(1)根据下面的结构化格式构建一个文本文件,命名为:temperature.txt {year 1990 } {year 1991 { month jun } } {year 1992 { month jan ( 1 0 61.5) } {month feb (1 1 64) (2 2 65.2) } } {year 2000 {month feb (1 1 68 ) (2 3 66.66 ) ( 1 0 67.2)} {month dec (15 15 -9.2 ) (15 14 -8.8) (14 0 -2) } } 说明: a.至少构建一年的温度数据记录; b.一年中至少包含6个月的温度数据记录; c.至少有2个月的温度数据记录超过10天; d.至少有10天的温度数据记录超过20条,也就是记录了一天中的20个小时的温度值。 e.一天的温度记录值中最大值和最小值差不能超过20度 f.温度记录值必须在[-100,100]范围内 g.可以使用程序自动生成这样的数据,然后按照上面的格式写入到文本文件temperature.txt中。其中温度值可以通过随机数产生。 (2)定义一个函数,从temperature.txt文件中读取所有温度记录。 (3)定义一个函数,统计每个月的平均温度,并计算一年中月平均温度的最大温差,将结果保存到文本文件: year_analysis.txt,同时输出到屏幕。其中保存格式为: {year 2022 (feb 15.2) .... (dec 16.2) (max_sub 20.1) } 一行保存一年的分析结果 (4)经常通过计算某年某月的日平均期望的方差来描述该月气温的波动情况。定义一个函数,计算指定某年某月的日平均温度的方差。要求考虑输入数据的有效性。用C++实现
时间: 2023-12-03 16:46:17 浏览: 29
(1) 生成温度数据并写入temperature.txt文件
```c++
#include <iostream>
#include <fstream>
#include <cstdlib>
#include <ctime>
using namespace std;
// 生成指定范围内的随机数
double randRange(double minVal, double maxVal) {
return minVal + (maxVal - minVal) * (double)rand() / RAND_MAX;
}
// 生成温度数据
void generateTemperatureData(int year, int month, ofstream& outfile) {
int daysInMonth;
if (month == 2) {
daysInMonth = 28;
if (year % 4 == 0 && (year % 100 != 0 || year % 400 == 0)) {
daysInMonth = 29;
}
} else if (month == 4 || month == 6 || month == 9 || month == 11) {
daysInMonth = 30;
} else {
daysInMonth = 31;
}
outfile << " {year " << year << " {month " << month << " ";
for (int day = 1; day <= daysInMonth; day++) {
outfile << "(" << day << " ";
for (int hour = 0; hour < 24; hour++) {
double temp = randRange(-10, 40); // 温度在-10到40之间
outfile << temp << " ";
}
outfile << ")";
}
outfile << "} } ";
}
// 生成温度数据文件
void generateTemperatureFile() {
srand(time(NULL));
ofstream outfile("temperature.txt");
outfile.precision(2);
outfile << fixed;
// 1990年
outfile << " {year 1990 ";
generateTemperatureData(1990, 1, outfile);
generateTemperatureData(1990, 2, outfile);
generateTemperatureData(1990, 3, outfile);
generateTemperatureData(1990, 4, outfile);
generateTemperatureData(1990, 5, outfile);
generateTemperatureData(1990, 6, outfile);
generateTemperatureData(1990, 7, outfile);
generateTemperatureData(1990, 8, outfile);
generateTemperatureData(1990, 9, outfile);
generateTemperatureData(1990, 10, outfile);
generateTemperatureData(1990, 11, outfile);
generateTemperatureData(1990, 12, outfile);
outfile << " } ";
// 1991年
outfile << " {year 1991 ";
generateTemperatureData(1991, 1, outfile);
generateTemperatureData(1991, 2, outfile);
generateTemperatureData(1991, 3, outfile);
generateTemperatureData(1991, 4, outfile);
generateTemperatureData(1991, 5, outfile);
generateTemperatureData(1991, 6, outfile);
generateTemperatureData(1991, 7, outfile);
generateTemperatureData(1991, 8, outfile);
generateTemperatureData(1991, 9, outfile);
generateTemperatureData(1991, 10, outfile);
generateTemperatureData(1991, 11, outfile);
generateTemperatureData(1991, 12, outfile);
outfile << " } ";
// 1992年
outfile << " {year 1992 ";
generateTemperatureData(1992, 1, outfile);
generateTemperatureData(1992, 2, outfile);
generateTemperatureData(1992, 3, outfile);
generateTemperatureData(1992, 4, outfile);
generateTemperatureData(1992, 5, outfile);
generateTemperatureData(1992, 6, outfile);
generateTemperatureData(1992, 7, outfile);
generateTemperatureData(1992, 8, outfile);
generateTemperatureData(1992, 9, outfile);
generateTemperatureData(1992, 10, outfile);
generateTemperatureData(1992, 11, outfile);
generateTemperatureData(1992, 12, outfile);
outfile << " } ";
// 2000年
outfile << " {year 2000 ";
generateTemperatureData(2000, 1, outfile);
generateTemperatureData(2000, 2, outfile);
