在文件系统功能设计过程中,不能采用系统调用的方式进行文件的创建、删除、读、写等操作,应该自己设计程序实现。可以考虑在内存建立一个虚拟的磁盘空间,设计程序模拟实现文件空闲空间管理、文件目录管理、文件创建、删除、读、写等功能 用c++实现并输出
时间: 2024-01-31 14:02:11 浏览: 112
好的,那我来简单设计一下这个文件系统的实现:
1. 文件空闲空间管理
在内存中维护一个位图,表示每个磁盘块是否被占用。当创建一个新文件时,先遍历位图,找到一段连续的未被占用的块,标记为已占用,并记录在文件的元数据中。当删除一个文件时,将其占用的磁盘块标记为未占用。
2. 文件目录管理
在内存中维护一个类似于树形结构的目录结构,每个目录节点包含当前目录下的文件和子目录。可以使用链表或数组来表示子节点。当创建新文件或目录时,需要在相应的父目录下添加一个新的节点。
3. 文件创建、删除、读、写等功能
创建文件时,先检查文件名是否合法并且是否已经存在同名文件。如果合法,就在目录中添加一个新的文件节点,并分配一段连续的磁盘块作为文件的存储空间。
删除文件时,先检查文件是否存在。如果存在,就在目录中删除该文件节点,并释放其占用的磁盘块。
读文件时,先检查文件是否存在并且是否已经打开。如果存在并且已经打开,就根据文件的元数据信息读取相应的磁盘块,并将其存储在内存中。
写文件时,先检查文件是否存在并且是否已经打开。如果存在并且已经打开,就将数据写入缓存中,并在缓存满或者文件关闭时将缓存中的数据写回磁盘。
下面是一个简单的 C++ 实现,仅供参考:
```cpp
#include <iostream>
#include <vector>
#include <map>
#include <cstring>
using namespace std;
const int BLOCK_SIZE = 1024; // 磁盘块大小
const int BLOCK_NUM = 1000; // 磁盘块数量
const int MAX_FILE_NUM = 100; // 最大文件数量
const int MAX_FILE_NAME_LEN = 20; // 文件名最大长度
class Disk {
public:
Disk() {
blocks_.resize(BLOCK_NUM);
}
bool read(int block_num, char* buffer) {
if (block_num < 0 || block_num >= BLOCK_NUM) {
return false;
}
memcpy(buffer, &blocks_[block_num * BLOCK_SIZE], BLOCK_SIZE);
return true;
}
bool write(int block_num, char* buffer) {
if (block_num < 0 || block_num >= BLOCK_NUM) {
return false;
}
memcpy(&blocks_[block_num * BLOCK_SIZE], buffer, BLOCK_SIZE);
return true;
}
private:
vector<char> blocks_;
};
class File {
public:
File() : name_(""), size_(0), block_num_(0), block_offset_(0), is_open_(false) {}
bool create(const char* name, int size) {
if (strlen(name) > MAX_FILE_NAME_LEN) {
return false;
}
name_ = name;
size_ = size;
block_num_ = (size_ + BLOCK_SIZE - 1) / BLOCK_SIZE; // 向上取整
block_offset_ = 0;
is_open_ = false;
return true;
}
bool open() {
if (is_open_) {
return false;
}
is_open_ = true;
return true;
}
bool close() {
if (!is_open_) {
return false;
}
is_open_ = false;
return true;
}
const char* get_name() const {
return name_.c_str();
}
int get_size() const {
return size_;
}
int get_block_num() const {
return block_num_;
}
int read_block(int index, char* buffer) const {
if (index < 0 || index >= block_num_) {
return -1;
}
int block_num = blocks_[index];
if (block_num < 0) {
return -1;
}
if (!disk_.read(block_num, buffer)) {
return -1;
}
return block_num;
}
int write_block(int index, char* buffer) {
if (index < 0 || index >= block_num_) {
return -1;
}
int block_num = blocks_[index];
if (block_num < 0) {
block_num = find_free_block();
if (block_num < 0) {
return -1;
}
blocks_[index] = block_num;
