
classrooms.push_back({"Classroom A", {0, 1, 2, 3, 4}});
classrooms.push_back({"Classroom B", {0, 1, 2, 3, 4}});
classrooms.push_back({"Classroom C", {1, 2, 3, 4}});
// 设定每个课程的信息
map<string, Course> courses;
courses["Math"] = {"Math", 2, "Teacher A"};
courses["English"] = {"English", 3, "Teacher B"};
courses["History"] = {"History", 1, "Teacher C"};
courses["Science"] = {"Science", 2, "Teacher D"};
courses["Art"] = {"Art", 1, "Teacher E"};
// 根据教师和课程的安排,确定每个时间段内需要排的课程
for (auto& [name, course] : courses) {
for (auto& timeslot : timeslots) {
if (timeslot.courses.size() < timeslot.num_courses && course.teacher == "Teacher A" && timeslot.name.find("Monday") != string::npos) {
timeslot.courses.push_back(course);
break;
}
else if (timeslot.courses.size() < timeslot.num_courses && course.teacher == "Teacher B" && timeslot.name.find("Tuesday") != string::npos) {
timeslot.courses.push_back(course);
break;
}
else if (timeslot.courses.size() < timeslot.num_courses && course.teacher == "Teacher C" && timeslot.name.find("Wednesday") != string::npos) {
timeslot.courses.push_back(course);
break;
}
else if (timeslot.courses.size() < timeslot.num_courses && course.teacher == "Teacher D") {
timeslot.courses.push_back(course);
break;
}
else if (timeslot.courses.size() < timeslot.num_courses && course.teacher == "Teacher E") {
timeslot.courses.push_back(course);
break;
}
}
}
// 根据教室的可用时间段和每个时间段内需要排的课程,确定每个课程的具体时间和教室
for (auto& classroom : classrooms) {
for (int i = 0; i < classroom.available_timeslots.size(); i++) {
int timeslot_index = classroom.available_timeslots[i];
Timeslot& timeslot = timeslots[timeslot_index];
for (auto& course : timeslot.courses) {
if (course.duration <= timeslot.num_courses - timeslot.courses.size()) {
course.duration = timeslot.num_courses - timeslot.courses.size();
course.name = course.name + " (" + classroom.name + ")";
timeslot.courses[timeslot.courses.size() - 1] = course;
break;
}
}
}
}
// 输出最终的课程表
for (auto& timeslot : timeslots) {
cout << timeslot.name << ":" << endl;
for (auto& course : timeslot.courses) {
cout << " " << course.name << " (" << course.teacher << ")" << endl;
}
}
return 0;
}
```
这个程序实现了一个简单的排课,但是还有很多可以改进的地方,比如可以根据学生的选课情况和教师的可用时间自动排课,可以设计更加复杂的数据结构来优化算法等。
相关推荐
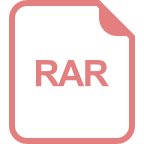
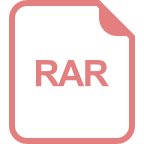
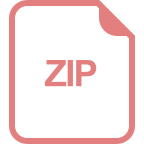
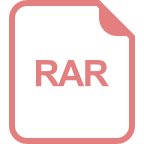
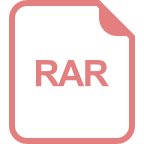
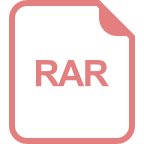
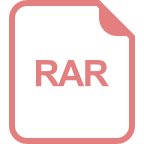