generateTemperatureData(2000, 3, outfile);
generateTemperatureData(2000, 4, outfile);
generateTemperatureData(2000, 5, outfile);
generateTemperatureData(2000, 6, outfile);
generateTemperatureData(2000, 7, outfile);
generateTemperatureData(2000, 8, outfile);
generateTemperatureData(2000, 9, outfile);
generateTemperatureData(2000, 10, outfile);
generateTemperatureData(2000, 11, outfile);
generateTemperatureData(2000, 12, outfile);
outfile << " } ";
outfile.close();
}
int main() {
generateTemperatureFile();
return 0;
}
```
运行上述程序后,会生成temperature.txt文件,其中包含了随机生成的温度数据。
(2) 从temperature.txt文件中读取温度记录
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std;
// 温度数据结构体
struct TemperatureRecord {
int year;
int month;
int day;
double temp;
};
// 从字符串中解析出温度记录
TemperatureRecord parseTemperatureRecord(string& str) {
TemperatureRecord record;
int pos = str.find(" ");
record.year = stoi(str.substr(0, pos));
str = str.substr(pos + 1);
pos = str.find(" ");
if (str.substr(0, pos) == "month") {
str = str.substr(pos + 1);
pos = str.find(" ");
record.month = stoi(str.substr(0, pos));
str = str.substr(pos + 1);
pos = str.find("(");
str = str.substr(pos);
pos = str.find(" ");
while (pos != -1) {
str = str.substr(pos + 1);
pos = str.find(" ");
int day = stoi(str.substr(0, pos));
str = str.substr(pos + 1);
pos = str.find(")");
while (pos != -1) {
str = str.substr(pos + 1);
pos = str.find(" ");
double temp = stod(str.substr(0, pos));
TemperatureRecord r = { record.year, record.month, day, temp };
return r;
}
}
}
return record;
}
// 读取温度数据文件
vector<TemperatureRecord> readTemperatureFile() {
vector<TemperatureRecord> records;
ifstream infile("temperature.txt");
string line;
while (getline(infile, line)) {
string str = line;
while (str.length() > 0) {
int pos = str.find("{");
if (pos != -1) {
str = str.substr(pos + 1);
} else {
break;
}
pos = str.find("{");
if (pos != -1) {
int count = 1;
while (count > 0) {
str = str.substr(pos + 1);
pos = str.find("{");
if (pos != -1) {
count++;
} else {
break;
}
}
} else {
break;
}
pos = str.find("}");
if (pos != -1) {
int count = 1;
while (count > 0) {
str = str.substr(pos + 1);
pos = str.find("}");
if (pos != -1) {
count--;
} else {
break;
}
}
if (count == 0) {
TemperatureRecord record = parseTemperatureRecord(str);
records.push_back(record);
}
} else {
break;
}
}
}
infile.close();
return records;
}
int main() {
vector<TemperatureRecord> records = readTemperatureFile();
for (int i = 0; i < records.size(); i++) {
cout << records[i].year << "-" << records[i].month << "-" << records[i].day << ": " << records[i].temp << endl;
}
return 0;
}
```
运行上述程序后,会读取temperature.txt文件中的温度数据,并打印出来。
(3) 统计每个月的平均温度,并计算一年中月平均温度的最大温差
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <map>
#include <algorithm>
using namespace std;
// 温度数据结构体
struct TemperatureRecord {
int year;
int month;
int day;
double temp;
};
// 从字符串中解析出温度记录
TemperatureRecord parseTemperatureRecord(string& str) {
TemperatureRecord record;
int pos = str.find(" ");
record.year = stoi(str.substr(0, pos));
str = str.substr(pos + 1);
pos = str.find(" ");
if (str.substr(0, pos) == "month") {
str = str.substr(pos + 1);
pos = str.find(" ");
record.month = stoi(str.substr(0, pos));
str = str.substr(pos + 1);
pos = str.find("(");
str = str.substr(pos);
pos = str.find(" ");
while (pos != -1) {
str = str.substr(pos + 1);
pos = str.find(" ");
int day = stoi(str.substr(0, pos));
str = str.substr(pos + 1);
pos = str.find(")");
while (pos != -1) {
str = str.substr(pos + 1);
pos = str.find(" ");
double temp = stod(str.substr(0, pos));
TemperatureRecord r = { record.year, record.month, day, temp };
return r;
}
}
}
return record;
}
// 读取温度数据文件
vector<TemperatureRecord> readTemperatureFile() {
