五子棋人机对战实现简单代码
时间: 2023-07-29 22:09:56 浏览: 58
下面是一个简单的五子棋人机对战的代码示例:
```python
import random
# 初始化棋盘
def init_board():
board = [[' ' for _ in range(15)] for _ in range(15)]
return board
# 打印棋盘
def print_board(board):
print(' 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14')
print(' ------------------------------')
for i in range(15):
print('{}|'.format(i), end='')
for j in range(15):
print('{}|'.format(board[i][j]), end='')
print('\n ------------------------------')
# 判断是否下满了棋子
def is_full(board):
for i in range(15):
for j in range(15):
if board[i][j] == ' ':
return False
return True
# 判断是否胜利
def is_win(board, row, col, player):
# 水平方向
count = 0
for i in range(col-4, col+5):
if i < 0 or i >= 15:
continue
if board[row][i] == player:
count += 1
if count == 5:
return True
else:
count = 0
# 垂直方向
count = 0
for i in range(row-4, row+5):
if i < 0 or i >= 15:
continue
if board[i][col] == player:
count += 1
if count == 5:
return True
else:
count = 0
# 左上到右下方向
count = 0
for i in range(-4, 5):
r = row + i
c = col + i
if r < 0 or r >= 15 or c < 0 or c >= 15:
continue
if board[r][c] == player:
count += 1
if count == 5:
return True
else:
count = 0
# 右上到左下方向
count = 0
for i in range(-4, 5):
r = row + i
c = col - i
if r < 0 or r >= 15 or c < 0 or c >= 15:
continue
if board[r][c] == player:
count += 1
if count == 5:
return True
else:
count = 0
return False
# 玩家下棋
def player_move(board):
while True:
row = int(input('请输入行号(0-14): '))
col = int(input('请输入列号(0-14): '))
if row >= 0 and row < 15 and col >= 0 and col < 15 and board[row][col] == ' ':
board[row][col] = 'X'
break
else:
print('输入无效,请重新输入!')
# AI下棋
def ai_move(board):
while True:
row = random.randint(0, 14)
col = random.randint(0, 14)
if board[row][col] == ' ':
board[row][col] = 'O'
break
# 主函数
def main():
board = init_board()
print_board(board)
while True:
player_move(board)
print_board(board)
if is_win(board, row, col, 'X'):
print('恭喜你,你赢了!')
break
if is_full(board):
print('平局!')
break
ai_move(board)
print_board(board)
if is_win(board, row, col, 'O'):
print('很遗憾,你输了!')
break
if is_full(board):
print('平局!')
break
if __name__ == '__main__':
main()
```
这个代码实现了简单的五子棋人机对战,玩家执黑棋,AI执白棋。玩家通过输入行号和列号来下棋,AI则随机选择一个空位置下棋。游戏在玩家或AI获胜、棋盘下满时结束。
相关推荐
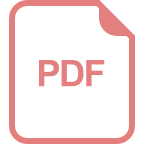
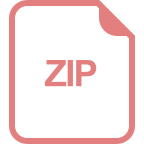