}
if (!disk_.write(block_num, buffer)) {
return -1;
}
return block_num;
}
private:
int find_free_block() {
for (int i = 0; i < BLOCK_NUM; ++i) {
if (!block_bitmap_[i]) {
block_bitmap_[i] = true;
return i;
}
}
return -1;
}
string name_; // 文件名
int size_; // 文件大小
int block_num_; // 文件占用的磁盘块数量
int block_offset_; // 当前读写位置相对于文件起始位置的偏移量
bool is_open_; // 是否已经打开
Disk disk_; // 磁盘
vector<int> blocks_; // 文件占用的磁盘块编号列表
static bool block_bitmap_[BLOCK_NUM]; // 磁盘块占用情况位图
};
bool File::block_bitmap_[BLOCK_NUM] = {false};
class Directory {
public:
Directory() {
files_.resize(MAX_FILE_NUM);
}
bool create_file(const char* name, int size) {
if (get_file(name) != nullptr) {
return false;
}
for (int i = 0; i < MAX_FILE_NUM; ++i) {
if (files_[i] == nullptr) {
files_[i] = new File();
if (files_[i]->create(name, size)) {
return true;
} else {
delete files_[i];
files_[i] = nullptr;
return false;
}
}
}
return false;
}
bool delete_file(const char* name) {
File* file = get_file(name);
if (file == nullptr) {
return false;
}
for (int i = 0; i < MAX_FILE_NUM; ++i) {
if (files_[i] == file) {
delete files_[i];
files_[i] = nullptr;
return true;
}
}
return false;
}
File* get_file(const char* name) const {
for (int i = 0; i < MAX_FILE_NUM; ++i) {
if (files_[i] != nullptr && strcmp(files_[i]->get_name(), name) == 0) {
return files_[i];
}
}
return nullptr;
}
private:
vector<File*> files_;
};
int main() {
Directory dir;
dir.create_file("test.txt", 1024);
File* file = dir.get_file("test.txt");
if (file != nullptr) {
file->open();
char buffer[BLOCK_SIZE];
memset(buffer, 0, BLOCK_SIZE);
for (int i = 0; i < file->get_block_num(); ++i) {
file->write_block(i, buffer);
}
file->close();
}
dir.delete_file("test.txt");
return 0;
}
```
阅读全文
相关推荐
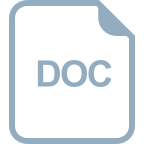
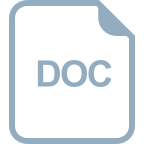
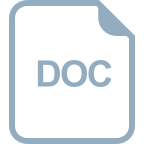
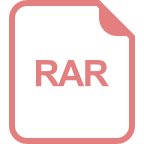
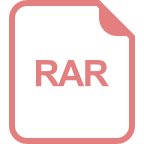
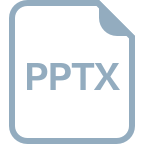
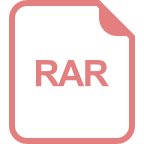
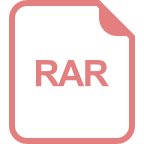
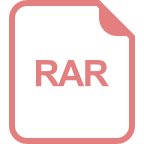
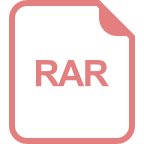
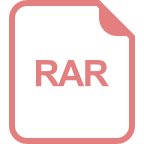
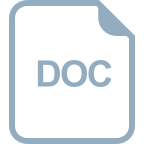
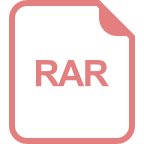
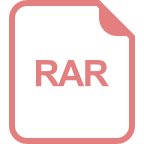
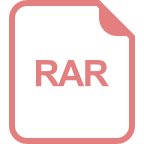
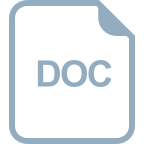
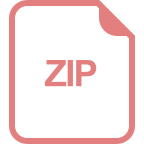
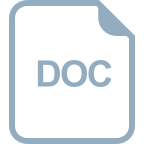
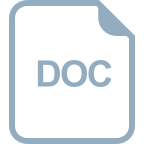