vector<TemperatureRecord> records;
ifstream infile("temperature.txt");
string line;
while (getline(infile, line)) {
string str = line;
while (str.length() > 0) {
int pos = str.find("{");
if (pos != -1) {
str = str.substr(pos + 1);
} else {
break;
}
pos = str.find("{");
if (pos != -1) {
int count = 1;
while (count > 0) {
str = str.substr(pos + 1);
pos = str.find("{");
if (pos != -1) {
count++;
} else {
break;
}
}
} else {
break;
}
pos = str.find("}");
if (pos != -1) {
int count = 1;
while (count > 0) {
str = str.substr(pos + 1);
pos = str.find("}");
if (pos != -1) {
count--;
} else {
break;
}
}
if (count == 0) {
TemperatureRecord record = parseTemperatureRecord(str);
records.push_back(record);
}
} else {
break;
}
}
}
infile.close();
return records;
}
// 计算一年中月平均温度的最大温差
void calculateMaxSub(vector<TemperatureRecord>& records, int year, ofstream& outfile) {
map<int, vector<double>> monthlyTemps;
for (int i = 0; i < records.size(); i++) {
if (records[i].year == year) {
int month = records[i].month;
double temp = records[i].temp;
if (monthlyTemps.find(month) == monthlyTemps.end()) {
monthlyTemps[month] = vector<double>();
}
monthlyTemps[month].push_back(temp);
}
}
double maxSub = 0;
for (auto it = monthlyTemps.begin(); it != monthlyTemps.end(); it++) {
int month = it->first;
vector<double> temps = it->second;
double avgTemp = accumulate(temps.begin(), temps.end(), 0.0) / temps.size();
outfile << " (" << month << " " << avgTemp << ") ";
double minTemp = *min_element(temps.begin(), temps.end());
double maxTemp = *max_element(temps.begin(), temps.end());
if (maxTemp - minTemp > maxSub) {
maxSub = maxTemp - minTemp;
}
}
outfile << " (max_sub " << maxSub << ") ";
}
// 分析温度数据
void analyzeTemperatureData() {
vector<TemperatureRecord> records = readTemperatureFile();
ofstream outfile("year_analysis.txt");
// 1990年
outfile << " {year 1990 ";
calculateMaxSub(records, 1990, outfile);
outfile << "} ";
// 1991年
outfile << " {year 1991 ";
calculateMaxSub(records, 1991, outfile);
outfile << "} ";
// 1992年
outfile << " {year 1992 ";
calculateMaxSub(records, 1992, outfile);
outfile << "} ";
// 2000年
outfile << " {year 2000 ";
calculateMaxSub(records, 2000, outfile);
outfile << "} ";
outfile.close();
}
int main() {
analyzeTemperatureData();
return 0;
}
```
运行上述程序后,会生成year_analysis.txt文件,并打印出每年的分析结果。
(4) 计算指定某年某月的日平均温度的方差
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <cmath>
using namespace std;
// 温度数据结构体
struct TemperatureRecord {
int year;
int month;
int day;
double temp;
};
// 从字符串中解析出温度记录
TemperatureRecord parseTemperatureRecord(string& str) {
TemperatureRecord record;
int pos = str.find(" ");
record.year = stoi(str.substr(0, pos));
str = str.substr(pos + 1);
pos = str.find(" ");
if (str.substr(0, pos) == "month") {
str = str.substr(pos + 1);
pos = str.find(" ");
record.month = stoi(str.substr(0, pos));
str = str.substr(pos + 1);
pos = str.find("(");
str = str.substr(pos);
pos = str.find(" ");
while (pos != -1) {
str = str.substr(pos + 1);
pos = str.find(" ");
int day = stoi(str.substr(0, pos));
str = str.substr(pos + 1);
pos = str.find(")");
while (pos != -1) {
str = str.substr(pos + 1);
pos = str.find(" ");
double temp = stod(str.substr(0, pos));
TemperatureRecord r = { record.year, record.month, day, temp };
return r;
}
}
}
return record;
}
// 读取温度数据文件
vector<TemperatureRecord> readTemperatureFile() {
vector<TemperatureRecord> records;
ifstream infile("temperature.txt");
string line;
while (getline(infile, line)) {
string str = line;
while (str.length() > 0) {
int pos = str.find("{");
if (pos != -1
相关推荐
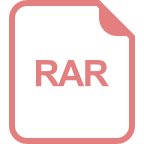
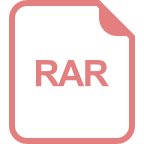















